So the other day I was trying to integrate Remita payment integration system into a React software application I was working on.
Like all developers, I browsed through their website looking for an official documentation to use, just like the other payment systems, but couldn’t find any.
Granted, there’s a documentation for Javascript, Node js, PHP and other software development programs listed on the Remita website. So what I’m going to do is convert the plain Javascript code to React.
At the time of writing this article, there still isn’t an official documentation on how to generate RRR in React JS on the Remita website, and I hope this simplifies things well enough for other developers.
See Also: How to Use Data tables in React JS
Let’s begin.
Make Payment With Remita
Since we’re using Crypto JS for the API hash, we need to first import that into our project using yarn.
yarn add crypto-js
Next, we initialise it in our project.
import React, { Component } from 'react';
let CryptoJS = require("crypto-js");
makeRemitaPayment = () => {
let merchantId = "2547916";
let apiKey = "1946"
let serviceTypeId = "4430731"
let d = new Date();
let orderId = d.getTime();
let totalAmount = "10000";
let apiHash = CryptoJS.SHA512(merchantId+serviceTypeId+orderId+totalAmount+apiKey);
let req = {
method: "POST",
headers: {
Accept: "application/json",
"Content-Type": "application/json",
Authorization: `remitaConsumerKey=${merchantId},remitaConsumerToken=${apiHash}`,
},
body: JSON.stringify({
"serviceTypeId": serviceTypeId,
"amount": totalAmount,
"orderId": orderId,
"payerName": 'Pete Attah',
"payerEmail": '[email protected]',
"payerPhone": '808080808080',
"description": "Your payment description here"
}),
};
fetch(`https://remitademo.net/remita/exapp/api/v1/send/api/echannelsvc/merchant/api/paymentinit`, req)
.then((response) => response.json())
.then((responseJson) => {
console.warn(responseJson);
})
.catch((error) => {
console.log(error);
});
}
Result:

Check Transaction Status
To check transaction status, we will pass the merchant id, the generated rrr and the api hash.
checkPaymentStatus = async () => {
let merchantId = "2547916";
let apiKey = "1946"
let serviceTypeId = "4430731"
let d = new Date();
let orderId = d.getTime();
let totalAmount = "10000";
let rrr = "your_generated_rrr";
let apiHash = CryptoJS.SHA512(rrr + apiKey + merchantId);
let req = {
method: "GET",
headers: {
Accept: "application/json",
"Content-Type": "application/json",
Authorization: `remitaConsumerKey=${merchantId},remitaConsumerToken=${apiHash}`,
},
};
await fetch(`https://remitademo.net/remita/exapp/api/v1/send/api/echannelsvc/${merchantId}/${rrr}/${apiHash}/status.reg`, req)
.then((response) => response.json())
.then((responseJson) => {
console.warn(responseJson);
})
.catch((error) => {
console.error(error);
});
});
}
Result For Remita Payment Integration:
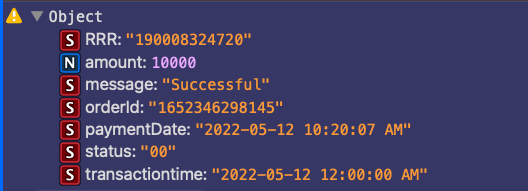
Conclusion
There we have it guys. We’ve been able to both generate RRR and check transaction status.
Let me know if you have any questions in the comments.
Enrol For A Software Development Training
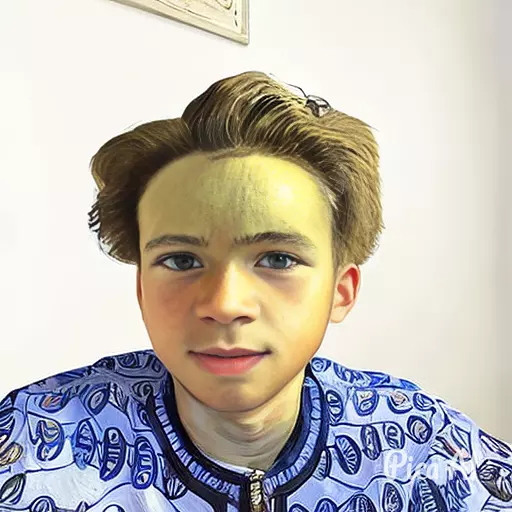
Hi…Please does this method show the payment widget for inputing card details?
No, this tutorial does not support that because the demonstration is on Remita’s documentation. This tutorial captures what Remita did not originally have in their documentation.