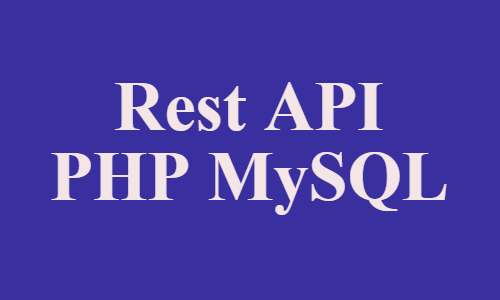
API stands for Application Programming Interface.
It’s an intermediary that allows two applications to talk to each other by both delivering requests to the provider and getting back a response.
REST stands for Representational State Transfer
See How to work with Fetch API
In this tutorial, we are going to demonstrate how to create a REST API in our software application using PHP.
We are going to create an API with a GET Request. We want to retrieve registration details from our database.
1. Setup the Database
First, we need to setup our table. We will create our table as follows
CREATE TABLE `your_database_name`.`test_registration` ( `id` INT NOT NULL AUTO_INCREMENT , `username` VARCHAR(255) NOT NULL , `email` VARCHAR(255) NOT NULL , `password` TEXT NOT NULL , `date_time` DATETIME NOT NULL DEFAULT CURRENT_TIMESTAMP , PRIMARY KEY (`id`)) ENGINE = MyISAM;
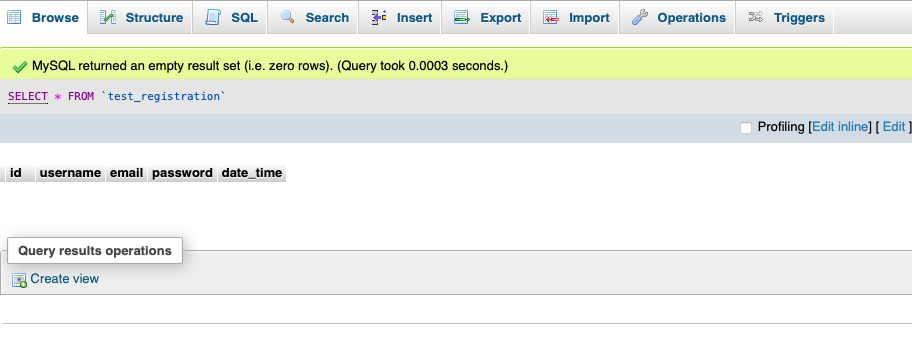
2. Add Mock Data
Next, we are going add sample data to our table so that we can have something to retrieve.
INSERT INTO `test_registration` (`id`, `username`, `email`, `password`, `date_time`) VALUES (NULL, 'Luke', '[email protected]', '123333', current_timestamp());
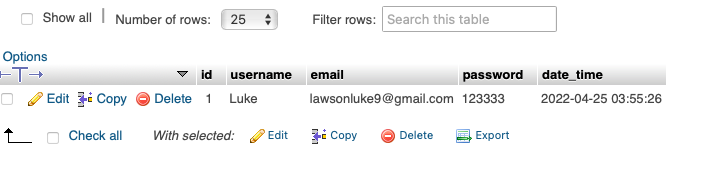
3. Create the API
Next, we are going to write the API that will fetch our records from the database.
What we want to do first is to write a database connection script and we’re using PDO. We’ve covered much about PDO here.
We’re fetching the result and we’re storing it in an array and encoding with JSON.
<?php
header("Access-Control-Allow-Origin: *");
header("Access-Control-Allow-Headers: access");
header("Access-Control-Allow-Methods: GET");
header("Content-Type: application/json; charset=UTF-8");
class Constants
{
static $DB_SERVER="localhost";
static $DB_NAME="your_database_name";
static $USERNAME="your_database_username";
static $PASSWORD="your_database_password";
static $SQL_SELECT_ALL="SELECT * FROM test_registration ORDER BY RAND()";
}
class Register
{
public function connect()
{
$con=new mysqli(Constants::$DB_SERVER,Constants::$USERNAME,Constants::$PASSWORD,Constants::$DB_NAME);
if($con->connect_error)
{
return null;
}else
{
return $con;
}
}
public function select()
{
$con=$this->connect();
if($con != null)
{
$result=$con->query(Constants::$SQL_SELECT_ALL);
if($result->num_rows>0)
{
$reg=array();
while($row=$result->fetch_array())
{
array_push($reg, array("id"=>$row['id'],"email"=>$row['email'],"username"=>$row['username']));
}
print(json_encode(array_reverse($reg)));
}else
{
print(json_encode(array("No records found")));
}
$con->close();
}else{
print(json_encode(array("PHP EXCEPTION : CAN'T CONNECT TO MYSQL. NULL CONNECTION.")));
}
}
}
$reg=new Register();
$reg->select();
?>
Result:

Conclusion
This is how we create API using vanilla PHP.
I have read your blog on create RESTful APIs with PHP. It was very interesting and helpful but I can add some extra points in your article. Here some extra points:
1.Create a database and DB table.
2.Establish database connection.
3.Create a REST API file. Create index or HTML file. Fetch the records from database via cURL.
These are some extra points to add to your article. Readers if you are confused about your web and App development , you can get a free consultation at Alakmalak technologies.Visit our site for more information.