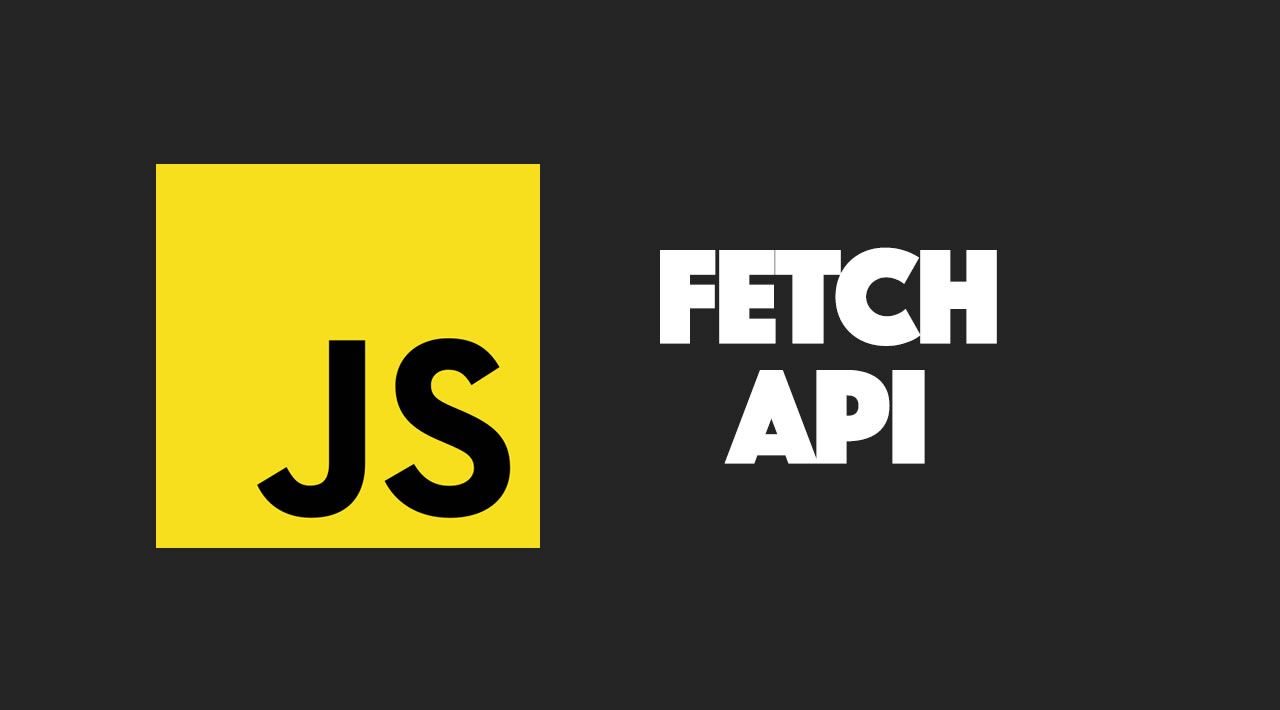
If you are writing a web application, there is every possibility that at some point you will have to work with external data.
This can be data from your own database, third party REST APIs, etc. In that case, you will require some way to get that data or, as the case may be, post, delete or update that data. The fetch API in Javascript lets you do just that.
When AJAX (Asynchronous Javascript And XML) first appeared in 1999, it gave us a better option in terms of web development. AJAX was a milestone in web development and is the brain behind many modern technologies like React, React Native, etc.
Before AJAX, you will have to re-render an entire webpage even for minor updates. But AJAX gave us a sleek way of fetching content from the backend and updating selected interface(s) without having to re-render the webpage. This has helped to improve user experience and build larger and complicated web platforms.
But Are REST APIs?
REST stands for Representational State Transfer.
A REST API allows you to push and pull (or retrieve) data from a database without you needing the database credentials.
This means that we could pull data from a third party API whose developers does not necessarily have any interaction or connection us.
Types of REST APIs
GET – Retrieve data from the API
POST – Push data to the API. E.g., create a new record.
PUT – Update existing record with new data
DELETE – Remove a record
Elements of a REST API
- REQUEST: This is usually the data that you send to the API.
- RESPONSE: This is the data you get back from the server after a successful or failed request.
- HEADERS: This is generally additional metadata passed to the API to assist the server in understanding what type of request you are sending.
USING THE FETCH API
The Fetch API is a watered-down version of the XMLHttpRequest, and it is used to consume data asynchronously.
The major difference between these methods of request is that Fetch works with promises, while XMLHttpRequest works with callbacks.
//METHOD OF USE
fetch('url')
.then(response => response.json()) //convert to json
.then(json => console.log(json)) //print data
.catch(err => console.log('Request failed', err));
Now, let’s do an example of GET Request that fetches data from an API and outputs the result to html.
We will be making use of a dummy API that fetches Github commits.
- First create your html page and add a div that shows the result
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="result"></div>
</body>
2. Add your Javascript logic
<script>
fetch('https://api.github.com/repos/javascript-tutorial/en.javascript.info/commits')
.then(response => response.json())
.then(response => response.map(data => {
//Let us display the data and see the result
console.log(data)
}
).catch(err => console.log(`error is: ${err}`));
</script>
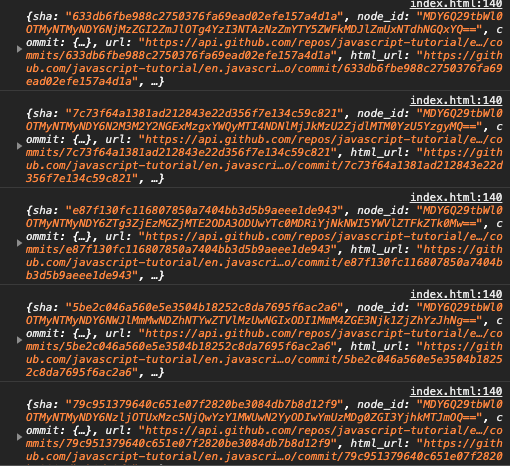
Notice that we are receiving our data objects in the browser console.
Now let us complete the code.
<script>
fetch('https://api.github.com/repos/javascript-tutorial/en.javascript.info/commits')
.then(response => response.json())
.then(response => response.map(data => {
//Let us display the data and see the result
//console.log(data)
for (const [key, value] of Object.entries(data.author)) {
let resultDiv = document.querySelector("#result")
let element = document.createElement('p');
element.innerHTML = `${key}: ${value}`
resultDiv.append(element);
}
}
).catch(err => console.log(`error is: ${err}`));
</script>
Complete code Here
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="result"></div>
<script>
fetch('https://api.github.com/repos/javascript-tutorial/en.javascript.info/commits')
.then(response => response.json())
.then(response => response.map(data => {
//Let us display the data and see the result
//console.log(data)
for (const [key, value] of Object.entries(data.author)) {
let resultDiv = document.querySelector("#result")
let element = document.createElement('p');
element.innerHTML = `${key}: ${value}`
resultDiv.append(element);
}
}
).catch(err => console.log(`error is: ${err}`));
</script>
</body>
1 thought on “Working With Fetch API”