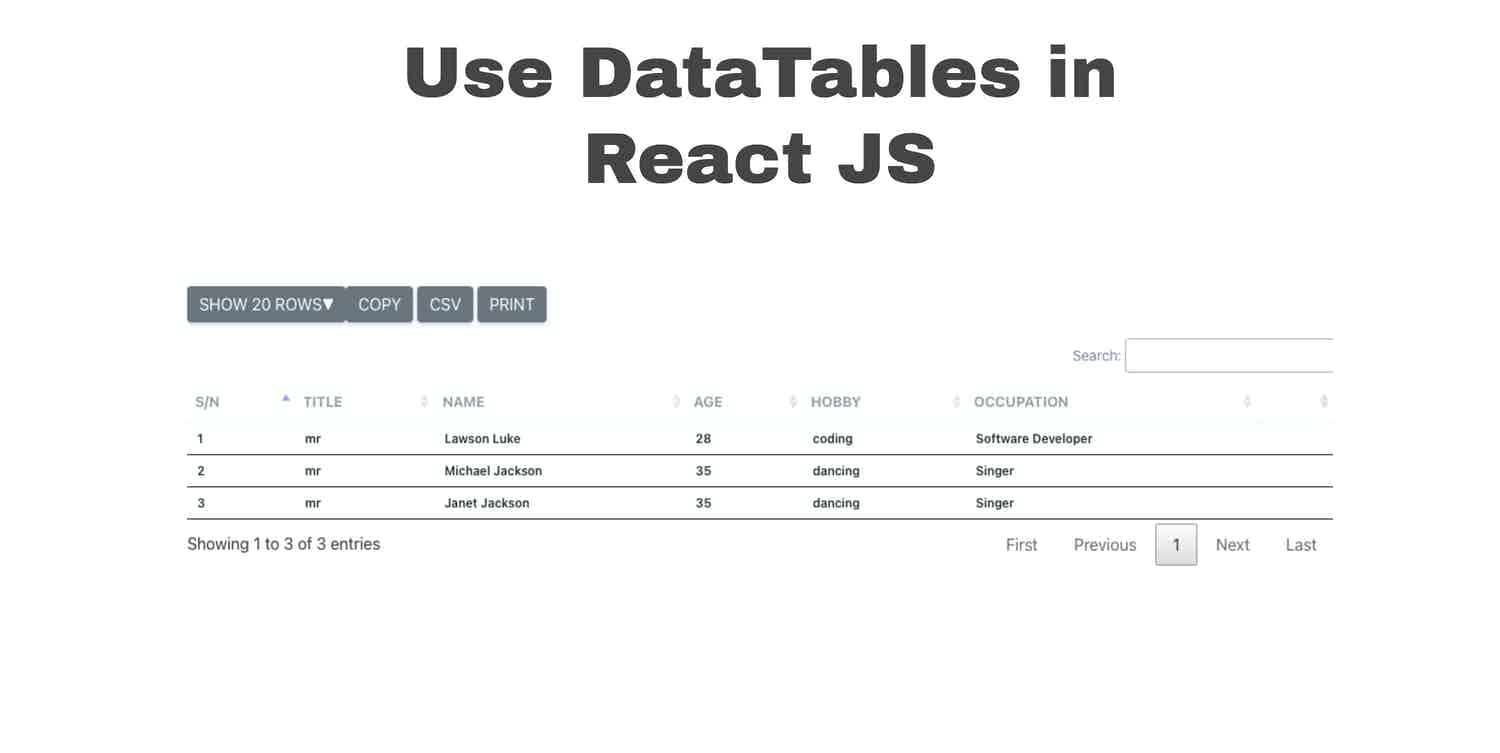
Knowing how to use dataTables in React JS can be very rewarding and time-saving.
DataTables is a powerful Javascript library for adding advanced interaction features to HTML tables.
This is generally useful especially when you need to not just merely display data from database in your software application, but to also do more like pagination, sorting, searching, etc. DataTables comes bundled with all those functionalities.
And while this explanation seems plausible enough, it can appear quite daunting to get started. However, taking those first steps and getting DataTables running on your web-site is actually quite straight forward as you need only include, maybe, just two files in your page. But with React JS, you’ll have to do more than that.
In this tutorial, we shall exploring dataTables with all of its in-built functionalities like sorting, copying records, printing records and downloading records as CSV file.
Let’s just get started.
First, we’ll need to add the following dependencies to our project:
yarn add jquery
yarn add datatable
yarn add datatables.net
yarn add datatables.net-buttons
yarn add datatables.net-dt
yarn add bootstrap
We’re adding jquery because that’s a necessary dependency for dataTable to work. Next, we’re the dataTable dependency itself and the one for sorting and also bootstrap for styling.
I’m already assuming you know the basics of creating and setting up a new React project, if not please go through the tutorial here.
Next, we’ll import these dependencies to our project
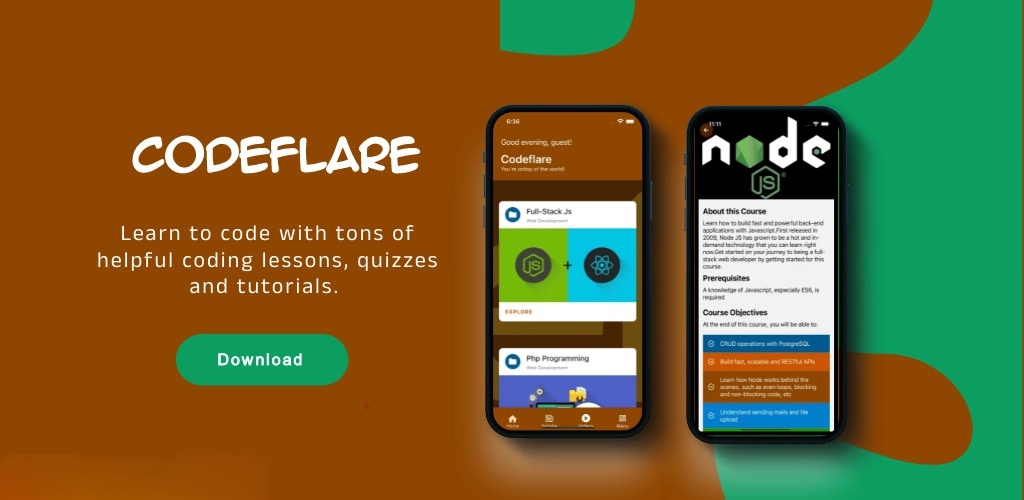
import "jquery/dist/jquery.min.js";
import "datatables.net-dt/js/dataTables.dataTables";
import "datatables.net-dt/css/jquery.dataTables.min.css";
import "datatables.net-buttons/js/dataTables.buttons.js";
import "datatables.net-buttons/js/buttons.colVis.js";
import "datatables.net-buttons/js/buttons.flash.js";
import "datatables.net-buttons/js/buttons.html5.js";
import "datatables.net-buttons/js/buttons.print.js";
import $ from "jquery";
class App extends Component {
render(){
return(
<div>
</div>
)
}
}
export default App;
We’re just going to have an array of objects and map the values to our table. But first, let’s define our array of objects, something simple.
const names = [
{
"title" : "mr",
"firstname" : "Lawson",
"lastname" : "Luke",
"age" : 28,
"occupation" : "Software Developer",
"hobby" : "coding"
},
{
"title" : "mr",
"firstname" : "Michael",
"lastname" : "Jackson",
"age" : 35,
"occupation" : "Singer",
"hobby" : "dancing"
},
{
"title" : "mr",
"firstname" : "Janet",
"lastname" : "Jackson",
"age" : 35,
"occupation" : "Singer",
"hobby" : "dancing"
}
]
To specify the rows to be viewed, show both print button, copy data records and download data as a CSV, we need to add the following code immediately our page loads.
Learn about component life cycles here
componentDidMount(){
if (!$.fn.DataTable.isDataTable("#myTable")) {
$(document).ready(function () {
setTimeout(function () {
$("#table").DataTable({
pagingType: "full_numbers",
pageLength: 20,
processing: true,
dom: "Bfrtip",
select: {
style: "single",
},
buttons: [
{
extend: "pageLength",
className: "btn btn-secondary bg-secondary",
},
{
extend: "copy",
className: "btn btn-secondary bg-secondary",
},
{
extend: "csv",
className: "btn btn-secondary bg-secondary",
},
{
extend: "print",
customize: function (win) {
$(win.document.body).css("font-size", "10pt");
$(win.document.body)
.find("table")
.addClass("compact")
.css("font-size", "inherit");
},
className: "btn btn-secondary bg-secondary",
},
],
fnRowCallback: function (
nRow,
aData,
iDisplayIndex,
iDisplayIndexFull
) {
var index = iDisplayIndexFull + 1;
$("td:first", nRow).html(index);
return nRow;
},
lengthMenu: [
[10, 20, 30, 50, -1],
[10, 20, 30, 50, "All"],
],
columnDefs: [
{
targets: 0,
render: function (data, type, row, meta) {
return type === "export" ? meta.row + 1 : data;
},
},
],
});
}, 1000);
});
}
}
Moving on, let’s define a function that will map our array values to our table.
showTable = () => {
try {
return names.map((item, index) => {
return (
<tr>
<td className="text-xs font-weight-bold">{index +1}</td>
<td className="text-xs font-weight-bold">{item.title}</td>
<td className="text-xs font-weight-bold">{item.firstname+ ' ' + item.lastname}</td>
<td className="text-xs font-weight-bold">{item.age}</td>
<td className="text-xs font-weight-bold">{item.hobby}</td>
<td className="text-xs font-weight-bold">{item.occupation}</td>
<td></td>
</tr>
);
});
} catch (e) {
alert(e.message);
}
};
Finally, in our render method, we will define our table headings and call our showTable() function.
<div class="container-fluid py-4">
<div class="table-responsive p-0 pb-2">
<table id="table" className="table align-items-center justify-content-center mb-0">
<thead>
<tr>
<th className="text-uppercase text-secondary text-sm font-weight-bolder opacity-7 ps-2">S/N</th>
<th className="text-uppercase text-secondary text-sm font-weight-bolder opacity-7 ps-2">Title</th>
<th className="text-uppercase text-secondary text-sm font-weight-bolder opacity-7 ps-2">Name</th>
<th className="text-uppercase text-secondary text-sm font-weight-bolder opacity-7 ps-2">Age</th>
<th className="text-uppercase text-secondary text-sm font-weight-bolder opacity-7 ps-2">Hobby</th>
<th className="text-uppercase text-secondary text-sm font-weight-bolder opacity-7 ps-2">Occupation</th>
<th></th>
</tr>
</thead>
<tbody>
{this.showTable()}
</tbody>
</table>
</div>
</div>
Now, putting all our code together …
import "jquery/dist/jquery.min.js";
import "datatables.net-dt/js/dataTables.dataTables";
import "datatables.net-dt/css/jquery.dataTables.min.css";
import "datatables.net-buttons/js/dataTables.buttons.js";
import "datatables.net-buttons/js/buttons.colVis.js";
import "datatables.net-buttons/js/buttons.flash.js";
import "datatables.net-buttons/js/buttons.html5.js";
import "datatables.net-buttons/js/buttons.print.js";
import $ from "jquery";
const names = [
{
"title" : "mr",
"firstname" : "Lawson",
"lastname" : "Luke",
"age" : 28,
"occupation" : "Software Developer",
"hobby" : "coding"
},
{
"title" : "mr",
"firstname" : "Michael",
"lastname" : "Jackson",
"age" : 35,
"occupation" : "Singer",
"hobby" : "dancing"
},
{
"title" : "mr",
"firstname" : "Janet",
"lastname" : "Jackson",
"age" : 35,
"occupation" : "Singer",
"hobby" : "dancing"
}
]
class App extends Component {
componentDidMount(){
if (!$.fn.DataTable.isDataTable("#myTable")) {
$(document).ready(function () {
setTimeout(function () {
$("#table").DataTable({
pagingType: "full_numbers",
pageLength: 20,
processing: true,
dom: "Bfrtip",
select: {
style: "single",
},
buttons: [
{
extend: "pageLength",
className: "btn btn-secondary bg-secondary",
},
{
extend: "copy",
className: "btn btn-secondary bg-secondary",
},
{
extend: "csv",
className: "btn btn-secondary bg-secondary",
},
{
extend: "print",
customize: function (win) {
$(win.document.body).css("font-size", "10pt");
$(win.document.body)
.find("table")
.addClass("compact")
.css("font-size", "inherit");
},
className: "btn btn-secondary bg-secondary",
},
],
fnRowCallback: function (
nRow,
aData,
iDisplayIndex,
iDisplayIndexFull
) {
var index = iDisplayIndexFull + 1;
$("td:first", nRow).html(index);
return nRow;
},
lengthMenu: [
[10, 20, 30, 50, -1],
[10, 20, 30, 50, "All"],
],
columnDefs: [
{
targets: 0,
render: function (data, type, row, meta) {
return type === "export" ? meta.row + 1 : data;
},
},
],
});
}, 1000);
});
}
}
showTable = () => {
try {
return names.map((item, index) => {
return (
<tr>
<td className="text-xs font-weight-bold">{index +1}</td>
<td className="text-xs font-weight-bold">{item.title}</td>
<td className="text-xs font-weight-bold">{item.firstname+ ' ' + item.lastname}</td>
<td className="text-xs font-weight-bold">{item.age}</td>
<td className="text-xs font-weight-bold">{item.hobby}</td>
<td className="text-xs font-weight-bold">{item.occupation}</td>
<td></td>
</tr>
);
});
} catch (e) {
alert(e.message);
}
};
render(){
return(
<div class="container-fluid py-4">
<div class="table-responsive p-0 pb-2">
<table id="table" className="table align-items-center justify-content-center mb-0">
<thead>
<tr>
<th className="text-uppercase text-secondary text-sm font-weight-bolder opacity-7 ps-2">S/N</th>
<th className="text-uppercase text-secondary text-sm font-weight-bolder opacity-7 ps-2">Title</th>
<th className="text-uppercase text-secondary text-sm font-weight-bolder opacity-7 ps-2">Name</th>
<th className="text-uppercase text-secondary text-sm font-weight-bolder opacity-7 ps-2">Age</th>
<th className="text-uppercase text-secondary text-sm font-weight-bolder opacity-7 ps-2">Hobby</th>
<th className="text-uppercase text-secondary text-sm font-weight-bolder opacity-7 ps-2">Occupation</th>
<th></th>
</tr>
</thead>
<tbody>
{this.showTable()}
</tbody>
</table>
</div>
</div>
)
}
}
export default App;
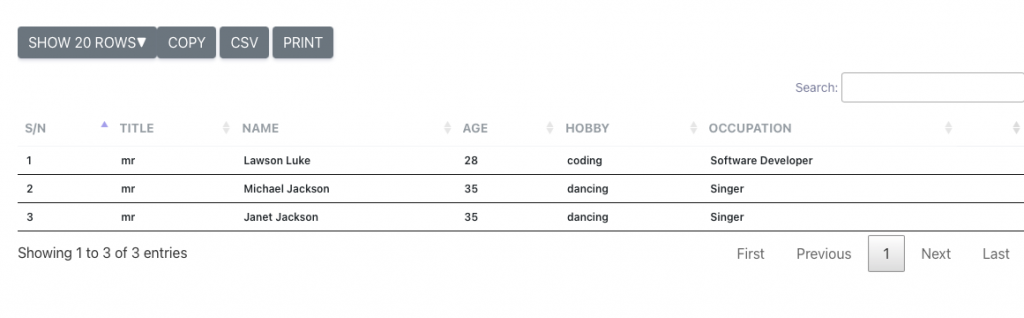
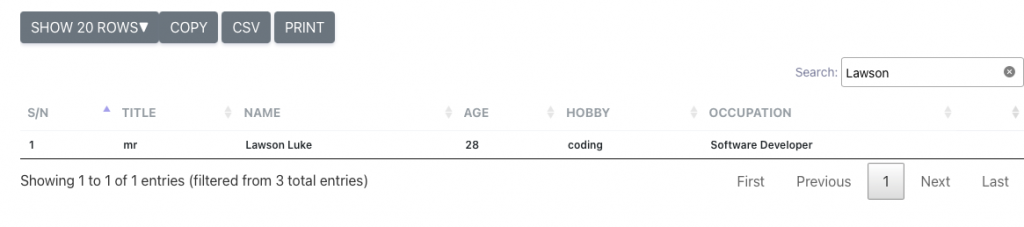
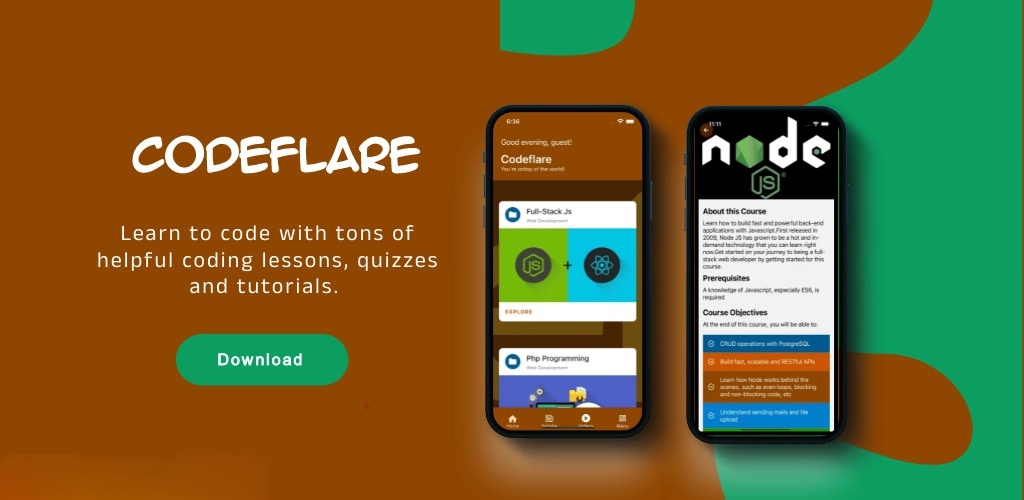
Conclusion
With this we have demonstrated how to use DataTables in React JS.
hi, I want to use Search panes to for this I install it from Datatables site. But When I import under your imports it returns “SearchPane requires Select at new SearchPanes ” error. What am I doing wrong ?
Could you please explain how to do this by using functional components