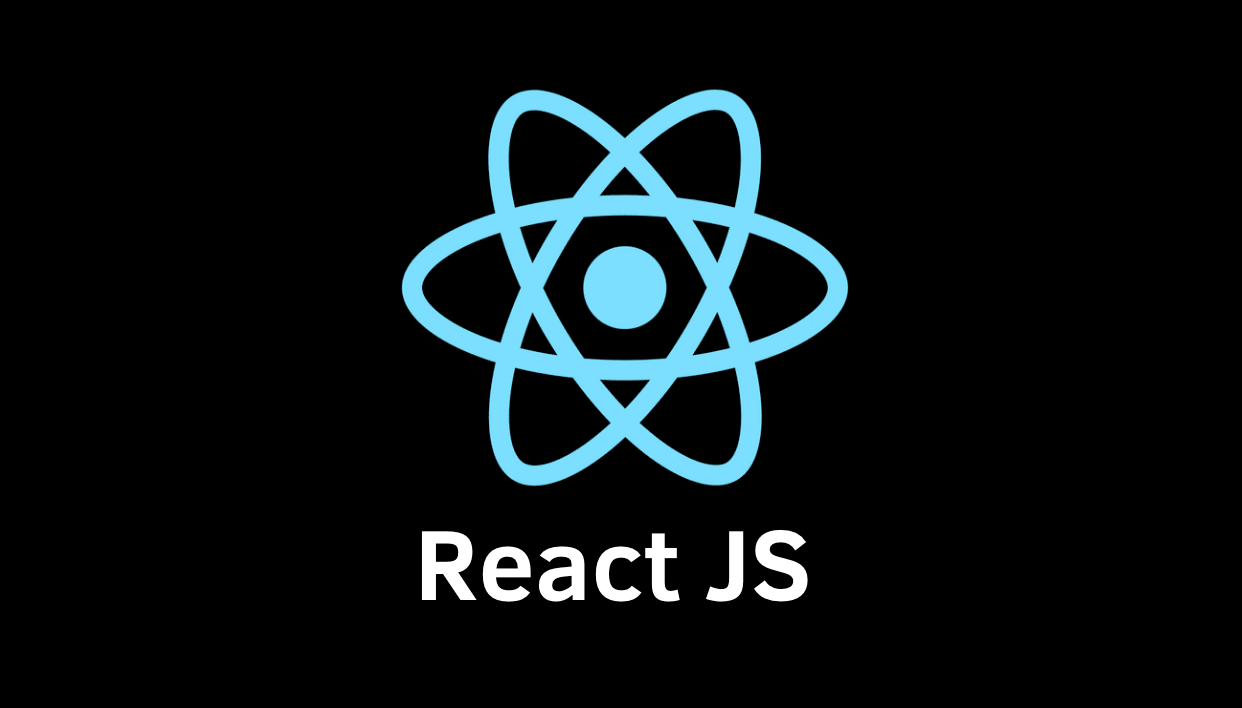
The componentDidMount() lifecycle method is called immediately after a component is mounted or, in simple terms, when that particular component or page is in use or in focus or in the DOM.
You can then decide what should happen when that page loads. If you’re fetching data from a remote endpoint or your application’s functionality relies heavily on this type of data, componentDidMount() is a good place to instantiate your network request.
Mounting a Component
When mounting a component, there are about four (4) in-built methods that get called in a particular order. These are:
- constructor()
- getDerivedStateFromProps()
- render()
- componentDidMount()
constructor()
The constructor method is the first that’s called before anything else in your React Component, and this is where initial states are usually set up for use by the application.
The constructor method is called with props passed in as a parameter and is always immediately followed by super before anything else is passed. This is done so that the parent’s constructor method can be initiated and will also allow the component to inherit methods from its parents.
Here’s an illustration
class YourClassName extends Component{
constructor(props){
super(props){
this.state = {
name: 'Lawson Luke'
}
}
}
render(){
return(
<div>
<p>My name is {this.state.name} </p>
</div>
)
}
}
export default YourClassName;
getDerivedStateFromProps()
The getDerivedStateFromProps()
method is called just before rendering the element(s) in the DOM.
This is usually where you update or set your state based on the initial value of the props.
static getDerivedStateFromProps(props, state) {
return {name: props.newName };
}
render()
The render() method is only required in a class component. When called, it examines the values you’ve passed into this.props, this.state and just outputs your HTML result or the results of your code.
componentDidMount()
This is called after the component is rendered or is available in the DOM. You may want to perform some logic here like, for example, set state value.
class YourClassName extends React.Component {
constructor(props) {
super(props);
this.state = {name: "Lawson Luke"};
}
componentDidMount() {
setTimeout(() => {
this.setState({name: "Matt Hendrik"})
}, 1000)
}
render() {
return (
<h1>My name is {this.state.name}</h1>
);
}
export default YourClassName