What is a POST Request?
The POST request is part of the Http methods which is used as a request protocol between a client and a server.
A POST method typically sends data to the server. The type of the body of the request is indicated by the Content-Type
header.
In creating mobile applications in React Native, there are times where you’ll need to submit a form or other resource to the server. This tutorial shows you how to do that using the Fetch API
Working With Fetch API
The Fetch API provides some type of interface for fetching resources from a network.
The fetch()
method takes one mandatory argument, the path to the resource you want to fetch. It returns a Promise
that resolves to the Response
to that request, whether it is successful or not. You can also optionally pass in an init
options object as the second argument.
Let’s see an example …
fetch('https://api.github.com/users/defunkt', {
method: 'POST',
headers: {
Accept: 'application/json',
'Content-Type': 'application/json',
},
body: JSON.stringify({
name: 'Lawson Luke',
email: '[email protected]',
password: '123',
}),
})
.then((response) => response.json())
.then((responseJson) => {
//Showing response message coming from server
console.warn(responseJson);
})
.catch((error) => {
//display error message
console.warn(error);
});
Like we explained earlier, the fetch() takes a mandatory url, which is usually the endpoint through which you want to make your request
The body takes in your parameters. The name, email and password are parameterised destination from your server which must also receive a corresponding input.
The response object returns back a response from the server and here you can proceed to the next logic of your application
The catch() handles any error that results from making the request. These errors could be shown to the users or they could handled nicely as you deem fit.
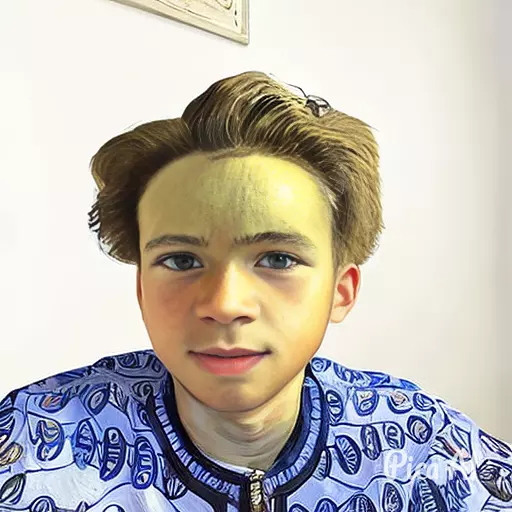