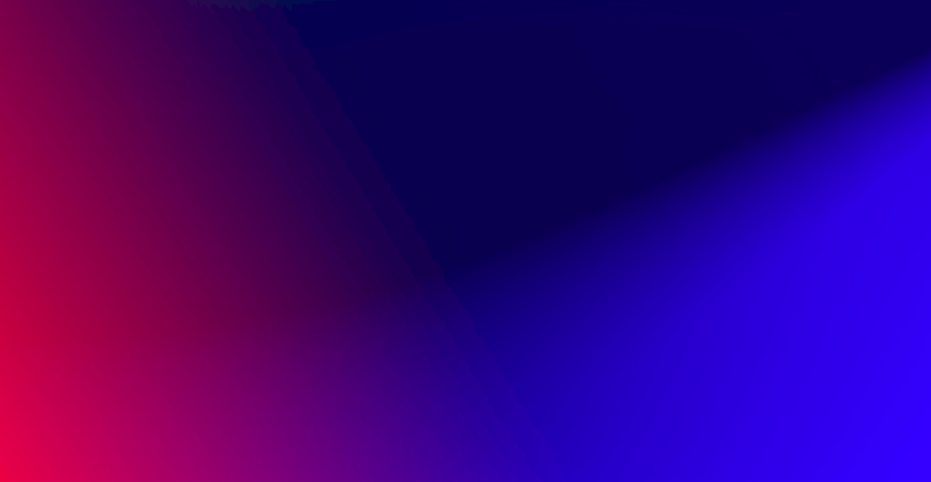
Add React Native gradient to your project.
A gradient is a smooth transition or mix of two or more colors or tones. It is a visual effect that generates a seamless transition from one color to the next, usually in a linear or radial pattern. Gradients are often employed to lend depth, dimension, and visual appeal to an image or layout in a variety of design applications such as graphic design, web design, and digital artwork.
In React Native, we can make a gradient design using the react-native-linear-gradient package. The first thing to do is to add this package to our project after which we add the import statement..
Take a look …
yarn add react-native-linear-gradient
React Native Gradient
Here’s the full code for the project …
import React, { Component } from 'react';
import { View, Text, StyleSheet } from 'react-native'
import LinearGradient from 'react-native-linear-gradient';
class App extends Component {
render(){
return(
<View style={styles.container}>
<Text style={styles.heading}>React Native Gradient</Text>
<View style={styles.gradientContainer}>
<LinearGradient style={styles.item} colors={['#fc4a1a', '#f7b733', '#192f6a']}>
<Text style={styles.text}>
Orange Fun
</Text>
</LinearGradient>
<LinearGradient style={styles.item} colors={['#e1eec3', '#f05053']}>
<Text>
Velvet Sun
</Text>
</LinearGradient>
<LinearGradient style={styles.item} colors={['#1a2a6c', '#b21f1f', '#fdbb2d']}>
<Text>
King Yna
</Text>
</LinearGradient>
<LinearGradient style={styles.item} colors={['#22c1c3', '#fdbb2d']}>
<Text>
Summer
</Text>
</LinearGradient>
</View>
</View>
)
}
}
const styles = StyleSheet.create({
gradientContainer: {
flex: 1,
width: '100%',
flexDirection: 'row',
justifyContent: 'space-evenly',
flexWrap: 'wrap',
marginTop: 36
},
item: {
width: 108,
height: 108,
marginBottom: 18,
alignItems: 'center',
justifyContent: 'center'
},
text: {
color: 'black'
},
heading: {
textAlign: 'center',
marginTop: 18,
fontSize: 27,
color: 'black',
fontWeight: 'bold'
}
})
export default App;
So in effect we’re just adding the gradient to the different boxes we created using flex-box. You can also choose whether or not you want a 2-or -3-color gradient design as shown in the result below.
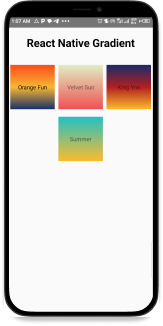
Conclusion
This is how to add gradient colors to your project. If you’re looking for how to add Google Places Autocomplete search to your React Native project, you can check out this article.