In React Native, an ActivityIndicator displays a loading circular mark which signals to the user that a task is being performed or a network request is being made.
Using ActivityIndicators in software development is a good practice and provides a good user experience to users of your application, and when you’re working with React Native apps, there’s a way to show such indication.
Let’s Get Started
The first thing to do is to import ActivityIndicator and other components like View and Text from React Native.
import React from "react";
import { ActivityIndicator, StyleSheet, Text, View } from "react-native";
Next, call your ActivityIndicator component like so.
import React from "react";
import { ActivityIndicator, StyleSheet, Text, View } from "react-native";
class App extends Component {
render(){
render(
<View>
<ActivityIndicator size="small" color="#0000ff" />
</View>
)
}
}
}
The size property tells whether the indicator should be “small” or “large”.
The color property defines the color of the indicator. If you fail to define a specific color, you might get some display on iOS, but you won’t see any rendering for Android.
Positioning ActivityIndicator
You can set position of your ActivityIndicator by defining some styles using the style tag.
import React from "react";
import { ActivityIndicator, StyleSheet, Text, View } from "react-native";
class App extends Component {
render(){
render(
<View>
<ActivityIndicator size="small"
style={{marginLeft: 0,
top: 0,
bottom: 0,
alignSelf: 'center',
right:0,
left:0
}}
color="#0000ff"
/>
</View>
)
}
}
}
export default App
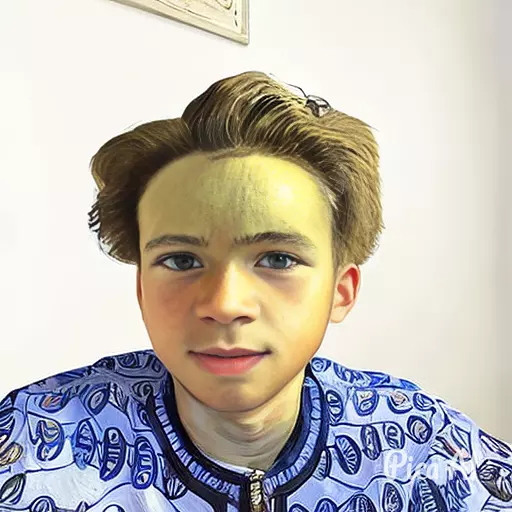