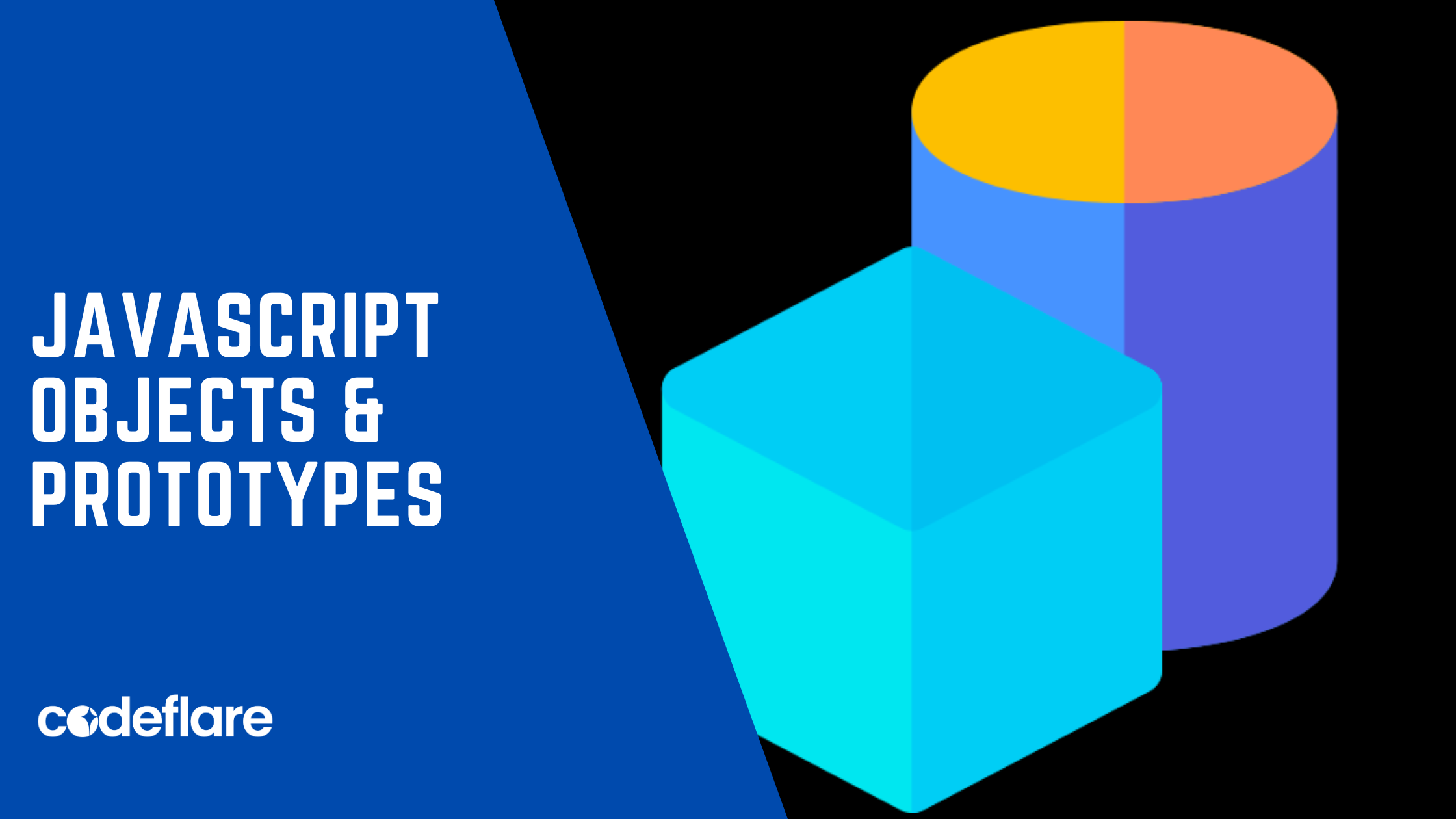
JavaScript, a language rooted in object-oriented programming, demands that you deeply understand how objects and prototypes function. This extensive guide not only unravels the fundamentals of JavaScript objects but also delves into the intricacies of prototypes. Through this exploration, we aim to empower you with the knowledge necessary to master the language’s capabilities.
Understanding JavaScript Objects
What are Objects?
JavaScript objects, dynamic data containers that facilitate the organization and storage of data, constitute a complex data type. These entities consist of key-value pairs, where each key, commonly a string or symbol, aligns with a value representing any data type, including other objects. Let’s now actively engage in creating a simple object to reinforce these concepts and enhance our understanding.
const person = {
name: 'John Doe',
age: 30,
address: {
city: 'Exampleville',
country: 'JSland',
},
sayHello: function() {
console.log(`Hello, I'm ${this.name}!`);
},
};
In this example, person
is an object with properties like name
, age
, and address
. The sayHello
property is a method, a function associated with the object.
Object Methods and this
Object methods, like sayHello
above, have access to the object’s properties using the this
keyword. The this keyword refers to the object the method is called on. Be cautious with arrow functions as they don’t bind their own this
but inherit it from the enclosing scope. Below is an Example!
const person = {
name: 'John Doe',
sayHello: () => {
console.log(`Hello, I'm ${this.name}!`); // Avoid using arrow functions here
},
};
person.sayHello(); // This will not work as expected
Prototypes in JavaScript
JavaScript operates on a prototype-based model. Each object associates with a prototype, another object from which it inherits properties and methods. A thorough understanding of prototypes is crucial for effective JavaScript development.
Creating Objects with Prototypes
When you create an object, JavaScript automatically links it to a prototype. You can create objects with a specific prototype using Object.create()
:
const animal = {
makeSound: function() {
console.log('Some generic sound');
},
};
const dog = Object.create(animal);
dog.makeSound(); // Outputs: Some generic sound
In this example, dog
is an object with a prototype set to the animal
object. The makeSound
method is inherited from the prototype.
Constructors and Prototypes
Constructors are functions used with the new
keyword to create objects. They can be associated with prototypes to define shared properties and methods:
function Person(name, age) {
this.name = name;
this.age = age;
}
Person.prototype.sayHello = function() {
console.log(`Hello, I'm ${this.name}!`);
};
const john = new Person('John Doe', 30);
john.sayHello(); // Outputs: Hello, I'm John Doe!
Here, Person
is a constructor function, and sayHello
is added to the Person
prototype. Objects created with new Person()
inherit the properties and methods from the Person
prototype.
ES6 Classes
ES6 introduced a more familiar syntax for working with prototypes through the ‘class
‘ keyword. However, it’s essential to understand that JavaScript classes are just syntactic sugar over prototypes.
class Animal {
constructor(sound) {
this.sound = sound;
}
makeSound() {
console.log(this.sound);
}
}
class Dog extends Animal {
constructor() {
super('Woof');
}
}
const myDog = new Dog();
myDog.makeSound(); // Outputs: Woof
In this example, Animal is a class with a method makeSound, and Dog is a subclass that extends Animal. Furthermore, the super keyword is used to call the constructor of the parent class. This ensures the proper initialization of the subclass while maintaining the inheritance hierarchy.
Conclusion
Mastering JavaScript objects and prototypes is crucial for crafting robust and maintainable code. Whether you prefer the classic prototype-based methodology or embrace the modern class syntax, understanding the principles behind objects and prototypes empowers you to create efficient and scalable JavaScript applications. Consequently, delve into the intricacies of prototypes, experiment with various object creation patterns, and enhance your JavaScript skills. In doing so, you’ll be well-equipped to build sophisticated and reliable applications. Happy coding!
Understanding JavaScript closures