JavaScript closures are a powerful and often misunderstood concept that plays a crucial role in the language’s ability to manage scope and encapsulate functionality. In this article, we’ll explore what closures are, how they work, provide examples of their use cases, and discuss both their benefits and potential pitfalls.
What are Closures?
In JavaScript, a closure is created when a function is defined inside another function (the outer function) and has access to the outer function’s variables. This inner function, even if executed outside the scope of the outer function, “closes over” the variables from the outer function, allowing it to retain access to them. In simpler terms, a closure allows a function to “remember” the environment in which it was created. This includes the variables, parameters and functions available in the outer Functions scope.
How Closures Work
Let’s delve into a simple example to understand how closures work:
function outerFunction() {
let outerVariable = 'I am from the outer function';
function innerFunction() {
console.log(outerVariable);
}
return innerFunction;
}
const closureFunction = outerFunction();
closureFunction(); // Logs 'I am from the outer function'
In this example, outerFunction
contains a variable outerVariable
and returns innerFunction
. When outerFunction
is called, it creates a closure by returning innerFunction
. The closureFunction
now has access to outerVariable
, even though outerFunction
has completed execution.
Use Cases of Closures
1. Data Encapsulation and Privacy
Closures are often used to create private variables and encapsulate data within a function. This helps in preventing unintended modifications to the internal state of an object.
function createCounter() {
let count = 0;
return function () {
count++;
console.log(count);
};
}
const counter = createCounter();
counter(); // Logs 1
counter(); // Logs 2
2. Factory Functions
Closures are instrumental in creating factory functions. These functions generate and return other functions with specific behavior based on the initial parameters.
function greet(prefix) {
return function (name) {
console.log(`${prefix}, ${name}!`);
};
}
const greetMorning = greet('Good morning');
greetMorning('John'); // Logs 'Good morning, John!'
Benefits of Closures
- Encapsulation: Closures allow for the creation of private variables, promoting encapsulation and preventing unintended external access.
- Data Persistence: Closures enable functions to retain access to variables, even after the outer function has completed execution.
- Dynamic Function Generation: Closures facilitate the creation of functions with dynamic behavior, enhancing flexibility in code design
Potential Pitfalls
- Memory Usage: Closures can lead to memory leaks if not managed properly. Variables from the outer function are retained in memory as long as the closure exists.
- Scope Chain Complexity: Deeply nested closures may lead to a complex scope chain, making code harder to read and debug.
Below is an example of how you can use HTML and JavaScript closures to create a simple counter application.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Counter App</title>
<style>
body {
font-family: Arial, sans-serif;
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
margin: 0;
}
button {
padding: 10px;
font-size: 16px;
margin: 0 10px;
}
</style>
</head>
<body>
<div>
<h1>Counter App</h1>
<p>Current Count: <span id="count">0</span></p>
<button onclick="increment()">Increment</button>
<button onclick="decrement()">Decrement</button>
</div>
<script>
// Closure to encapsulate the counter logic
const counterApp = (function () {
let count = 0; // Private variable
function updateCount() {
document.getElementById('count').innerText = count;
}
return {
increment: function () {
count++;
updateCount();
},
decrement: function () {
count--;
updateCount();
}
};
})();
function increment() {
counterApp.increment();
}
function decrement() {
counterApp.decrement();
}
</script>
</body>
</html>
Here is the result!
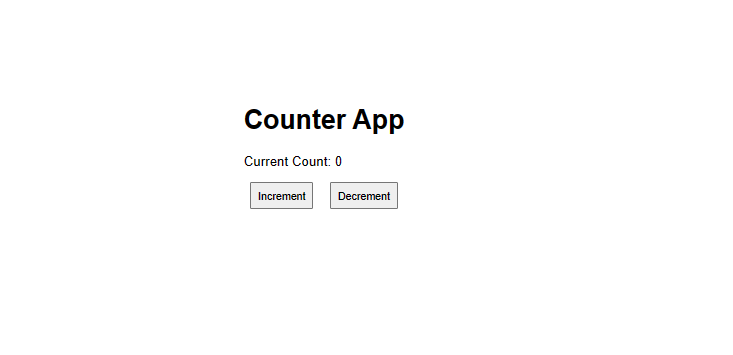
in this result, once you click on Decrement the Current Count decreases, the same applies to Increment.
Conclusion
Understanding JavaScript closures is crucial for mastering the language’s more advanced features. Closures provide an elegant solution for data encapsulation, dynamic function generation, and maintaining scope. Therefore, they offer numerous benefits. However, developers should be mindful of potential pitfalls to write efficient and maintainable code.
Exploring JavaScript variables: var, let, and const.