JavaScript, as a versatile programming language, provides multiple ways to declare variables. In this article, we’ll explore the var
, let
, and const
keywords, understand their differences, delve into the concept of variable hoisting, and discover the best practices for using each.
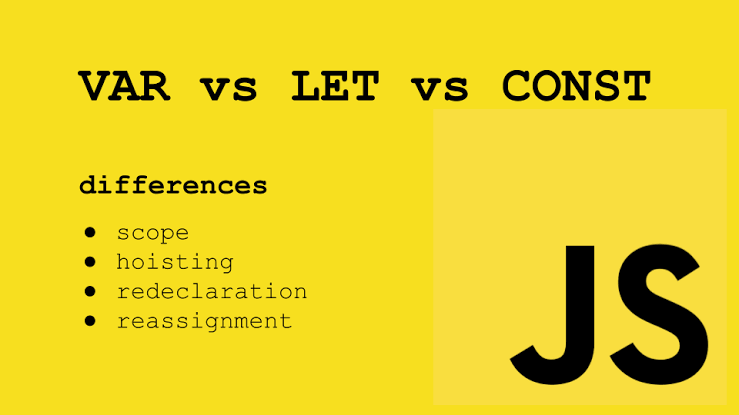
Variable Declaration:
Var:
var greeting = "Hello, World!";
let:
let count = 10;
const:
const PI = 3.14;
Differences:
- Scope:
var
has a function scope.let
andconst
have block scope.
- Hoisting:
- Variables declared with
var
are hoisted to the top of their scope. - Variables declared with
let
andconst
are hoisted but not initialized.
- Variables declared with
- Reassignment:
var
andlet
can be reassigned.const
variables cannot be reassigned.
Examples:
Variable Hoisting:
console.log(message); // undefined
var message = "Variable Hoisting";
// Results in ReferenceError
console.log(animal);
let animal = "Lion";
Reassignment:
var countVar = 5;
countVar = 8; // Valid
let countLet = 5;
countLet = 8; // Valid
const countConst = 5;
// Results in TypeError
countConst = 8;
Best Practices:
- Use
const
by default and only uselet
when reassignment is necessary. - Avoid using
var
due to its function scope and hoisting behavior.
Scenarios:
- Use
var
if compatibility with older browsers is required. - Use
let
for variables that need to be reassigned. - Use
const
for constants and variables that should not be reassigned.
Conclusion:
Understanding the differences between var
, let
, and const
is crucial for writing clean and maintainable JavaScript code. Embrace const
for immutability, use let
when reassignment is necessary, and limit the use of var
in modern JavaScript development. Consider variable hoisting and choose the appropriate variable declaration based on your specific use case.