We can pass parameters easily between two components in React. Passing data between components is a fundamental aspect of building dynamic and interactive applications.
In this article, we shall see how to pass data in one component and receive it in another component.
To begin, you have to first install react router, if you don’t already have it installed:
yarn add react-router-dom
Next, we create a file called RouteScreen.js. We’ll create two files and pass data between them.
import React from 'react'
import ( BrowserRouter as Router, Routes, Route } from 'react-router-dom'
import Home from './App':
import About from './About':
const RouteScreen = () => {
return(
<Router>
<div>
<Routes>
<Route exact path="/about" element={<About >} />
<Route exact path="/" element={<Home >} />
<Routes>
<div>
</Router>
)
}
export default RouteScreen;
Since we’re going to be passing parameter to our about component, we need to indicate that in our RouteScreen file.
<Route exact path="/about/:id" element={<About >} >
Next, we will define what type of data we want to pass and we will do that in our home component. You can decide to pass any data you want, but most commonly it is used to pass API parameters. For the sake of this tutorial we will pass a simple “hello”.
In our App.js file, we pass the following:
import React from 'react';
import {Link} from 'react-router-dom';
const App = () => {
return(
<div>
<Link to={`/discussion-description/hello`} className="col-sm-12">Pass Data</Link>
</div>
)
}
export default App;
Finally, we want to be able to retrieve the passed parameter in our other component. We do the following:
import React from 'react';
import {Link, useParams} from 'react-router-dom';
const About = () => {
const { id } = useParams();
return(
<div>
<h2>{id}</h2>
</div>
)
}
export default About;
Explanation
To retrieve the passed parameter from our parent component, we have to use useParams, which is a hook available in the React Router library. This is how to pass parameters easily between two components in React.
Earn Professional Certification
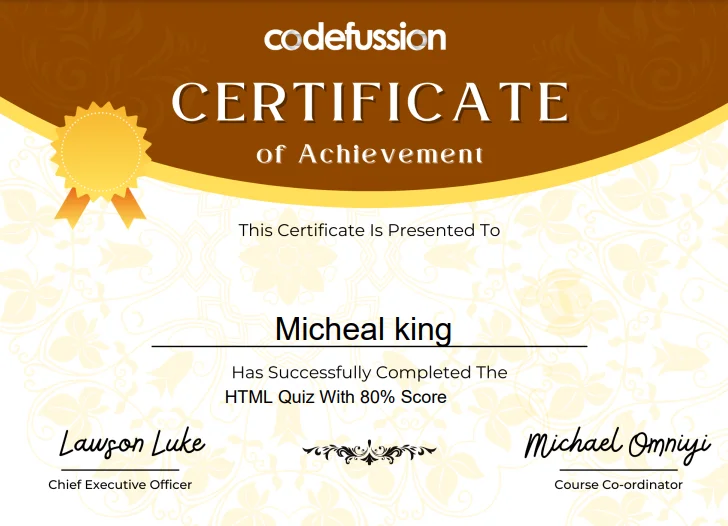
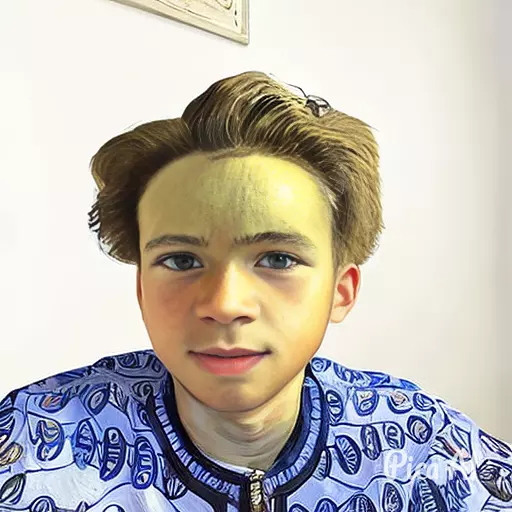