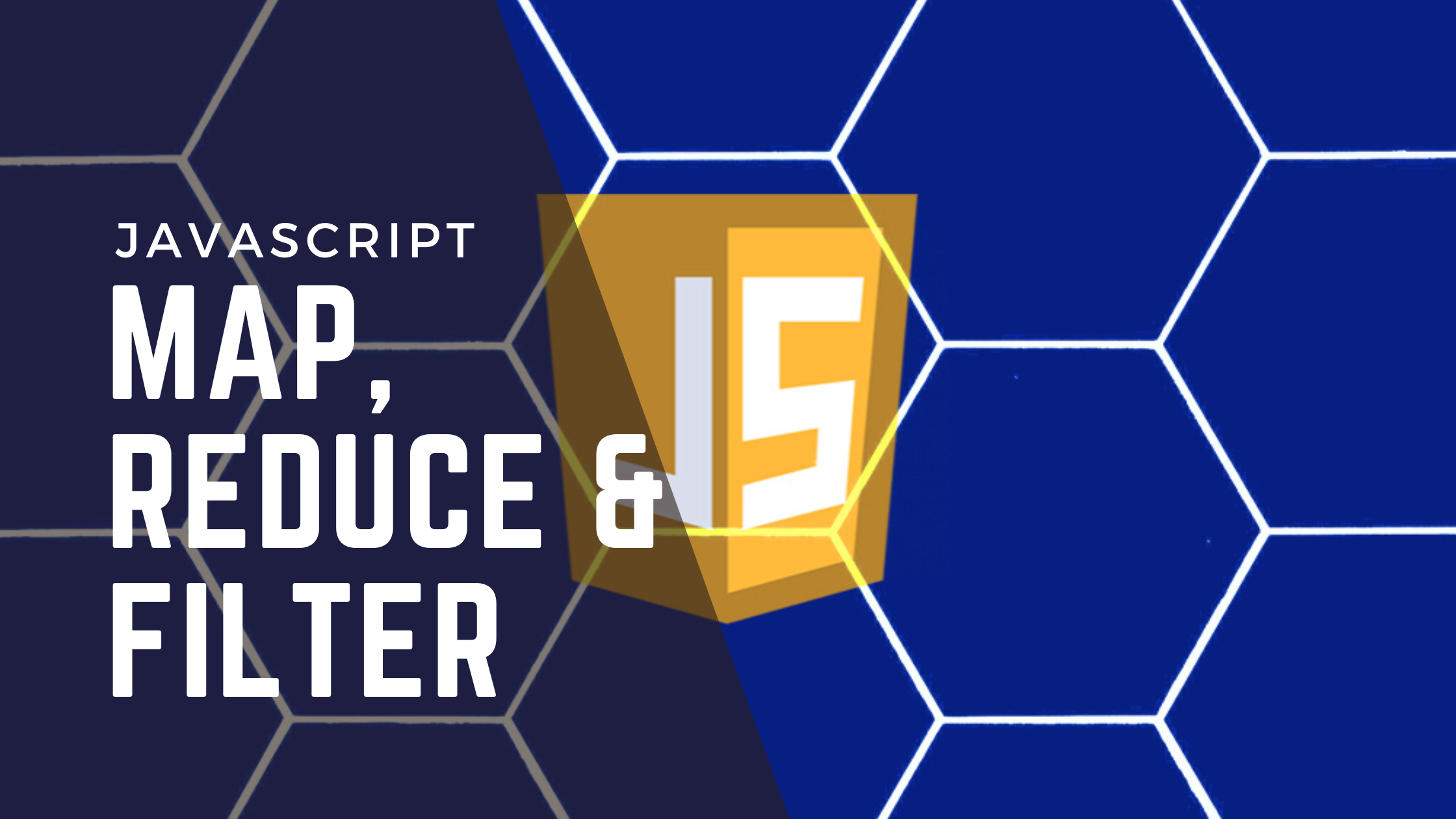
map
, reduce
, and filter
in JavaScript stand out as powerful array methods that allow software developers to transform, aggregate, and filter data efficiently.
In this comprehensive guide, we will explain each of these methods, exploring their use cases, syntax, and examples to help you master them.
Practical software development training in Abuja, Nigeria
Understanding Arrays in JavaScript
Before diving into the specifics of map
, reduce
, and filter
, let’s briefly revisit JavaScript arrays. Arrays are collections of values stored in a single variable. Each value is called an element, and they are accessed by their index. Arrays are incredibly versatile and are used to store and manipulate data in various ways.
The map
Method
The map
method is used to transform an array by applying a given function to each element in the array and returning a new array with the results. It doesn’t modify the original array, making it a non-destructive operation. The basic syntax of map
is as follows:
const newArray = array.map(callback(currentValue[, index[, array]]) {
// return element for newArray
});
callback
: The function to execute for each element.currentValue
: The current element being processed.index
(optional): The index of the current element.array
(optional): The arraymap
was called upon.
Let’s illustrate this with an example. Suppose we have an array of numbers, and we want to double each number:
const numbers = [1, 2, 3, 4, 5];
const doubledNumbers = numbers.map((num) => num * 2);
console.log(doubledNumbers); // Output: [2, 4, 6, 8, 10]
In this example, the map
method iterates through each element in the numbers
array, doubles it, and stores the results in the doubledNumbers
array.
Learn software development in Abuja, Nigeria
The reduce
Method
The reduce
method is used to reduce an array to a single value by applying a given function to accumulate values. It’s often used for tasks like summing up numbers, finding the maximum or minimum value, or concatenating strings. The basic syntax of reduce
is as follows:
const result = array.reduce(callback(accumulator, currentValue[, index[, array]])[, initialValue]);
callback
: The function to execute for each element.accumulator
: The accumulator accumulates the callback’s return values; it is the accumulated result.currentValue
: The current element being processed.index
(optional): The index of the current element.array
(optional): The arrayreduce
was called upon.initialValue
(optional): A value to use as the first argument to the first call of the callback function.
Let’s use an example to illustrate how reduce
works. Suppose we have an array of numbers, and we want to find their sum:
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce((accumulator, num) => accumulator + num, 0);
console.log(sum); // Output: 15
In this example, the reduce
method starts with an initial value of 0
for the accumulator. It then iterates through each element in the numbers
array, adding the current element’s value to the accumulator.
The filter
Method
The filter
method is used to create a new array with all elements that pass a given test. It doesn’t modify the original array, similar to map
. The basic syntax of filter
is as follows:
const newArray = array.filter(callback(element[, index[, array]]) {
// return true to keep the element, false to discard it
});
callback
: The function to execute for each element.element
: The current element being processed.index
(optional): The index of the current element.array
(optional): The arrayfilter
was called upon.
Let’s see an example of how to use filter
. Suppose we have an array of numbers, and we want to filter out all even numbers:
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter((num) => num % 2 === 0);
console.log(evenNumbers); // Output: [2, 4]
In this example, the filter
method checks each element in the numbers
array and keeps only the elements that satisfy the condition (num % 2 === 0
), resulting in an array containing only the even numbers.
Practical Use Cases
Now that we’ve covered the basics of map
, reduce
, and filter
, let’s explore some practical use cases for each of these methods.
Practical Use of map
- Data Transformation: When you have an array of data and need to transform it into a new format,
map
is your go-to method. For example, you can convert an array of objects into an array of specific properties:
const people = [
{ name: 'Alice', age: 30 },
{ name: 'Bob', age: 25 },
{ name: 'Charlie', age: 35 },
];
const names = people.map((person) => person.name);
console.log(names); // Output: ['Alice', 'Bob', 'Charlie']
2. Generating JSX in React: In React, you often use map
to generate JSX elements dynamically. For instance, creating a list of components based on an array of data:
const items = ['Item 1', 'Item 2', 'Item 3'];
const itemList = items.map((item, index) => (
<li key={index}>{item}</li>
));
// Render `itemList` in your React component.
Practical Use of reduce
- Summing Values: As shown earlier,
reduce
is perfect for summing an array of numbers:
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce((accumulator, num) => accumulator + num, 0);
console.log(sum); // Output: 15
2. Finding Maximum or Minimum Value: You can use reduce
to find the maximum or minimum value in an array:
const values = [7, 2, 10, 4, 1];
const max = values.reduce((maxValue, current) => (current > maxValue ? current : maxValue), values[0]);
console.log(max); // Output: 10
3. Concatenating Strings: To concatenate all elements of an array into a single string:
const words = ['Hello', ' ', 'world', '!'];
const sentence = words.reduce((fullSentence, word) => fullSentence + word, '');
console.log(sentence); // Output: 'Hello world!'
Practical Use of filter
- Filtering Data: Filtering an array to include only elements that meet specific criteria is a common task. For instance, filtering out inactive users from a list of users:
const users = [
{ name: 'Alice', isActive: true },
{ name: 'Bob', isActive: false },
{ name: 'Charlie', isActive: true },
];
const activeUsers = users.filter((user) => user.isActive);
console.log(activeUsers);
Searching and Retrieving Data: When you want to retrieve specific elements from an array based on a condition, filter
is a handy tool. For example, finding products with a certain price range:
const products = [
{ name: 'Laptop', price: 800 },
{ name: 'Phone', price: 500 },
{ name: 'Tablet', price: 300 },
{ name: 'Monitor', price: 250 },
];
const affordableProducts = products.filter((product) => product.price < 400);
console.log(affordableProducts);
Chaining Methods
One of the great advantages of these array methods is that they can be chained together to perform complex operations on arrays. Chaining allows you to combine map
, reduce
, and filter
to achieve more advanced data manipulations. Here’s an example:
see also JavaScript optional chaining
const numbers = [1, 2, 3, 4, 5];
const result = numbers
.filter((num) => num % 2 === 0) // Filter even numbers
.map((num) => num * 2) // Double each remaining number
.reduce((sum, num) => sum + num, 0); // Sum the results
console.log(result); // Output: 12
In this example, we first filter the even numbers, then double each of them using map
, and finally, we use reduce
to find their sum.
In this software development training in Abuja, Nigeria, you will learn how to build functional mobile and websites.
Conclusion
map
, reduce
, and filter
in JavaScript are powerful array methods that allow you to manipulate and transform data with ease. By mastering these methods, you can write more efficient and concise code for various tasks, from data transformation to aggregation and filtering.
To become proficient with these methods, practice is essential. Experiment with different scenarios and data sets to gain a deeper understanding of how map
, reduce
, and filter
can simplify your JavaScript programming tasks. These methods are not only valuable in JavaScript but also in other programming languages, making them a fundamental skill for any developer.