Among its many features, the useEffect
hook stands out as a critical component for managing side effects and handling lifecycle events within a React component.
In this comprehensive guide, we will delve deep into the world of useEffect
in React, understanding and exploring its purpose, syntax, common use cases, and best practices.
What is the useEffect Hook?
Before we dive into the intricacies of useEffect
, it’s crucial to grasp its fundamental purpose. At its core, useEffect
enables you to perform side effects in your functional components. Side effects can encompass a wide range of operations, such as data fetching, DOM manipulation, and subscriptions. In class components, these tasks were typically handled in methods like componentDidMount
and componentDidUpdate
. useEffect
simplifies and unifies this functionality in functional components.
Syntax and Basics
The basic syntax of the useEffect
hook looks like this:
import React, { useState, useEffect } from 'react';
function MyComponent() {
// State initialization and other component logic here
useEffect(() => {
// Side effect code goes here
}, [dependency1, dependency2]);
return (
// JSX rendering
);
}
useEffect
accepts two arguments: a function containing your side effect code and an optional array of dependencies.- The function inside
useEffect
will be executed after the component has rendered. - The dependencies array is a crucial part of optimizing your component’s performance. When one or more dependencies in the array change, the side effect function is re-executed. If you omit this array, the function runs after every render.
Now, let’s explore the practical applications of useEffect
by examining some common use cases.
Common Use Cases for useEffect
1. Data Fetching
Fetching data from APIs or other sources is one of the most common use cases for useEffect
. Here’s an example of how you might use it to fetch data when a component mounts:
import React, { useState, useEffect } from 'react';
function DataFetchingComponent() {
const [data, setData] = useState(null);
useEffect(() => {
// Fetch data here
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => setData(data))
.catch(error => console.error('Error fetching data:', error));
}, []); // Empty dependency array means this runs once when the component mounts
return (
<div>
{data ? (
// Render data here
) : (
// Render loading or error message
)}
</div>
);
}
In this example, the data fetching code runs when the component mounts. The empty dependency array ensures that it runs only once.
2. Managing Subscriptions
useEffect
can also be used to manage subscriptions, such as WebSocket connections. When the component mounts, you can establish the subscription, and when it unmounts, you can clean it up to prevent memory leaks.
import React, { useState, useEffect } from 'react';
function WebSocketComponent() {
const [message, setMessage] = useState('');
useEffect(() => {
// Establish a WebSocket connection
const socket = new WebSocket('wss://example.com/socket');
// Listen for incoming messages
socket.addEventListener('message', event => {
setMessage(event.data);
});
// Clean up the WebSocket when the component unmounts
return () => {
socket.close();
};
}, []); // Empty dependency array for mounting and unmounting
return (
<div>
<p>Received message: {message}</p>
</div>
);
}
In this example, the WebSocket connection is established when the component mounts, and it’s closed when the component unmounts, preventing potential memory leaks.
3. Reacting to Dependency Changes
When you want to run a side effect in response to changes in one or more dependencies, you can include those dependencies in the array passed as the second argument to useEffect
. This ensures that the side effect code runs whenever the specified dependencies change.
import React, { useState, useEffect } from 'react';
function DependencyChangeComponent({ count }) {
const [message, setMessage] = useState('');
useEffect(() => {
// Run this when the 'count' prop changes
setMessage(`Count changed to ${count}`);
}, [count]); // 'count' is a dependency
return (
<div>
<p>{message}</p>
</div>
);
}
In this example, the message is updated whenever the count
prop changes, thanks to the dependency array [count]
.
4. Performing Cleanup
useEffect
allows you to perform cleanup when a component unmounts or when dependencies change. For instance, if you’re using a library that requires cleanup, you can include the cleanup logic in the return function of your useEffect
.
import React, { useState, useEffect } from 'react';
function CleanupComponent() {
useEffect(() => {
// Initialization code
return () => {
// Cleanup code (e.g., clearing timers or subscriptions)
};
}, []); // Empty dependency array for mounting and unmounting
return (
// Component rendering
);
}
In this example, any cleanup code is executed when the component unmounts.
Best Practices and Tips
As you start using useEffect
in your React applications, consider these best practices to ensure efficient and maintainable code:
1. Declare Dependencies Accurately
Ensure that your dependencies accurately represent the values that your effect relies on. Declaring unnecessary dependencies can lead to performance issues, while omitting necessary dependencies can cause bugs.
2. Use Multiple useEffect
Hooks
Don’t hesitate to use multiple useEffect
hooks within a single component. This allows you to separate concerns and keep your code organized.
3. Avoid Infinite Loops
Be cautious when using dependencies that change frequently, as this can trigger an infinite loop of re-renders. Make sure your code doesn’t inadvertently create such a loop.
4. Extract Reusable Logic
If you find yourself repeating the same side effect logic in multiple components, consider extracting it into a custom hook for reusability.
5. Test Effectful Code
Don’t forget to test the code inside your useEffect
functions. Testing helps catch potential issues early in the development process.
Conclusion
The useEffect
hook is a fundamental tool in the React developer’s toolbox, enabling you to manage side effects, handle lifecycle events, and create dynamic and responsive web applications. By understanding its syntax, common use cases, and best practices, you can harness the full power of useEffect
to build robust and efficient React components. As you continue to explore React and its ecosystem, you’ll find useEffect
to be an invaluable resource for your web development journey.
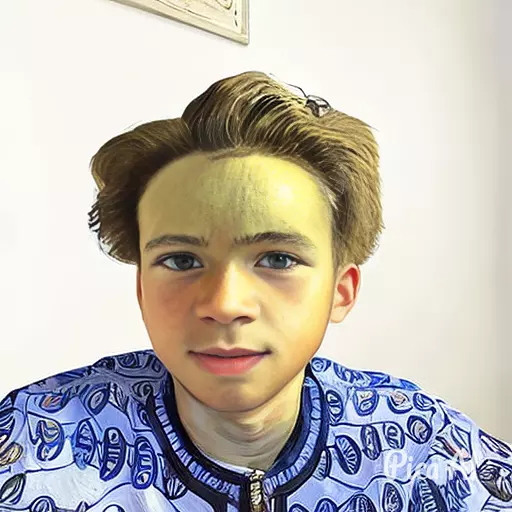