Sometimes when trying to access the properties of an object especially if you’re fetching data from an endpoint, you can be confronted with the code-breaking error: UNDEFINED.
See also Null, undefined, and not defined
This is where optional chaining comes in.
What is Optional Chaining?
Optional chaining allows you to access properties and methods of an object without the need to validate the existence of each property or method in the chain explicitly. It provides a more concise and error-resistant way to traverse deep object structures, avoiding the dreaded “Cannot read property ‘x’ of undefined” errors.
Before the introduction of optional chaining, developers typically used conditional statements or ternary operators to check if each property in a chain exists before accessing it. This approach was verbose and often resulted in nested conditionals, making code harder to read and maintain. Optional chaining simplifies this process.
The Syntax of Optional Chaining
The syntax for optional chaining involves using the question mark (?
) immediately before the dot (.
) or square bracket ([]
) notation when accessing properties or methods. Here’s a basic example:
const person = {
name: "John",
address: {
city: "New York",
},
};
const city = person.address?.city;
console.log(city); // "New York"
In this example, we access the city
property of the address
object using optional chaining. If the address
object is null
or undefined
, the result will be undefined
, but no error will be thrown.
Use Cases for Optional Chaining
Optional chaining is incredibly useful in various scenarios, especially when dealing with complex object structures and data fetched from APIs or external sources. Let’s explore some common use cases.
1. Safely Accessing Nested Object Properties
When working with nested objects, optional chaining simplifies property access:
const user = {
profile: {
email: "[email protected]",
},
};
const email = user.profile?.email;
console.log(email); // "[email protected]"
const phoneNumber = user.profile?.phoneNumber;
console.log(phoneNumber); // undefined
Without optional chaining, you would need to check each nested property manually, resulting in more code and reduced readability.
2. Accessing Properties in Arrays of Objects
Optional chaining can also be handy when working with arrays of objects. Consider the following example:
const users = [
{ id: 1, name: "Alice" },
{ id: 2, name: "Bob", address: { city: "Paris" } },
{ id: 3, name: "Charlie" },
];
const city = users[1]?.address?.city;
console.log(city); // "Paris"
const missingCity = users[0]?.address?.city;
console.log(missingCity); // undefined
Optional chaining allows you to gracefully handle cases where certain properties might be missing in some objects within the array.
3. Function Calls with Optional Chaining
You can also use optional chaining with function calls. This is particularly useful when you want to call a function on an object that may not exist:
const order = {
items: [
{ id: 1, name: "Widget", getPrice: () => 10 },
{ id: 2, name: "Gadget" },
],
};
const price = order.items[0]?.getPrice?.();
console.log(price); // 10
const missingPrice = order.items[1]?.getPrice?.();
console.log(missingPrice); // undefined
In this example, we use optional chaining to call the getPrice
method on the second item, which doesn’t have the method defined.
Benefits of Optional Chaining
Now that we’ve explored some common use cases for optional chaining, let’s delve into the benefits it brings to your JavaScript code.
1. Improved Readability
Optional chaining makes your code more concise and readable. It eliminates the need for verbose conditional checks, reducing clutter and making your codebase more maintainable.
2. Error Prevention
By avoiding explicit property checks, optional chaining helps prevent “Cannot read property ‘x’ of undefined” errors. It gracefully handles cases where properties or methods are missing, allowing your code to continue execution without interruption.
3. Simplified Code
Optional chaining simplifies your code, reducing the need for nested conditionals or ternary operators. This simplicity leads to cleaner and more understandable code.
4. Enhanced API Consumption
When working with external APIs or data sources, you often encounter varying data structures. Optional chaining helps you navigate these structures without extensive validation, making it easier to integrate and work with external data.
Browser Support
As of September 2021, optional chaining is supported in modern web browsers, including Chrome, Firefox, Edge, and Safari. However, it may not be supported in older versions of these browsers. To ensure cross-browser compatibility, consider using a transpiler like Babel to convert your modern JavaScript code, including optional chaining, into an older version that can be executed in older browsers.
Conclusion
JavaScript optional chaining is a valuable addition to the language, simplifying property access and improving code readability. It offers a concise and error-resistant way to traverse complex object structures, making your code more robust and easier to maintain. By embracing optional chaining, you can write cleaner, more efficient code while reducing the risk of common runtime errors. As you continue to explore JavaScript and its evolving features, consider incorporating optional chaining into your coding practices to enhance your development workflow.
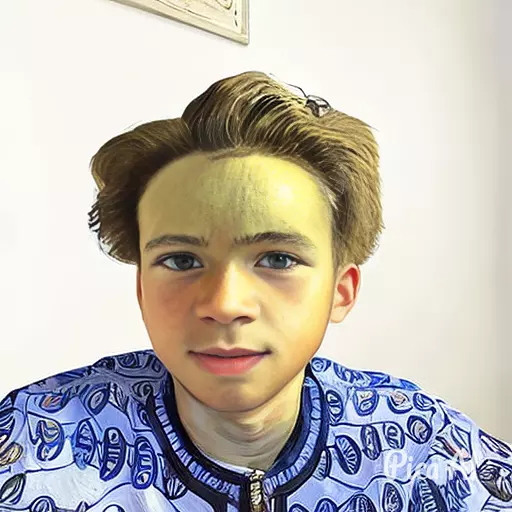