In the context of frontend web development, a functional component is a fundamental building block in many modern JavaScript frameworks and libraries. It is a type of component used in frameworks like React and Vue.js, and it can also refer to a similar concept in other programming paradigms, like functional programming.
In the context of React (which is one of the most popular JavaScript libraries that we are dealing), a functional component is a JavaScript function that returns JSX (JavaScript XML) to define the structure and appearance of a user interface element.
You could choose to use either class or functional components in rendering your JSX. For this particular example, we have already demonstrated the “class” example using React Native. See this article
In this tutorial, we are going to fetch data from an endpoint and display the results on our page using, of course, functional components as well as bootstrap for styling.
We are going to be fetching data from this endpoint https://catfact.ninja/breeds . It’s a GET Request and requires no access token or authorization.
The first thing we want to do is create a new react project and add our code. In our App.js or App.tsx, whichever one applies to you, we’re going to add the following:
import { useState, useEffect } from 'react';
const App = () => {
return(
<div>
</div>
}
export default App
Next, we want to have a function that fetches data from the given endpoint. We will create a function and call it fetchItems().
Let us fetch data from the endpoint in React JS.
const fetchItem = () => {
requestAnimationFrame(() =>
fetch(`https://catfact.ninja/breeds`, {
method: 'GET',
})
.then(response => response.json())
.then(responseJson => {
setData(responseJson.data)
console.warn(responseJson.data);
})
.catch(error => {
{
alert(error)
}
}),
);
}
Next, we need to show this data to see that at least we’re getting a response. So we will u

Next, we need to render that data in a readable and presentable format. We will create a function called showData() and add the following code:
const showData = () => {
return data.map(item => {
return(
<div class="col-sm-3">
<div class="card" style={{width: "18rem"}}>
<img src={image} class="card-img-top" alt="..." />
<div class="card-body">
<p class="card-text">Breed: {item.breed}.</p>
<p class="card-text">Coat: {item.coat}.</p>
<p class="card-text">Coat: {item.country}.</p>
<p class="card-text">Origin: {item.origin}.</p>
<p class="card-text">Patter: {item.pattern}.</p>
</div>
</div>
</div>
)
})
}
So, if you can see from the response, this endpoint does not really have an image for the various dog breeds, so I’m putting a placeholder dog image in the image section. Go ahead and use any placeholder image of your choice.
Next, we want to render our main JSX in the return section as follows:
return(
<div class="container">
<div class="row">
{showData()}
</div>
</div>
)
Here’s the full working code:
import { useState, useEffect } from 'react';
import image from './assets/images/happy.png';
import Nav from './Nav'
const Home = () => {
const [data, setData] = useState([]);
useEffect(() => {
fetchItem()
},[])
const fetchItem = () => {
requestAnimationFrame(() =>
fetch(`https://catfact.ninja/breeds`, {
method: 'GET',
})
.then(response => response.json())
.then(responseJson => {
setData(responseJson.data)
console.warn(responseJson.data);
})
.catch(error => {
{
alert(error)
}
}),
);
}
const showData = () => {
return data.map(item => {
return(
<div class="col-sm-3">
<div class="card" style={{width: "18rem"}}>
<img src={image} class="card-img-top" alt="..." />
<div class="card-body">
<p class="card-text">Breed: {item.breed}.</p>
<p class="card-text">Coat: {item.coat}.</p>
<p class="card-text">Coat: {item.country}.</p>
<p class="card-text">Origin: {item.origin}.</p>
<p class="card-text">Patter: {item.pattern}.</p>
</div>
</div>
</div>
)
})
}
return(
<div class="container">
<div class="row">
{showData()}
</div>
</div>
)
}
export default Home

This is what our code looks like and this is how we fetch data from an api in react js. I hope it was helpful. Give me your feedback.
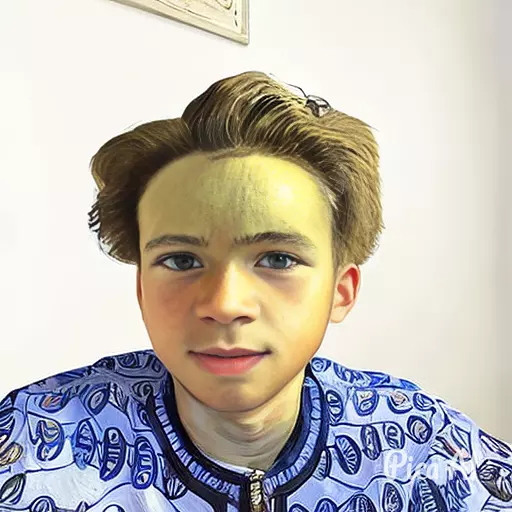