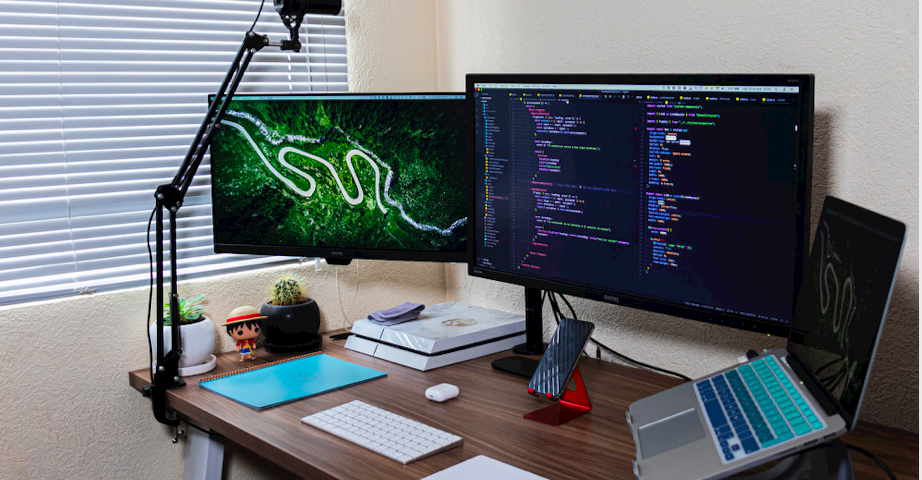
JavaScript, one of the most widely used programming languages for web development, is known for its flexibility and dynamic nature. However, this very flexibility can lead to some unexpected behavior when it comes to type coercion and comparison. In this article, we’ll delve deep into JavaScript’s type coercion and comparison mechanisms, demystifying the quirks and showcasing best practices to write robust and predictable code.
Understanding Type Coercion
Type coercion, often referred to as type conversion, is the process of converting a value from one data type to another. JavaScript performs type coercion implicitly and explicitly, depending on the context.
Implicit Type Coercion
Implicit type coercion occurs when JavaScript automatically converts values from one data type to another during operations or comparisons. This automatic conversion can sometimes lead to unexpected results if not understood correctly. Let’s explore some common scenarios where implicit type coercion takes place:
1. String Concatenation
Consider the following code:
let num = 5;
let str = "10";
let result = num + str;
In this example, num
is a number, and str
is a string. When we add them together, JavaScript implicitly converts num
to a string and performs string concatenation. The value of result
will be the string "510"
, not 15
.
2. Numeric Operations
JavaScript will try to convert operands to numbers when you perform numeric operations. For instance:
let num = 10;
let str = "5";
let result = num - str;
Here, str
is converted to a number, and the subtraction operation results in 5
, not "5"
.
3. Truthy and Falsy Values
JavaScript has a concept of truthy and falsy values. Not all values are strictly true or false in boolean context. Some values are considered “falsy,” meaning they evaluate to false
, while others are “truthy,” meaning they evaluate to true
. For instance:
if ("hello") {
console.log("This will be executed.");
}
if (0) {
console.log("This will not be executed.");
}
In the first if
statement, the string "hello"
is a truthy value, so the message will be logged. In the second if
statement, the number 0
is a falsy value, so the message will not be logged.
Explicit Type Coercion
Explicit type coercion, also known as type casting, occurs when you deliberately convert a value from one data type to another using functions or operators provided by JavaScript. This allows you to have more control over the type conversion process. Here are some common examples:
1. parseInt()
and parseFloat()
You can explicitly convert strings to numbers using parseInt()
and parseFloat()
functions:
let str = "42";
let num = parseInt(str);
In this case, the string "42"
is explicitly converted to the number 42
.
2. Number()
The Number()
function can also be used for explicit type conversion:
let str = "3.14";
let num = Number(str);
This code will convert the string "3.14"
to the number 3.14
.
3. String()
To convert a value to a string, you can use the String()
function:
let num = 42;
let str = String(num);
Here, the number 42
is explicitly converted to the string "42"
.
The Importance of Understanding Type Coercion
Understanding when and how type coercion occurs is crucial for writing JavaScript code that behaves predictably. It helps you avoid unexpected bugs and ensures that your code works as intended.
Consider the following example:
let num1 = 5;
let num2 = "10";
if (num1 === num2) {
console.log("Equal");
} else {
console.log("Not equal");
}
In this code, num1
is a number, and num2
is a string. If you expect them to be equal because the values are 5
and "10"
, respectively, you might be surprised to see “Not equal” in the console. This happens because ===
performs a strict equality comparison, which checks not only the values but also the data types. Since num1
is a number and num2
is a string, they are not strictly equal.
JavaScript Comparison Operators
JavaScript provides a set of operators for comparing values. These operators allow you to make decisions in your code based on whether certain conditions are true or false. Understanding how these operators work, especially in conjunction with type coercion, is crucial for writing reliable code.
Equality (==) and Inequality (!=) Operators
The equality (==
) and inequality (!=
) operators are used to compare values for equality or inequality. These operators perform type coercion if the types of operands are different. Let’s explore some examples:
Equality Operator (==)
5 == "5"; // true
In this example, JavaScript performs type coercion, converting the string "5"
to the number 5
before making the comparison. As a result, the expression evaluates to true
.
Inequality Operator (!=)
5 != "6"; // true
Similarly, the inequality operator !=
also performs type coercion, converting the string "6"
to the number 6
before making the comparison, which evaluates to true
.
Strict Equality (===) and Strict Inequality (!==) Operators
To avoid type coercion and ensure that both the values and data types match, JavaScript provides the strict equality (===
) and strict inequality (!==
) operators. These operators are considered best practice for most comparisons:
Strict Equality Operator (===)
5 === "5"; // false
In this case, ===
performs a strict comparison without type coercion, and since the data types of the operands are different (number
and string
), the expression evaluates to false
.
Strict Inequality Operator (!==)
5 !== "5"; // true
Similarly, the strict inequality operator !==
performs a strict comparison without type coercion and evaluates to true
because the data types are different.
Relational Operators (<, >, <=, >=)
JavaScript also provides relational operators for comparing values numerically:
<
(less than)>
(greater than)<=
(less than or equal to)>=
(greater than or equal to)
These operators perform type coercion when comparing different data types:
10 > "5"; // true
Here, JavaScript coerces the string "5"
to a number before performing the comparison, resulting in true
.
Common Pitfalls and Best Practices
Understanding JavaScript’s type coercion and comparison operators is essential for writing robust and predictable code. Here are some common pitfalls to avoid and best practices to follow:
1. Use Strict Equality and Strict Inequality
In most cases, it’s a good practice to use strict equality (===
) and strict inequality (!==
) to avoid unexpected type coercion. These operators provide more predictable results, especially when dealing with different data types.
2. Be Explicit in Type Conversion
When you need to convert a value from one data type to another, be explicit about it. Use functions like parseInt()
, parseFloat()
, Number()
, and String()
to ensure that type conversion is performed as intended.
3. Use Parentheses for Clarity
When you have complex expressions involving different operators, use parentheses to make your code more readable and to explicitly indicate the order of operations.
4. Be Mindful of Truthy and Falsy Values
Understand the concept of truthy and falsy values in JavaScript. This knowledge is crucial when writing conditional statements and handling values that might evaluate to false
in boolean context.
5. Test and Review Your Code
Always test your code thoroughly and review it for potential issues related to type coercion and comparison. Code review by peers can help catch unexpected behavior.
Conclusion
JavaScript type coercion and comparison mechanisms can be both powerful and tricky. While they allow for flexibility and ease of use, they can also lead to unexpected results if not handled carefully. By understanding how type coercion works, using the appropriate comparison operators, and following best practices, you can write JavaScript code that is more reliable and less prone to bugs.
In the dynamic world of web development, mastering these concepts is essential for creating robust and predictable applications. So, embrace the nuances of JavaScript’s type coercion and comparison, and use them to your advantage in building better web experiences.