In the world of web development, forms are ubiquitous. Whether you’re building a simple contact form or a complex e-commerce checkout page, user input is essential. However, with great power comes great responsibility, and handling user input securely and efficiently is crucial. JavaScript form validation is a vital part of this process.
Form validation refers to the process of ensuring that the data entered by a user into a web form meets certain criteria before it is submitted to the server for processing. Without proper validation, your application may accept incorrect or malicious data, leading to security vulnerabilities or poor user experiences.
In this article, we’ll explore JavaScript form validation, covering its importance, the different types of validation, and how to implement it effectively.
The Importance of Form Validation
Form validation serves several critical purposes in web development:
1. Data Accuracy
One of the primary objectives of form validation is to ensure that the data submitted is accurate and conforms to expected formats. For instance, if you have a registration form, you want to make sure that users enter valid email addresses, passwords that meet security requirements, and other relevant information correctly. Valid data reduces errors and ensures that the data stored in your application’s database is consistent and reliable.
2. Security
Without proper validation, forms can be an entry point for attackers to exploit vulnerabilities in your application. Malicious users can inject code, submit harmful data, or perform actions that compromise the security of your system. By validating user input on the client side (using JavaScript) and the server side, you can prevent common security threats like SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF).
3. User Experience
Good form validation improves the overall user experience by providing immediate feedback to users. Instead of submitting a form and waiting for the server to return an error message, users are informed of any issues in real-time, allowing them to correct their input quickly. This instant feedback reduces frustration and encourages users to complete the form accurately.
4. Data Consistency
Validation helps maintain data consistency by enforcing predefined rules. For example, if you’re collecting dates of birth, you can ensure that users enter a valid date and format, making it easier to analyze and display the data consistently throughout your application.
Types of Form Validation
Form validation can be divided into two main categories: client-side validation and server-side validation. Both are essential, and each serves a unique purpose in ensuring the integrity and security of user-submitted data.
1. Client-Side Validation
Client-side validation is performed on the user’s device, typically in the web browser, using JavaScript. It provides instant feedback to users as they fill out a form, improving the user experience. However, it should be noted that client-side validation alone is not sufficient for security purposes, as it can be bypassed or manipulated by knowledgeable users.
Here are some common client-side validation techniques:
a. Required Fields
Ensure that mandatory fields are not left empty. This can be done by checking if the input elements have values.
function validateForm() {
var name = document.forms["myForm"]["name"].value;
if (name == "") {
alert("Name must be filled out");
return false;
}
}
b. Format Validation
Check if the input data matches the expected format. For example, validate email addresses, phone numbers, or postal codes.
function validateEmail(email) {
var regex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
return regex.test(email);
}
if (!validateEmail(emailInput.value)) {
alert("Please enter a valid email address");
return false;
}
c. Length and Complexity Validation
Ensure that passwords meet specific length and complexity requirements.
function validatePassword(password) {
return password.length >= 8 && /[A-Z]/.test(password) && /\d/.test(password);
}
if (!validatePassword(passwordInput.value)) {
alert("Password must be at least 8 characters long and include an uppercase letter and a digit");
return false;
}
2. Server-Side Validation
Server-side validation is the ultimate line of defense. It occurs on the server after the form data has been submitted. While client-side validation improves user experience, server-side validation is essential for security, as it cannot be bypassed by users.
Here’s how server-side validation works:
- The client submits the form data to the server.
- The server validates the data received, checking it against predefined rules and requirements.
- If the data is valid, it is processed; otherwise, an error response is sent back to the client.
Server-side validation is crucial for preventing attacks like SQL injection, where malicious SQL queries are inserted into form fields to manipulate the database.
Implementing JavaScript Form Validation
Now that we understand the importance of form validation and the two main types, let’s explore how to implement JavaScript form validation in your web applications.
1. HTML Forms
Before you can validate a form, you need to create one using HTML. Here’s a basic example of an HTML form:
<form name="myForm" onsubmit="return validateForm()">
<label for="name">Name:</label>
<input type="text" id="name" name="name"><br><br>
<label for="email">Email:</label>
<input type="text" id="email" name="email"><br><br>
<input type="submit" value="Submit">
</form>
In this example, we have a simple form with two fields (name and email) and a submit button. The onsubmit
attribute of the <form>
element specifies the JavaScript function (validateForm()
) that will be called when the form is submitted.
2. JavaScript Validation Function
Next, let’s create the JavaScript function (validateForm()
) that will validate the form data. This function should return false
to prevent the form from being submitted if validation fails.
function validateForm() {
var name = document.forms["myForm"]["name"].value;
var email = document.forms["myForm"]["email"].value;
if (name == "") {
alert("Name must be filled out");
return false;
}
if (email == "") {
alert("Email must be filled out");
return false;
}
return true;
}
In this function, we retrieve the values of the name and email fields using the document.forms
object. We then check if these fields are empty and display an alert message if they are. If either field is empty, the function returns false
, preventing the form from being submitted.
3. Testing the Validation
Now, when a user submits the form with empty fields, they will receive alert messages indicating the required fields.
However, this is a basic example of client-side validation. To enhance security and ensure data integrity, server-side validation is essential.
4. Server-Side Validation with Node.js
To demonstrate server-side validation, let’s assume you’re using Node.js on the server side. First, you need to set up a server to handle form submissions and validation. Here’s a simplified example using the Express.js framework:
const express = require("express");
const bodyParser = require("body-parser");
const app = express();
app.use(bodyParser.urlencoded({ extended: false }));
app.post("/submit", (req, res) => {
const name = req.body.name;
const email = req.body.email;
if (!name || !email) {
res.status(400).json({ error: "Name and email are required fields" });
} else {
// Perform further processing or save data to a database.
res.status(200).json({ success: "Form submitted successfully" });
}
});
app.listen(3000, () => {
console.log("Server is running on port 3000");
});
In this Node.js example, we’re using the Express.js framework to create a server that listens for POST requests at the “/submit” route. We use the body-parser
middleware to parse form data sent in the request.
The server checks if the name
and email
fields are present in the request body and returns an error response if they are missing. Otherwise, it proceeds with further processing or data storage.
Conclusion
JavaScript form validation is an essential aspect of web development that ensures data accuracy, enhances security, improves user experience, and maintains data consistency. By implementing both client-side and server-side validation, you can create robust and secure web applications.
Remember that client-side validation provides immediate feedback to users but should not be relied upon solely for security. Always perform server-side validation to protect your application from malicious attacks and ensure the integrity of user-submitted data.
Form validation is just one piece of the puzzle when it comes to creating secure web applications. As you continue to develop your skills in web development, consider exploring additional security measures and best practices to build resilient and user-friendly web applications.
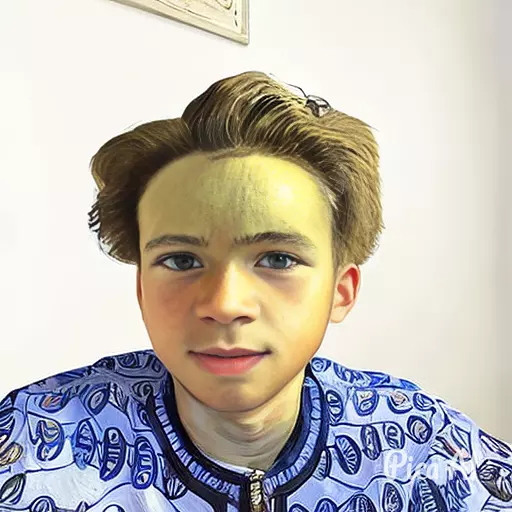