In JavaScript, you can create variables using three different keywords: var
, let
, and const
. The choice of which keyword to use depends on the variable’s intended scope and whether you want the variable to be mutable (changeable) or immutable (constant). Here’s how to create variables with each keyword:
- Using
var
(legacy, not recommended):Thevar
keyword is the oldest way to declare variables in JavaScript. It has function-level scope, which means variables declared withvar
are scoped to the nearest enclosing function or, if declared outside of any function, they are global.
var x = 10;
Variables declared with var
can be re-declared within the same scope, which can lead to unexpected behavior.
2. Using let
(block-scoped, mutable):The let
keyword was introduced in ECMAScript 6 (ES6) and is the preferred way to declare variables in modern JavaScript. It has block-level scope, which means variables declared with let
are scoped to the nearest enclosing block (e.g., a block inside a function, loop, or conditional statement).
Javascript Animation Libraries
let y = 20;
Variables declared with let
can be reassigned new values within the same scope.
3. Using const
(block-scoped, immutable):The const
keyword is used to declare constants in JavaScript. Variables declared with const
are also block-scoped like let
, but they cannot be reassigned once they are initialized.
const z = 30;
Attempting to reassign a const
variable will result in an error:
z = 40; // Error: Assignment to a constant variable
Choose the appropriate keyword (var
, let
, or const
) based on your specific use case and the desired behavior of the variable. In modern JavaScript, it’s generally recommended to use let
for mutable variables and const
for immutable values whenever possible to improve code clarity and prevent unintended changes.
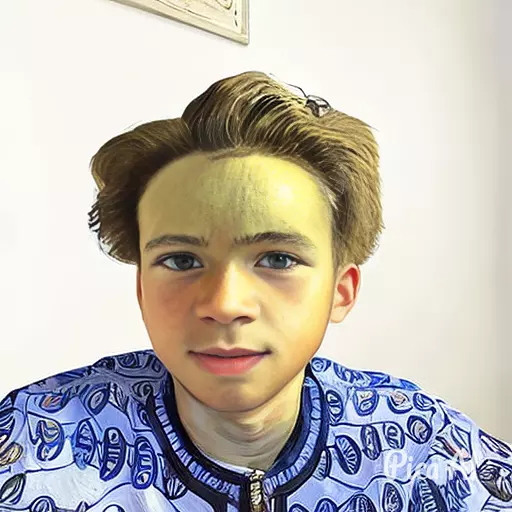