React, the JavaScript library for building user interfaces, offers developers two primary ways to create components: functional components and class components. These two approaches have been central to React’s evolution and have their own distinct advantages and use cases. In this article, we will delve into the core differences between functional and class components in React, highlighting their unique features, capabilities, and when to use each.
Functional Components: The Basics
Functional components, also known as stateless or functional stateless components, were introduced in React as a way to simplify the creation of reusable UI elements. At their core, functional components are JavaScript functions that take in props as arguments and return React elements, making them lightweight and easy to read. Let’s explore the key characteristics of functional components.
- Simplicity and Clarity:
- Functional components are minimalistic and focused on doing one thing well: rendering UI elements. This simplicity leads to cleaner and more readable code, making it easier for developers to understand and maintain.
- No Internal State:
- One of the most significant distinctions of functional components is that they do not have internal state. This means they are entirely dependent on the props provided to them and cannot manage their own state.
- Stateless:
- Functional components are inherently stateless, meaning they are incapable of maintaining any state information. This simplicity is beneficial for certain types of components, such as presentational components.
- Reusability:
- Functional components encourage reusability, as they are essentially pure functions. Developers can pass different props to the same functional component to render various instances of it throughout an application.
Class Components: The Foundation
Class components were the original way to create components in React and are sometimes referred to as “traditional” or “legacy” components. They provide more extensive capabilities compared to functional components, making them suitable for a broader range of use cases. Let’s delve into the defining characteristics of class components.
- Internal State Management:
- Class components are capable of managing their own internal state. This ability to hold and modify state within the component itself is particularly useful for handling complex interactions and data manipulation.
- Lifecycle Methods:
- Class components come with a range of lifecycle methods, such as componentDidMount, componentDidUpdate, and componentWillUnmount. These methods allow developers to control component behavior at various stages of its lifecycle.
- Component Hierarchy:
- Class components can have a more complex hierarchy with child components. This hierarchical structure can be useful for creating deeply nested UI structures and managing inter-component communication.
- Refs:
- Refs in React are a way to access the DOM directly, and they are primarily used with class components. Refs can be helpful when you need to interact with or manipulate a DOM element directly.
Differences Between Functional and Class Components
Now that we have a solid understanding of the fundamental characteristics of functional and class components, let’s explore the key differences between these two approaches.
- Functional components are defined as JavaScript functions. They take props as arguments and return JSX elements. Here’s an example:
const FunctionalComponent = (props) => {
return <div>{props.text}</div>;
};
- Class components, on the other hand, are ES6 classes that extend the React.Component class. They include a render method for rendering UI and can have a constructor for initializing state. Here’s an example:
class ClassComponent extends React.Component {
constructor(props) {
super(props);
this.state = { text: props.text };
}
render() {
return <div>{this.state.text}</div>;
}
}
- The syntax differences are evident, with functional components being more concise and class components involving more boilerplate.
- State Management:
- Functional components do not have internal state. They rely solely on the props passed to them. Any state required for rendering or functionality must be managed externally by higher-level components (usually through React hooks).
- Class components have the ability to manage their own state using this.state and setState. This internal state management can simplify certain aspects of component logic.
- Lifecycle Methods:
- Functional components do not have access to lifecycle methods. Instead, developers use React hooks like useEffect to achieve similar functionality. useEffect allows functional components to perform side effects and manage lifecycle-related tasks.
- Class components offer a range of lifecycle methods, giving developers more fine-grained control over component behavior at different stages of its lifecycle.
- Refs:
- Refs are primarily used with class components. They allow you to access and manipulate DOM elements directly. Functional components can use refs, but it’s a less common practice, and they often require the use of the useRef hook.
When to Use Functional Components
Functional components have gained popularity in recent years due to their simplicity and ease of use. They are well-suited for certain scenarios:
- Presentational Components:
- Functional components are ideal for creating presentational or dumb components that focus on rendering UI elements based on the provided props. These components don’t need to manage complex state or interactions.
- Reusability:
- If you need to create reusable UI components that accept different sets of props, functional components are an excellent choice. They promote a functional programming approach and make it easy to share components across your application.
- Functional Composition:
- Functional components work well when composing larger components from smaller ones. You can build complex UI structures by combining simple functional components.
- Performance Optimization:
- Functional components, when used with React hooks like useMemo and useCallback, can be optimized for performance. These hooks allow you to memoize values and functions, reducing unnecessary re-renders.
When to Use Class Components
While functional components are suitable for many scenarios, class components still have their place in React applications:
- Complex State Management:
- When dealing with complex state management requirements, such as forms with controlled inputs or intricate data manipulation, class components with internal state management can be beneficial.
- Lifecycle Control:
- Class components are necessary when fine-grained control over component lifecycles is required. This is often the case when dealing with animations, third-party libraries, or interactions that involve precise timing.
- Refs and Direct DOM Manipulation:
- If your application requires direct access to DOM elements or if you’re working with third-party libraries that rely on refs, class components are the better choice. They provide a straightforward way to manage refs.
- Legacy Codebase:
- In older React codebases, class components may still be prevalent. It can be more practical to continue using class components in these cases, especially if rewriting components would be time-consuming.
The Transition: From Class to Functional Components
With the introduction of React hooks, the line between functional and class components has blurred. Hooks, such as useState, useEffect, and useContext, allow functional components to manage state, perform side effects, and access context. This has led to a shift towards using functional components in most scenarios, even for components that previously required class components.
Here’s a brief overview of how some class component features can be achieved in functional components using hooks:
- State Management:
- useState hook allows functional components to manage state effectively. You can create and update component state within functional components.
- Lifecycle Methods:
- useEffect hook enables you to replicate the behavior of componentDidMount, componentDidUpdate, and componentWillUnmount. You can control side effects and component updates with useEffect.
- Refs:
- useRef hook provides a way to create and access refs in functional components, making it possible to perform direct DOM manipulation when needed.
- Context:
- useContext hook allows functional components to consume context values, simplifying global state management.
The transition from class to functional components is often driven by the desire for cleaner, more maintainable code. Newer React codebases tend to favor functional components and hooks due to their simplicity and conciseness.
Conclusion
In the world of React development, understanding the differences between functional and class components is crucial for making informed design decisions. Functional components, with their simplicity and ease of use, have become the default choice for many scenarios. They are particularly well-suited for creating presentational components, achieving reusability, and composing complex UIs.
Class components, with their internal state management, lifecycle methods, and refs, still have their place in more complex applications. They shine when precise control over component behavior and state is required.
Ultimately, the choice between functional and class components depends on the specific needs of your project, your team’s preferences, and the existing codebase. As React continues to evolve, it’s essential to stay up-to-date with best practices and embrace the flexibility that both functional and class components offer in building dynamic and interactive user interfaces.
React Router Navigation With Examples
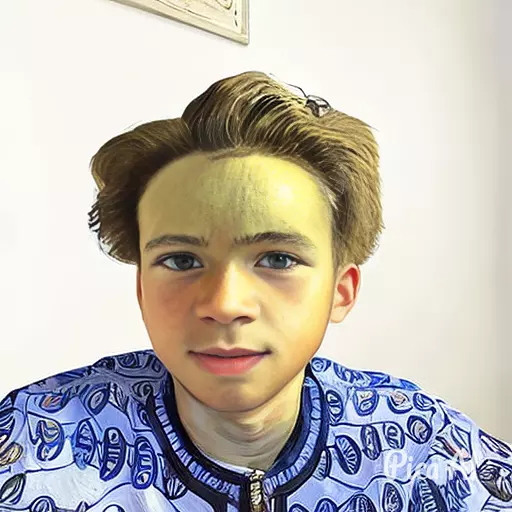