JavaScript, one of the most widely used programming languages in web development, has come a long way in terms of performance and efficiency. Part of this progress is attributed to a crucial behind-the-scenes process called “garbage collection.”
In this article, we’ll delve deep into the world of garbage collection in JavaScript, exploring what it is, how it works, its impact on performance, and best practices for developers to optimize memory management.
What is Garbage Collection?
Garbage collection is a memory management process that automatically identifies and reclaims memory that is no longer in use, making it available for new data and objects. In JavaScript, which is a dynamically typed language, memory management is handled by the browser’s JavaScript engine, allowing developers to focus on writing code without worrying about memory allocation and deallocation.
How Garbage Collection Works
JavaScript’s garbage collector works by employing various algorithms to track and identify memory that can be safely reclaimed. Let’s explore some key concepts and methods used in garbage collection:
- Reference Counting: One of the simplest garbage collection techniques involves counting references to objects. When an object’s reference count drops to zero, meaning there are no references to it, the memory it occupies can be safely released. However, this method is not foolproof, as it can’t detect circular references, where objects reference each other, keeping their reference count nonzero.
- Mark-and-Sweep Algorithm: Modern JavaScript engines, like V8 in Chrome or SpiderMonkey in Firefox, use more advanced techniques such as the Mark-and-Sweep algorithm. This algorithm involves two phases:
- Mark Phase: The garbage collector traverses the entire object graph starting from the global object (window in the case of a web browser) and marks objects that are still reachable.
- Sweep Phase: After marking, the garbage collector sweeps through memory and frees up memory occupied by objects that were not marked as reachable during the mark phase.
- Generational Garbage Collection: This technique takes advantage of the observation that most objects have a short lifetime. Objects are divided into generations based on their age, with new objects placed in the youngest generation. The garbage collector focuses its efforts on the younger generations, as they tend to contain more short-lived objects. This approach improves garbage collection efficiency.
Impact on Performance
Effective garbage collection is essential for maintaining a smooth and responsive web application. However, it’s important to note that poorly managed memory and inefficient garbage collection can lead to performance issues, such as:
- Memory Leaks: A memory leak occurs when objects that are no longer needed are not collected, leading to a gradual increase in memory usage over time. This can cause applications to slow down or crash.
- Jank: Jank refers to the stuttering or unresponsiveness of a web application. Frequent garbage collection pauses can result in jank, negatively impacting user experience.
- Increased CPU Usage: Inefficient garbage collection can consume a significant amount of CPU resources, making the application less energy-efficient and potentially draining device batteries faster.
Best Practices for Developers
To ensure efficient garbage collection and optimal memory management in JavaScript, developers can follow these best practices:
- Minimize Global Variables: Global variables persist for the entire lifetime of the application, making it harder for the garbage collector to free up memory. Minimize the use of global variables and opt for local variables whenever possible.
- Use Scope Wisely: Understand variable scope in JavaScript. Variables declared inside functions have limited scope and are easier for the garbage collector to manage. Avoid creating unnecessary closures that can retain references to objects longer than needed.
- Avoid Circular References: Be cautious when creating circular references between objects. If two or more objects reference each other, they won’t be collected even if they are no longer needed.
- Memory Profiling: Use browser developer tools to profile memory usage. This can help identify memory leaks and inefficient memory management in your application.
- Dispose of Event Listeners: Remove event listeners when they are no longer needed. Failing to do so can prevent the associated objects from being garbage collected.
- Optimize Loops: Be mindful of loops, especially in long-running processes. Avoid creating new objects unnecessarily inside loops, and use functional programming techniques like
map
andfilter
when appropriate. - Use Proper Data Structures: Choose the right data structures for your application. Arrays, maps, and sets can be more memory-efficient and performant than using plain objects for all purposes.
- Limit DOM Manipulations: Frequent and unnecessary changes to the DOM can increase memory usage and trigger more frequent garbage collection. Use techniques like DOM batching and virtual DOM libraries to optimize updates.
Conclusion
Garbage collection is a critical aspect of memory management in JavaScript, ensuring that web applications run smoothly and efficiently. Understanding how garbage collection works and following best practices for memory management can help developers create high-performing and responsive web applications. By being mindful of memory usage and optimizing code for efficient garbage collection, developers can harness the full potential of JavaScript for building modern web applications.
Understanding JavaScript Promises
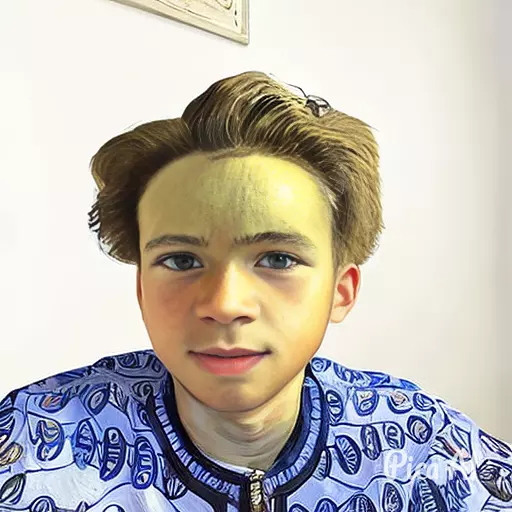