JavaScript, as a versatile and powerful programming language for software development, offers numerous features and techniques that can help software developers write efficient and maintainable code. One such technique is the use of self-executing functions, also known as immediately-invoked function expressions (IIFE).
In this article, we will explore what self-executing functions are, why they are useful, and how to leverage them effectively in your JavaScript projects.
Enroll for software development training in Abuja, Nigeria
What are Self-Executing Functions?
A self-executing function, or IIFE, is a JavaScript function that is defined and executed immediately after its creation. Unlike regular functions that require a call to execute their code, IIFE runs automatically. This feature is particularly handy when you want to encapsulate code and create a private scope for your variables, preventing them from polluting the global scope and is a good software development practice.
The basic syntax for an IIFE looks like this:
(function () {
// Your code here
})();
Here’s a breakdown of how this works:
- Enclose the function definition within parentheses
(function () { ... })
. - Immediately following the closing parentheses, add another pair of parentheses
( ... )
to invoke the function.
In this software development training in Abuja, Nigeria, you will learn how to build software solutions that solve real time problems.
Inside the first set of parentheses, you can pass parameters to the IIFE just like you would with any regular function. For example:
(function (x, y) {
console.log(x + y);
})(5, 10); // Outputs: 15
Benefits of Self-Executing Functions
Self-executing functions offer several advantages that make them a valuable tool for software developers who use JavaScript:
1. Encapsulation and Scope Isolation
One of the primary benefits of IIFE is that they create a private scope for your code. Any variables declared inside an IIFE are not accessible from the outside, preventing them from conflicting with variables in the global scope or other parts of your code. This helps improve code maintainability and reduces the risk of naming collisions.
(function () {
var privateVar = 'This is private';
console.log(privateVar); // Outputs: 'This is private'
})();
console.log(typeof privateVar); // Outputs: 'undefined'
2. Avoiding Global Pollution
In JavaScript, global variables can be a source of bugs and unintended side effects. Self-executing functions allow you to define and use variables without polluting the global scope. This is especially important when working on large projects with multiple developers to prevent naming conflicts and make code more predictable.
(function () {
var localVar = 'This is not global';
})();
console.log(typeof localVar); // Outputs: 'undefined'
3. Modular Code
IIFE can be used to create modular code by encapsulating related functionality within separate self-executing functions. This modular approach promotes code organization and reusability.
var moduleA = (function () {
var privateVar = 'Module A';
function privateFunction() {
console.log(privateVar);
}
return {
publicMethod: function () {
privateFunction();
}
};
})();
var moduleB = (function () {
var privateVar = 'Module B';
function privateFunction() {
console.log(privateVar);
}
return {
publicMethod: function () {
privateFunction();
}
};
})();
moduleA.publicMethod(); // Outputs: 'Module A'
moduleB.publicMethod(); // Outputs: 'Module B'
4. Preventing Variable Hoisting
JavaScript has a feature called “variable hoisting,” which means that variable declarations are moved to the top of their containing scope during runtime. IIFE can help avoid unexpected behavior caused by variable hoisting by providing a clearly defined scope for your variables.
(function () {
console.log(myVar); // Outputs: 'undefined'
var myVar = 'I am hoisted';
})();
5. Initialization Code
IIFE is useful for executing code that needs to run immediately when a script is loaded. For example, you can use them to set up configuration settings, initialize modules, or establish event listeners.
(function () {
// Initialize some configuration settings
var config = {
apiUrl: 'https://example.com/api',
apiKey: 'your-api-key'
};
// Set up event listeners
document.addEventListener('DOMContentLoaded', function () {
// Your initialization code here
});
})();
Common Use Cases
Self-executing functions are employed in various scenarios to improve code structure and maintainability:
1. Avoiding Global Variables
One of the most common use cases for IIFE is to avoid creating global variables. By encapsulating code within an IIFE, you can ensure that variables are limited to the scope of the function, preventing them from leaking into the global scope.
(function () {
var counter = 0;
function incrementCounter() {
counter++;
console.log('Counter:', counter);
}
// Attach an event listener
document.getElementById('increment-button').addEventListener('click', incrementCounter);
})();
2. Creating Modules
IIFE is frequently used to create modular code. By returning an object or function containing the module’s public API, you can expose specific functionality while keeping the module’s internal details hidden.
var myModule = (function () {
var privateVar = 'This is private';
function privateFunction() {
console.log(privateVar);
}
return {
publicFunction: function () {
privateFunction();
}
};
})();
3. Avoiding Conflicts in Libraries
Library authors often use IIFE to prevent variable and function name conflicts between their library and other JavaScript code on a page. This practice is particularly important when creating libraries that will be used in various contexts.
var myLibrary = (function () {
// Library code here
return {
// Public API here
};
})();
Best Practices and Considerations
While self-executing functions offer many benefits, it’s essential to follow best practices and consider potential caveats:
1. Maintainability
While IIFE can enhance code maintainability, overusing them can lead to complex and hard-to-follow code. Use them judiciously, primarily for encapsulating specific functionality or avoiding global variables.
2. Minification
When you minify your JavaScript code for production, IIFE can be automatically transformed to save space. This means that you don’t need to worry about the extra function names or parentheses in your minified code.
3. Use ES6 Modules
In modern JavaScript development, ES6 modules provide a more structured and standard way to create modular code. Consider using ES6 modules when working on projects that support them.
4. Debugging
IIFE can make debugging more challenging since the code is encapsulated in a private scope. Use browser developer tools effectively and consider logging values to the console within your IIFE to aid in debugging.
Conclusion
Self-executing functions, or IIFE, are a powerful and flexible technique in JavaScript for encapsulating code, avoiding global scope pollution, and creating modular code. They offer a range of benefits, from improved maintainability to preventing variable hoisting and naming conflicts. By understanding when and how to use IIFE effectively, you as a software developer can write cleaner, more organized JavaScript code that is easier to manage and maintain.
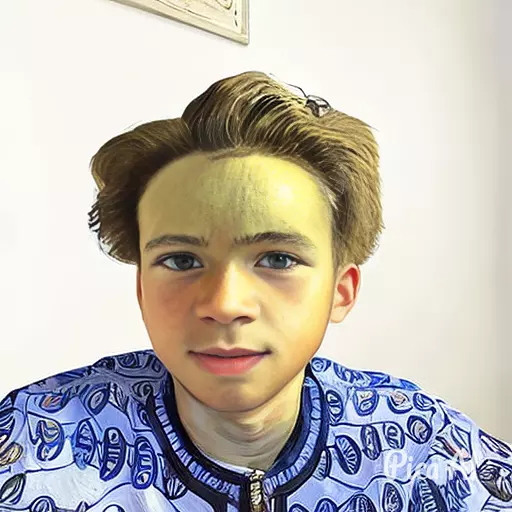