With PHP we can upload various files to the server depending on the given requirements and specifications. In this tutorial, we shall look at how to upload image to the server and store the path in our database.
So let’s begin!
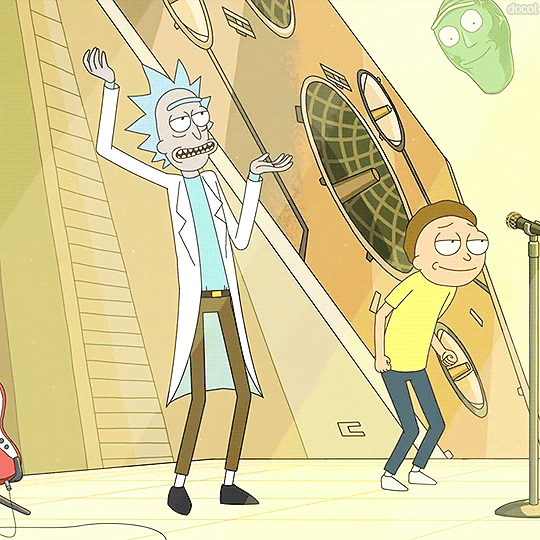
First, let us create our database connection:
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
$host = 'localhost';
$user = 'root';
$password = '';
$dbname = 'test';
$dsn = '';
try{
$dsn = 'mysql:host='.$host. ';dbname='.$dbname;
$pdo = new PDO($dsn, $user, $password); $pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
}catch(PDOException $e){
echo 'connection failed: '.$e->getMessage();
}
?>
Next, we create our table called images. Here we’re adding both the image name and the image file.
CREATE TABLE `images` (
`id` int(11) NOT NULL,
`image_name` varchar(300) NOT NULL,
`image_file` text NOT NULL,
`date_time` datetime NOT NULL DEFAULT current_timestamp()
) ENGINE=MyISAM DEFAULT CHARSET=latin1;
Next, we create a form that with which we can upload our file.
<form action="index.php" method="post" enctype="multipart/form-data">
<div>
<label for="title">Image title</label>
<input id="title" name="image_name" required="required" type="text" aria-required="true" aria-invalid="false">
</div>
<div class="form-group">
<div id="preview-container-imageThre">
<button required type="button" id="upload-dialog-three">Choose Image</button><br /><br />
<input require type="file" id="file" name="file" accept="image/jpg,image/png" style="display:none" required="required"/>
<img id="preview-image-three" style="display:none" />
</div>
</div>
<div>
<button id="payment-button" type="submit" name="sub">
Add
</button>
</div>
</form>
Next, we will write our Javascript code that will preview our image using jQuery. Don’t forget to add your jQuery cdn dependency.
Create Login and registration form using PDO
Add Upload Preview Using jQuery
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
let _PREVIEW_URL;
/* Show Select File dialog */
document.querySelector("#upload-dialog-three").addEventListener('click', function() {
document.querySelector("#file").click();
});
/* Selected File has changed */
document.querySelector("#file").addEventListener('change', function() {
// user selected file
var file = this.files[0];
// allowed MIME types
var mime_types = [ 'image/jpeg', 'image/png' ];
// validate MIME
if(mime_types.indexOf(file.type) == -1) {
alert('Error : Incorrect file type');
return;
}
// validate file size
if(file.size > 5*1024*1024) {
alert('Error : Exceeded size 2MB');
return;
}
// validation is successful
// replace upload dialog button text
document.querySelector("#upload-dialog-three").textContent = 'Replace image';
// object url
_PREVIEW_URL = URL.createObjectURL(file);
// set src of image and show
document.querySelector("#preview-image-three").setAttribute('src', _PREVIEW_URL);
document.querySelector("#preview-image-three").style.display = 'inline-block';
});
</script>
<?php
define('BASEPATH', true);
require './database.php';
$exists = "";
if(isset($_POST['sub'])){
try {
$dsn = new PDO("mysql:host=$host;dbname=$dbname", $user, $password);
$dsn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
$title = $_POST['image_name'];
$target_file = "";
$target_dir = "./images/"; //set your folder path
$target_file = $target_dir . basename($_FILES["file"]["name"]);
$uploadOk = 1;
$imageFileType = strtolower(pathinfo($target_file,PATHINFO_EXTENSION));
move_uploaded_file($_FILES["file"]["tmp_name"], $target_file) ;
$target_file = 'https://yourdomain.com/'.$target_file;
$sql = "SELECT COUNT(image_file) AS num FROM images WHERE image_file = :image_file"; //check if file exists
$stmt = $pdo->prepare($sql);
$stmt->bindValue(':image_file', $target_file);
$stmt->execute();
$row = $stmt->fetch(PDO::FETCH_ASSOC);
if($row['num'] > 0 ){
//Show Bootstrap alert. Optional here
$exists = '<div class="col-sm-6 alert alert-danger alert-dismissible fade show" role="alert" style="margin: 0 auto">
<p><span class="fa fa-exclamation-triangle"></span> This image already exists.</p>
<span><span class="fa fa-lightbulb-o fa-warning"></span> Hint: Rename image</span>
</div>';
}else{
$stmt = $dsn->prepare("INSERT INTO images (image_name, image_file)
VALUES (:image_name, :image_file)");
$stmt->bindParam(':image_name', $title);
$stmt->bindParam(':image_file', $target_file);
if($stmt->execute()){
echo '<script>alert("New image added the Gallery successfully.")</script>';
}else{
echo '<script>alert("An error occurred")</script>';
}
}
}catch(PDOException $e){
$error = "Error: " . $e->getMessage();
echo '<script type="text/javascript">alert("'.$error.'");</script>';
}
}
?>
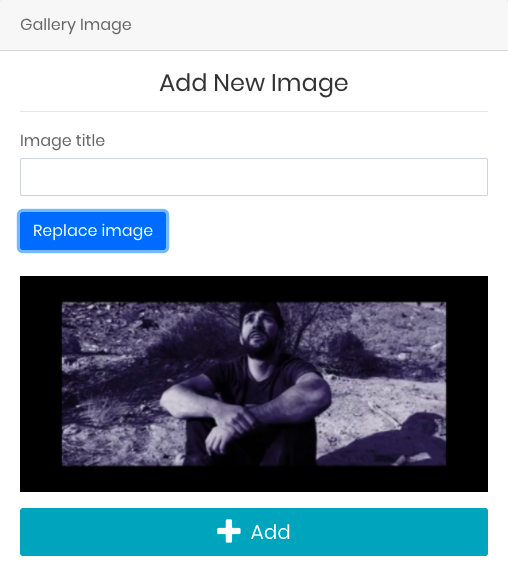
Congratulations!
You have successfully uploaded your image to the server.
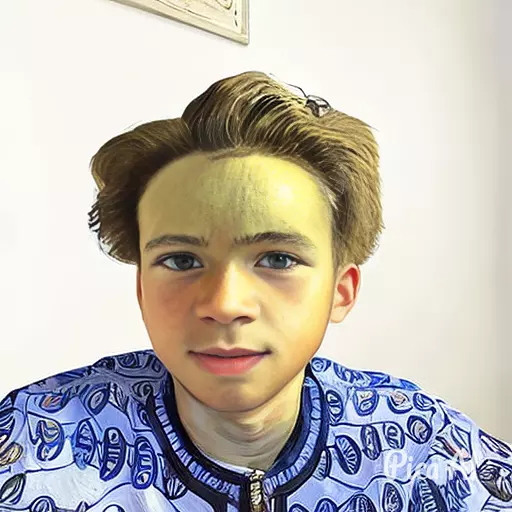