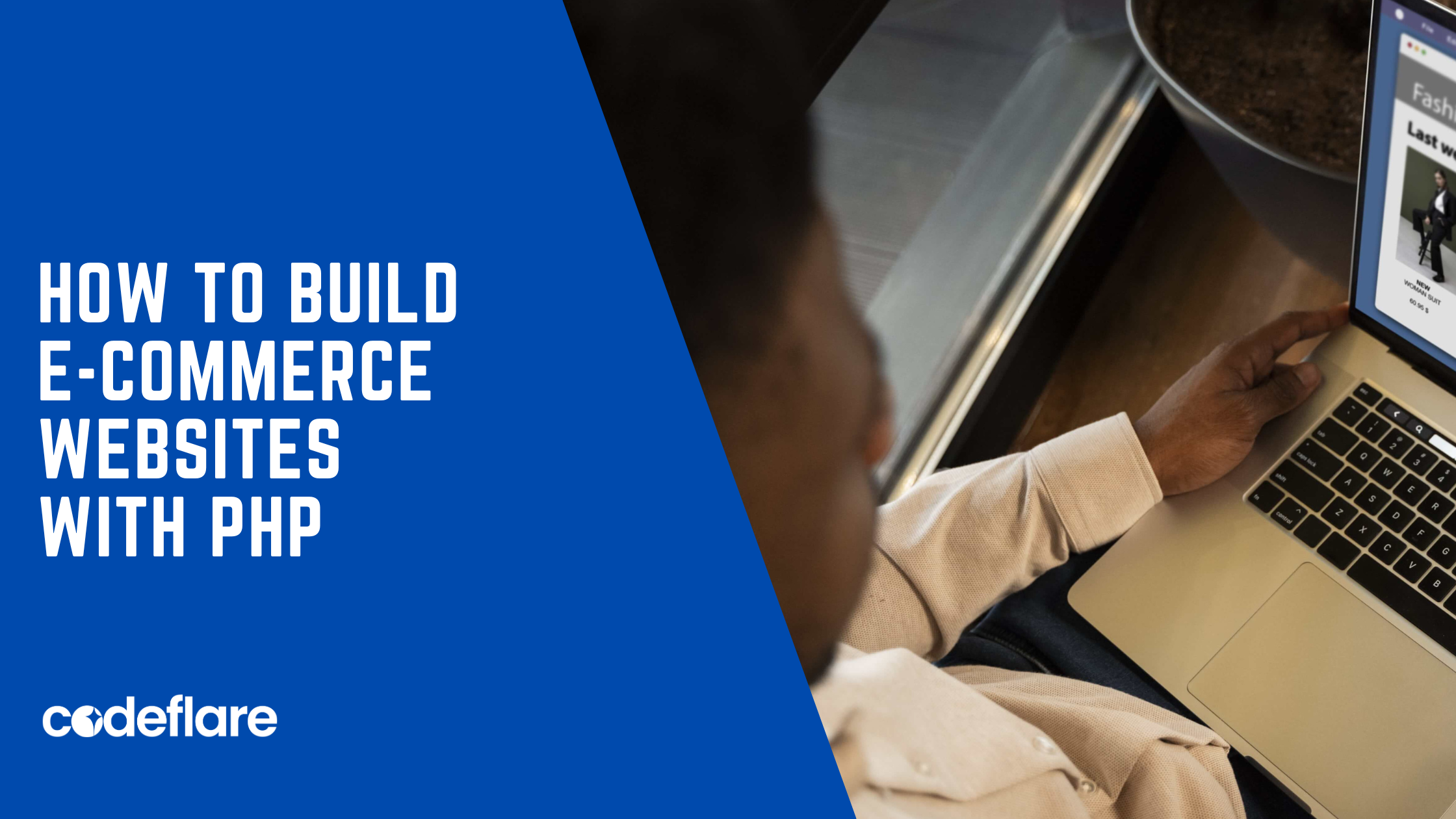
In today’s digital age, the e-commerce industry flourishes as businesses and consumers increasingly favor online platforms for shopping. Creating e-commerce websites with PHP demands a strong framework capable of managing products, shopping carts, payment integration, and other functionalities. In this guide, we will explore renowned platforms such as WooCommerce and Magento, focusing on building e-commerce websites with PHP. Additionally, we will delve into key aspects like product management, shopping cart functionality, and payment integration.
Best school to learn php development
1. Choosing the Right Platform: When embarking on an e-commerce project, selecting the appropriate platform is crucial. Platforms like WooCommerce and Magento offer powerful features tailored for e-commerce needs. WooCommerce, being a WordPress plugin, provides seamless integration with WordPress websites, making it an excellent choice for small to medium-sized businesses. On the other hand, Magento offers scalability and advanced customization options, making it suitable for large-scale enterprises.
2. Setting Up Product Management: Effective product management is at the core of any successful e-commerce website. With PHP, developers can implement robust product management systems that allow for easy addition, editing, and categorization of products. Using PHP frameworks like Laravel or CodeIgniter, developers can create admin panels where store owners can manage inventory, update product descriptions, and upload images effortlessly.
Code Example – Product Management in Laravel:
// Controller method for adding a new product
public function addProduct(Request $request)
{
$product = new Product();
$product->name = $request->input('name');
$product->price = $request->input('price');
$product->save();
return redirect()->route('products.index')->with('success', 'Product added successfully.');
}
3. Implementing Shopping Cart Functionality: The shopping cart is a critical component of an e-commerce website, allowing users to add products, review their selections, and proceed to checkout. Additionally, PHP frameworks offer libraries and tools to implement shopping cart functionality seamlessly. Furthermore, developers can store cart data in sessions or databases, calculate totals, apply discounts, and handle various checkout scenarios.
Code Example – Shopping Cart in PHP Session:
// Adding a product to the cart
if(isset($_POST['add_to_cart'])) {
$product_id = $_POST['product_id'];
$quantity = $_POST['quantity'];
$_SESSION['cart'][$product_id] = $quantity;
}
4. Integrating Secure Payment Gateways: Payment integration is a pivotal aspect of e-commerce development. PHP supports integration with leading payment gateways like PayPal, Stripe, and Square. Developers can use APIs provided by these gateways to securely process payments, handle refunds, and manage recurring billing. Implementing SSL certificates and adhering to PCI DSS standards ensures the security of sensitive customer information during transactions.
Code Example – PayPal Integration in PHP:
// PayPal payment processing
$paypal = new PayPal();
$payment = $paypal->processPayment($totalAmount);
if($payment->isSuccessful()) {
// Payment successful, update order status
} else {
// Payment failed, handle error
}
5. Enhancing User Experience with Responsive Design: A crucial element of modern e-commerce websites is responsive design. Additionally, using PHP alongside HTML, CSS, and JavaScript frameworks like Bootstrap or Foundation, developers can craft responsive and mobile-friendly interfaces. Responsive design guarantees seamless adaptation to diverse devices, providing users with optimal browsing and shopping experiences across desktops, tablets, and smartphones.
Code Example – Responsive Design with Bootstrap:
<!-- Bootstrap responsive navigation bar -->
<nav class="navbar navbar-expand-lg navbar-light bg-light">
<a class="navbar-brand" href="#">E-commerce Website</a>
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarSupportedContent" aria-controls="navbarSupportedContent" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarSupportedContent">
<ul class="navbar-nav mr-auto">
<li class="nav-item active">
<a class="nav-link" href="#">Home <span class="sr-only">(current)</span></a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Products</a>
</li>
<!-- More navigation links -->
</ul>
</div>
</nav>
6. Optimizing Performance and Scalability: Performance optimization is essential for e-commerce websites to deliver fast loading times and smooth navigation. PHP developers can optimize code, leverage caching mechanisms, and implement content delivery networks (CDNs) to enhance website performance. Scalability is also key, especially for growing businesses. PHP frameworks offer scalability options to handle increased traffic, transactions, and data volume effectively.
In conclusion: Building e-commerce websites with PHP offers numerous opportunities for businesses aiming to enhance their online presence. Additionally, by utilizing PHP frameworks, developers can craft websites with advanced features such as streamlined product management, seamless shopping cart functionality, secure payment integration, responsive design, and optimized performance. Furthermore, whether opting for WooCommerce, Magento, or tailored solutions, PHP remains a flexible and dependable choice for e-commerce development.