In JavaScript, an array is a data structure that allows you to store multiple values in a single variable. It is a container that holds an ordered collection of elements. These elements can be of any data type, such as numbers, strings, objects, or even other arrays.
Arrays in JavaScript are declared using square brackets ([]). Here’s an example of creating an array with three elements:
let myArray = [1, 2, 3];
In this example, myArray
is an array that contains three elements: 1, 2, and 3.
Arrays in JavaScript are zero-indexed, which means the first element is accessed using the index 0, the second element with index 1, and so on. You can access or modify the elements of an array using their respective indexes. Here’s an example:
let myArray = [1, 2, 3];
console.log(myArray[0]); // Output: 1
myArray[1] = 5;
console.log(myArray); // Output: [1, 5, 3]
In the first console.log
statement, myArray[0]
accesses the first element of the array, which is 1. In the second statement, myArray[1]
modifies the second element to the value 5. The resulting array is [1, 5, 3].
Merging Two Arrays
To merge two arrays in JavaScript, you can use various approaches depending on your requirements. Here are a few common methods to merge arrays:
- The Spread operator: The spread operator (
...
) is a feature introduced in JavaScript ES6 (ECMAScript 2015) that allows you to expand elements of an iterable (like an array or string) or object properties into another array, object, or function call.
let array1 = [1, 2, 3];
let array2 = [4, 5, 6];
let mergedArray = [...array1, ...array2];
console.log(mergedArray); // Output: [1, 2, 3, 4, 5, 6]
2. Concatenation using the concat()
method: The concat()
method in JavaScript is used to merge two or more arrays and returns a new array containing the combined elements. It does not modify the original arrays; instead, it creates a new array.
let array1 = [1, 2, 3];
let array2 = [4, 5, 6];
const mergeResult = array1.concat(array2);
console.log(array1); // Output: [1, 2, 3, 4, 5, 6]
3. push()
method: The push()
method in JavaScript is used to add one or more elements to the end of an array. It modifies the original array and returns the new length of the array.
let array1 = [1, 2, 3];
let array2 = [4, 5, 6];
Array.prototype.push.apply(array1, array2);
console.log(array1); // Output: [1, 2, 3, 4, 5, 6]
Summary
These methods create a new array that contains elements from both arrays. Note that the original arrays remain unchanged, and the merged array is assigned to a new variable (mergedArray
in the examples above). You can also choose to modify one of the existing arrays if desired and you can always choose a method that best fits your coding style.
Conditional Rendering in React JS
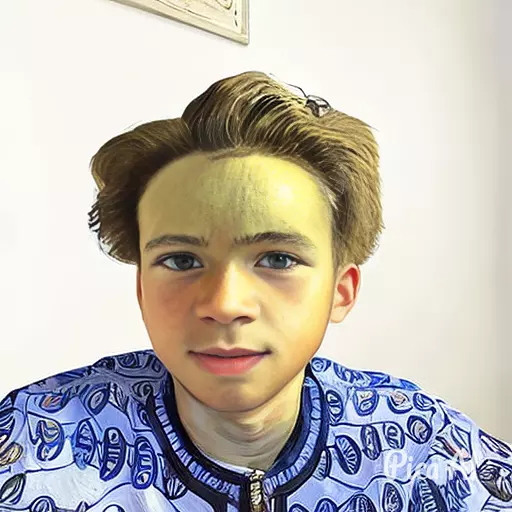