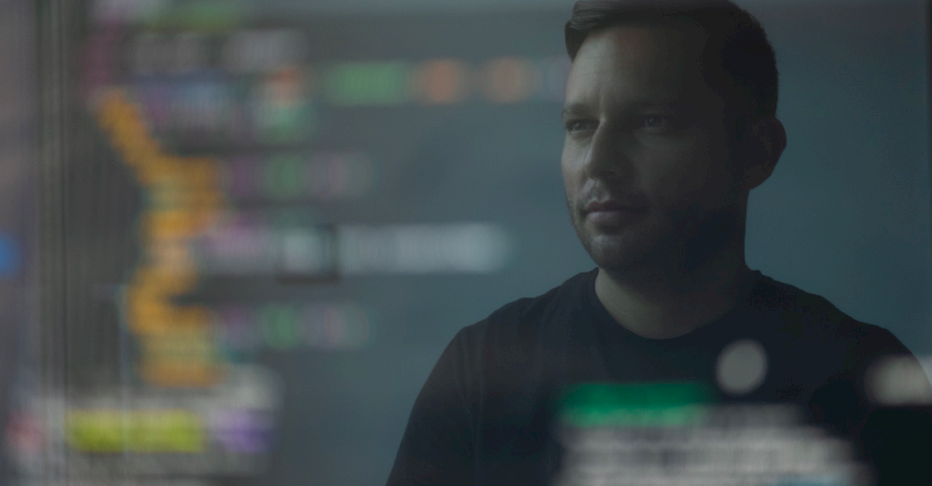
Conditional rendering is a crucial concept in React, a popular JavaScript library for building user interfaces. It allows you to control the display of components based on certain conditions, enabling dynamic and interactive web applications.
For instance, if you’re building an application that requires admin privileges to perform some task, instead having different pages for the different administrators, you can choose to conditionally render action buttons based on privilege the admin user has. If the current user does not have delete privilege, then he will not see the delete action button and so on and so forth.
In this article, we will delve into the world of conditional rendering in React, exploring its fundamental principles, best practices, and various techniques to handle conditional logic effectively. Whether you’re a beginner or an experienced React developer, this guide will equip you with the knowledge and skills to harness the power of conditional rendering and build highly responsive user interfaces.
First Understand the Concept of Conditional Rendering
In React, conditional rendering refers to the process of deciding whether to render a particular component or its contents based on certain conditions. This approach allows developers to create UI components that adapt to changing data or user interactions. By employing conditional rendering, you can control the visibility, behavior, and appearance of different elements in your application, leading to a more intuitive and personalized user experience, like in the scenario I pointed out earlier.
Conditional rendering in React typically revolves around conditional statements, such as if
, else
, and ternary operators
, that evaluate a condition and determine which components or elements should be rendered. React’s declarative nature enables developers to express these conditions seamlessly, making code more readable and maintainable.
Conditional Rendering Techniques
React provides multiple techniques for conditional rendering, catering to different use cases and preferences. Let’s explore some of the most commonly used techniques:
- If/Else Statements: You can use JavaScript’s
if
andelse
statements to conditionally render components. By evaluating a condition, you can render different components based on the result. - Ternary Operator: The ternary operator (
condition ? true : false
) is a concise way to perform conditional rendering. It allows you to render one component or another based on the condition. - && Operator: React supports using the logical AND operator (
&&
) to conditionally render a component. If the condition evaluates totrue
, the component is rendered; otherwise, it’s skipped. - Switch Statements: For more complex conditions, you can employ JavaScript’s
switch
statement to conditionally render components. By associating each case with a specific component, you can render different components based on the condition’s value.
Conditional Rendering Using If/else Statements
Let’s see an example of the most basic form of conditional rendering using if/else statements. Consider a scenario where we want to display a greeting message based on the time of day. Here’s how you can achieve this with conditional rendering:
import React, {Component} from 'react';
class Greeting extends Component {
const currentTime = new Date();
const hour = currentTime.getHours();
let message;
if (hour < 12) {
message = 'Good morning!';
} else if (hour < 18) {
message = 'Good afternoon!';
} else {
message = 'Good evening!';
}
render(){
return <div>{message}</div>;
}
}
export default Greeting;
In this example, we obtain the current hour using the getHours()
method and assign the appropriate message to the message
variable based on the hour’s value. The message is then rendered inside a <div>
element.
Conditional Rendering Using Ternary Operators
The ternary operator provides a concise way to perform conditional rendering in React. Let’s extend the previous example using the ternary operator:
import React, {Component} from 'react';
class Greeting extends Component {
const currentTime = new Date();
const hour = currentTime.getHours();
const message = hour < 12 ? 'Good morning!' : hour < 18 ? 'Good afternoon!' : 'Good evening!';
render(){
return <div>{message}</div>;
}
}
export default Greeting;
In this updated version, we utilize the ternary operator to evaluate the condition and assign the appropriate message to the message
variable in a single line of code. This approach offers a more concise and readable alternative to if/else statements for simple conditions.
Conditional Rendering with Logical AND Operator
The logical AND operator (&&
) can also be used for conditional rendering in React. Let’s consider a scenario where we want to render a button only if a user is logged in:
import React, {Component} from 'react';
const isLoggedIn = true;
class App extends Component {
render(){
return (
<div>
<h1>Welcome to the App!</h1>
{isLoggedIn && <button>Logout</button>}
</div>
);
}
}
export default App;
Conditional Rendering with Component Composition
React’s component composition allows us to create more modular and reusable code. Let’s consider the admin privilege example where we want to render a specific component based on a user’s role.
import React, {Component} from 'react';
class Dashboard extends Component {
constructor(props){
super(props);
this.state = {
useHasAccess: "admin"
}
}
UserDashboard() {
return <div>Welcome, User!</div>;
}
AdminDashboard() {
return <div>Welcome, Admin!</div>;
}
render(){
const { userRole } = this.state;
return (
<div>
<h1>Dashboard</h1>
{userRole === 'admin' ? <AdminDashboard /> : <UserDashboard />}
</div>
);
}
}
export default Dashboard;
In this example, we define two separate functional components: AdminDashboard
and UserDashboard
. The Dashboard
component receives the userRole
as a prop and renders the appropriate dashboard component based on the value of userRole
. This pattern allows for flexible and reusable code by composing different components based on conditions.
Conclusion
Conditional rendering is a powerful feature in React that allows you to create dynamic and interactive user interfaces. By mastering the various techniques and best practices outlined in this guide, you can effectively handle conditional logic, resulting in more flexible, responsive, and maintainable code. As you continue to explore React and its ecosystem, keep experimenting with conditional rendering to enhance the user experience and build exceptional web applications.