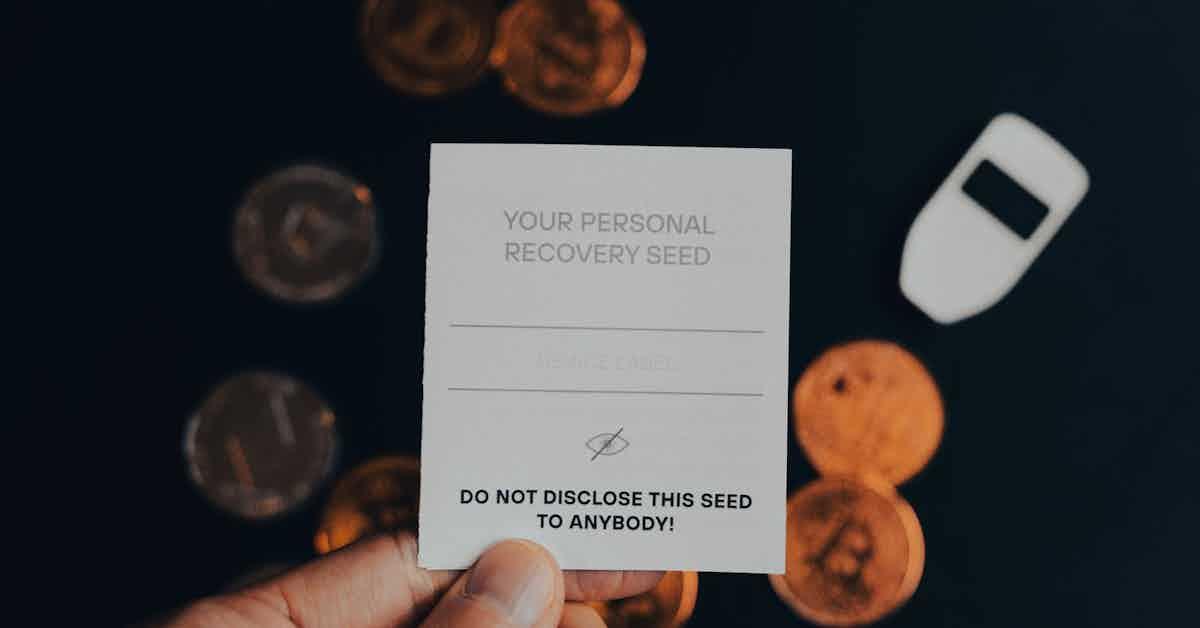
Toggle password visibility in React can be done easily with the following steps.
Toggle password visibility is a feature commonly found in password input fields on websites or applications. It allows users to switch between displaying the characters they type in a password field as plain text or as masked characters (usually dots or asterisks). The purpose of this feature is to provide users with the option to view their entered password temporarily, enabling them to verify its accuracy or to make any necessary corrections.
By clicking or tapping on an icon or checkbox labeled “Toggle Password Visibility” or an eye symbol, the user can switch between the visible and masked modes. When the visibility is toggled on, the characters they type will be shown as plain text, allowing them to see exactly what they are entering. Conversely, when the visibility is toggled off, the characters are hidden behind masked characters, which are commonly used to protect sensitive information from prying eyes.
This feature is particularly useful when entering passwords on devices or in environments where privacy is not a concern. It helps users ensure that they have entered their passwords correctly, reducing the likelihood of errors during the authentication process.
In this article, we are going to see how we can implement the toggle password visibility in React.
First, we will create a new React project with just a basic setup: an input field and a checkbox. We will also use Bootstrap for easy styling.
See how to validate a checkbox in React
npx create-react-app my-web-project
Next, we will add our JSX code as seen below.
import React, { PureComponent } from 'react';
import './App.css';
class App extends PureComponent {
render(){
return(
<div className="container">
<form>
<div className="mb-3">
<label for="exampleInputPassword1" className="form-label">Password</label>
<input type="password" className="form-control" id="exampleInputPassword1" />
</div>
<div className="mb-3 form-check">
<input type="checkbox" className="form-check-input" id="exampleCheck1" />
<label class="form-check-label" for="exampleCheck1">Check me out</label>
</div>
<button type="submit" className="btn btn-primary">Submit</button>
</form>
</div>
)
}
}
export default App;
Next, we will create a state variable called “showPassword” and set its initial value false.
constructor(props){
super(props);
this.state = {
showPassword: false,
}
}
Next, we will create a function called “togglePassword” as follows:
togglePassword = (e) => {
this.setState({ showPassword: e.target.checked});
}
Don’t forget that we need to bind this function to our state. We will do that as follows:
constructor(props){
super(props);
this.state = {
showPassword: false,
}
this.togglePassword = this.togglePassword.bind(this);
}
Next, we will call this function in our JSX as follows:
<div className="mb-3 form-check">
<input type="checkbox" onChange={this.togglePassword} className="form-check-input" id="exampleCheck1" />
<label class="form-check-label" for="exampleCheck1">{showPassword ? 'Hide password' : 'Show password'}</label>
</div>
What we are really doing here is to check if the checkbox has been clicked. If it has been clicked that means it’s true, so we update our state variable to true and set the text to ‘Hide password’ and vice versa.
Finally, we need to use a ternary operator to update the type attribute for our password input. If the “showPassword” value is true, we set the type to text else we set it to password.
<input type={showPassword ? 'text' : 'password'} className="form-control" id="exampleInputPassword1" />
Full Working Code
import React, { PureComponent } from 'react';
import './App.css';
class App extends PureComponent {
constructor(props){
super(props);
this.state = {
showPassword: false,
}
this.togglePassword = this.togglePassword.bind(this);
}
togglePassword = (e) => {
this.setState({ showPassword: e.target.checked});
}
render(){
const { showPassword } = this.state
return(
<div className="container">
<form>
<div className="mb-3">
<label for="exampleInputPassword1" className="form-label">Password</label>
<input type={showPassword ? 'text' : 'password'} className="form-control" id="exampleInputPassword1" />
</div>
<div className="mb-3 form-check">
<input type="checkbox" onChange={this.togglePassword} className="form-check-input" id="exampleCheck1" />
<label class="form-check-label" for="exampleCheck1">{showPassword ? 'Hide password' : 'Show password'}</label>
</div>
<button type="submit" className="btn btn-primary">Submit</button>
</form>
</div>
)
}
}
export default App;