PDO is an acronym for PHP Data Objects and is a useful alternative to MYSQLI. PHP introduced PHP Data Objects (PDO) in PHP 5.1 to help standardize and smoothen the development process.
PDO comprises the use of objects and abstraction layers to setup PDO database connections and queries. PDO is a database access layer provides a fast and consistent interface for accessing and managing databases in PHP applications.
So let us create a simple Login and Register form using this PDO principle.
Step 1: Database setup
First, we need to setup a script that will handle our database connections. Let us create a file called db.php and add the following code:
<?php
defined('BASEPATH') OR exit('No direct script access allowed'); //prevent direct script access
$host = 'localhost';
$user = 'root'; //replace with your database username
$password = ''; //replace with your database password
$dbname = 'test'; //replace with your database name
$dsn = '';
try{
$dsn = 'mysql:host='.$host. ';dbname='.$dbname;
$pdo = new PDO($dsn, $user, $password);
$pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
}catch(PDOException $e){
echo 'connection failed: '.$e->getMessage();
}
?>
Step 2: Setup Table
Next, we need to create table as follows:
CREATE TABLE `admin` (
`id` int(11) NOT NULL,
`username` varchar(100) NOT NULL,
`email` varchar(100) NOT NULL,
`password` text NOT NULL,
`date_time` datetime NOT NULL DEFAULT current_timestamp()
) ENGINE=MyISAM DEFAULT CHARSET=latin1;

Step 3: Create Register page
Since this tutorial is not about design and styling, we are just going to create a basic html form.
Let us call that file register.php and put the following contents:
<form action="register.php" method="post">
<input type="text" required="required" name="username" placeholder="Username">
<input required="required" type="email" name="email" placeholder="Email">
<input required="required" type="password" name="password" placeholder="Password">
<button name="submit" type="submit">register</button>
</form>
Next, we will write the PHP logic to handle the registration as seen below:
<?php
define('BASEPATH', true); //access connection script if you omit this line file will be blank
require 'db.php'; //require connection script
if(isset($_POST['submit'])){
try {
$dsn = new PDO("mysql:host=$host;dbname=$dbname", $user, $password);
$dsn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
$user = $_POST['username'];
$email = $_POST['email'];
$pass = $_POST['password'];
$pass = password_hash($pass, PASSWORD_BCRYPT, array("cost" => 12));
//Check if username exists
$sql = "SELECT COUNT(username) AS num FROM admin WHERE username = :username";
$stmt = $pdo->prepare($sql);
$stmt->bindValue(':username', $user);
$stmt->execute();
$row = $stmt->fetch(PDO::FETCH_ASSOC);
if($row['num'] > 0){
echo '<script>alert("Username already exists")</script>';
}
else{
$stmt = $dsn->prepare("INSERT INTO admin (username, email, password)
VALUES (:username,:email, :password)");
$stmt->bindParam(':username', $user);
$stmt->bindParam(':email', $email);
$stmt->bindParam(':password', $pass);
if($stmt->execute()){
echo '<script>alert("New account created.")</script>';
//redirect to another page
echo '<script>window.location.replace("index.php")</script>';
}else{
echo '<script>alert("An error occurred")</script>';
}
}
}catch(PDOException $e){
$error = "Error: " . $e->getMessage();
echo '<script type="text/javascript">alert("'.$error.'");</script>';
}
}
?>
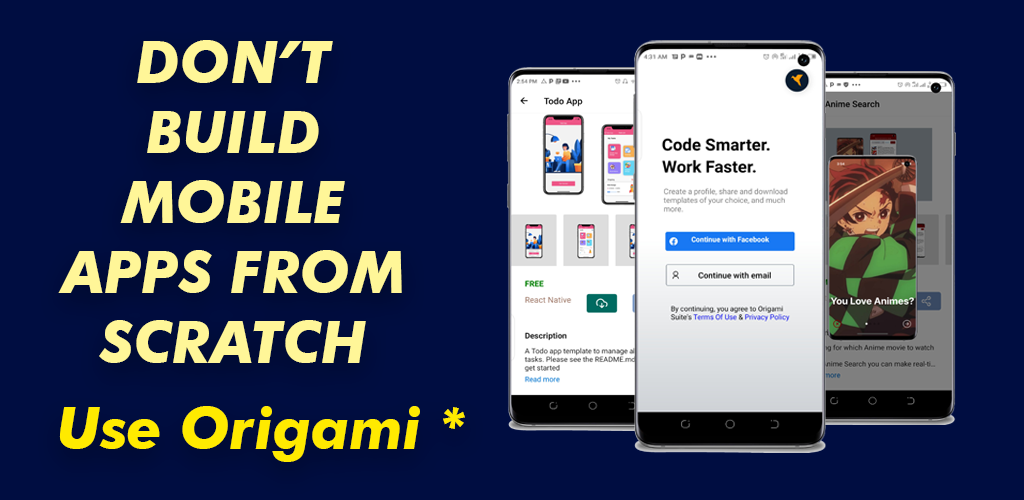
<?php
define('BASEPATH', true); //access connection script if you omit this line file will be blank
require 'db.php'; //require connection script
if(isset($_POST['submit'])){
try {
$dsn = new PDO("mysql:host=$host;dbname=$dbname", $user, $password);
$dsn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
$user = $_POST['username'];
$email = $_POST['email'];
$pass = $_POST['password'];
//encrypt password
$pass = password_hash($pass, PASSWORD_BCRYPT, array("cost" => 12));
//Check if username exists
$sql = "SELECT COUNT(username) AS num FROM admin WHERE username = :username";
$stmt = $pdo->prepare($sql);
$stmt->bindValue(':username', $user);
$stmt->execute();
$row = $stmt->fetch(PDO::FETCH_ASSOC);
if($row['num'] > 0){
echo '<script>alert("Username already exists")</script>';
}
else{
$stmt = $dsn->prepare("INSERT INTO admin (username, email, password)
VALUES (:username,:email, :password)");
$stmt->bindParam(':username', $user);
$stmt->bindParam(':email', $email);
$stmt->bindParam(':password', $pass);
if($stmt->execute()){
echo '<script>alert("New account created.")</script>';
//redirect to another page
echo '<script>window.location.replace("index.php")</script>';
}else{
echo '<script>alert("An error occurred")</script>';
}
}
}catch(PDOException $e){
$error = "Error: " . $e->getMessage();
echo '<script type="text/javascript">alert("'.$error.'");</script>';
}
}
?>
<form action="register.php" method="post">
<input type="text" required="required" name="username" placeholder="Username">
<input required="required" type="email" name="email" placeholder="Email">
<input required="required" type="password" name="password" placeholder="Password">
<button name="submit" type="submit">register</button>
</form>
Step 4: Create Login page
Let us also create a form for the login page as follows:
<form action="login.php" method="post">
<input type="text" name="username" placeholder="Username">
<input type="password" name="password" placeholder="Password">
<button name="submit" type="submit">sign in</button>
</form>
Next we will write the PHP logic that will handle the login process
<?php
define('BASEPATH', true); //access connection script if you omit this line file will be blank
require 'db.php'; //require connection script
if(isset($_POST['submit'])){
// try {
$dsn = new PDO("mysql:host=$host;dbname=$dbname", $user, $password);
$dsn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
//ensure fields are not empty
$username = !empty($_POST['username']) ? trim($_POST['username']) : null;
$passwordAttempt = !empty($_POST['password']) ? trim($_POST['password']) : null;
//Retrieve the user account information for the given username.
$sql = "SELECT id, username, password FROM admin WHERE username = :username";
$stmt = $pdo->prepare($sql);
//Bind value.
$stmt->bindValue(':username', $username);
//Execute.
$stmt->execute();
//Fetch row.
$user = $stmt->fetch(PDO::FETCH_ASSOC);
//If $row is FALSE.
if($user === false){
echo '<script>alert("invalid username or password")</script>';
} else{
//Compare and decrypt passwords.
$validPassword = password_verify($passwordAttempt, $user['password']);
//If $validPassword is TRUE, the login has been successful.
if($validPassword){
//Provide the user with a login session.
$_SESSION['admin'] = $username;
echo '<script>window.location.replace("dashboard.php");</script>';
exit;
} else{
//$validPassword was FALSE. Passwords do not match.
echo '<script>alert("invalid username or password")</script>';
}
}
}
?>
<?php
define('BASEPATH', true); //access connection script if you omit this line file will be blank
require 'db.php'; //require connection script
if(isset($_POST['submit'])){
// try {
$dsn = new PDO("mysql:host=$host;dbname=$dbname", $user, $password);
$dsn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
//ensure fields are not empty
$username = !empty($_POST['username']) ? trim($_POST['username']) : null;
$passwordAttempt = !empty($_POST['password']) ? trim($_POST['password']) : null;
//Retrieve the user account information for the given username.
$sql = "SELECT id, username, password FROM admin WHERE username = :username";
$stmt = $pdo->prepare($sql);
//Bind value.
$stmt->bindValue(':username', $username);
//Execute.
$stmt->execute();
//Fetch row.
$user = $stmt->fetch(PDO::FETCH_ASSOC);
//If $row is FALSE.
if($user === false){
echo '<script>alert("invalid username or password")</script>';
} else{
//Compare and decrypt passwords.
$validPassword = password_verify($passwordAttempt, $user['password']);
//If $validPassword is TRUE, the login has been successful.
if($validPassword){
//Provide the user with a login session.
$_SESSION['admin'] = $username;
echo '<script>window.location.replace("dashboard.php");</script>';
exit;
} else{
//$validPassword was FALSE. Passwords do not match.
echo '<script>alert("invalid username or password")</script>';
}
}
}
?>
<form action="login.php" method="post">
<input type="text" name="username" placeholder="Username">
<input type="password" name="password" placeholder="Password">
<button name="submit" type="submit">sign in</button>
</form>
Conclusion
There we have it. This is how you can create a Login and Register form using PDO with password hash and secure script access. Have any questions? Feel free to leave them in comments.
Create Register and Login Form using Node.js
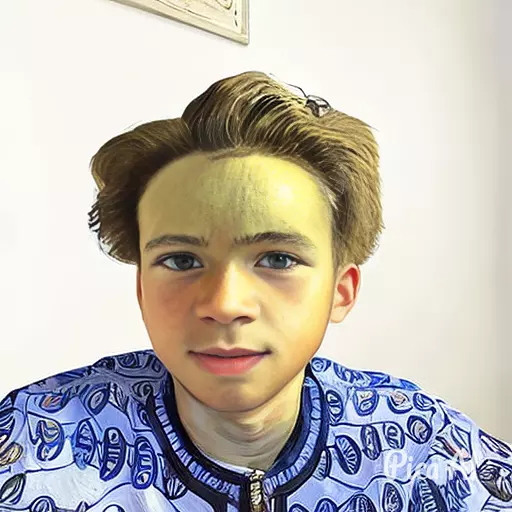
Symantec
HostPenguin