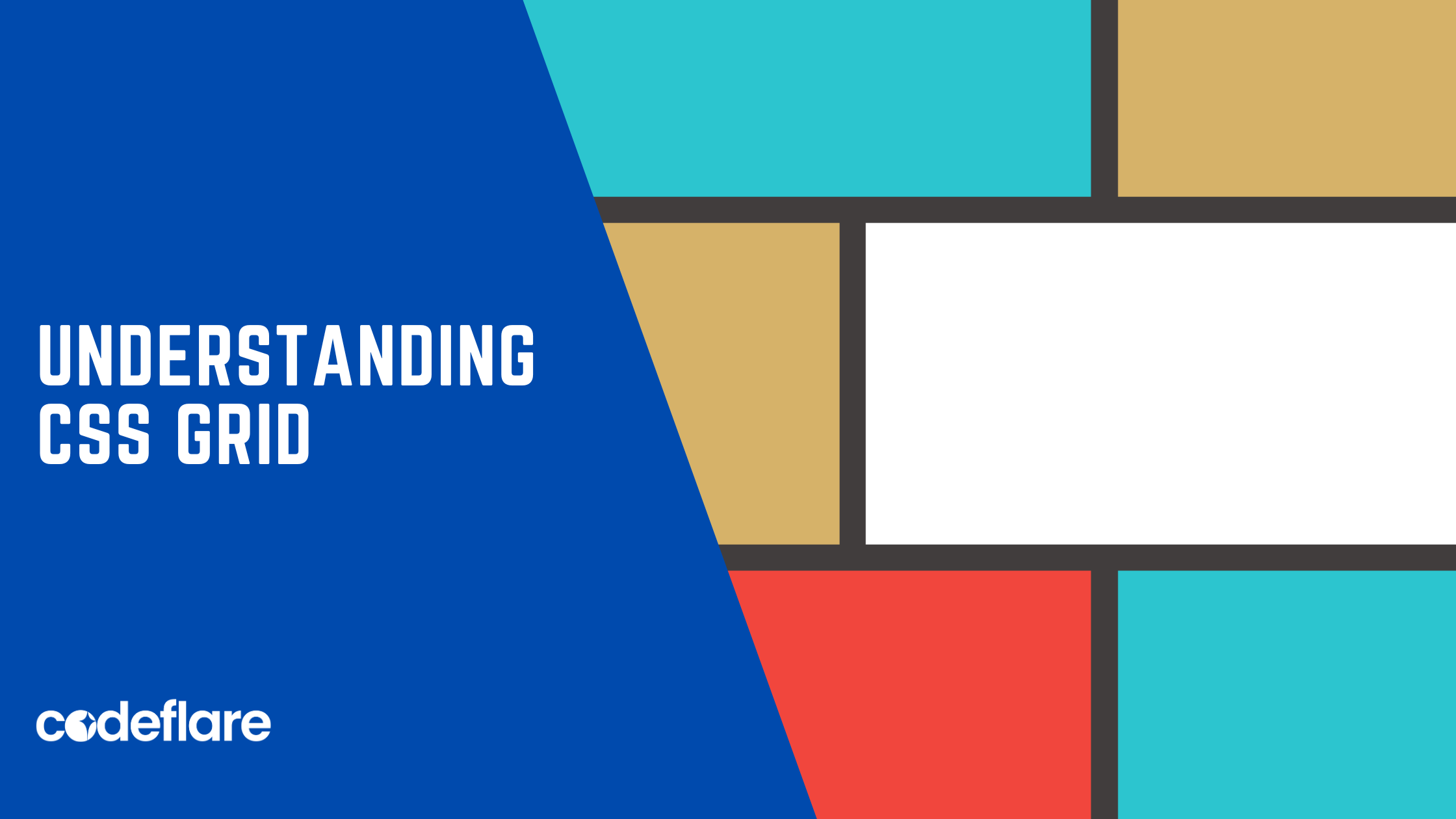
CSS Grid Layout is a powerful two-dimensional layout system that enables developers to create complex and responsive web designs with ease. It provides a grid-based layout system for building web pages, allowing you to arrange elements into rows and columns. In this article, we’ll explore the basics of CSS Grid Layout and how to use it to create flexible and responsive layouts.
Introduction to CSS Grid
CSS Grid Layout is part of the CSS3 specification and is supported by modern web browsers. It offers a more advanced layout mechanism compared to traditional methods like floats and positioning. With CSS Grid, you can define both rows and columns, creating a grid of intersecting tracks.
Creating a Grid Container
To get started with CSS Grid, you need a container element. This container will hold all the items you want to arrange in a grid. Let’s create a simple grid container:
.grid-container {
display: grid;
grid-template-columns: 1fr 1fr 1fr; /* Three equal columns / grid-gap: 20px; / Gap between grid items */
}
In this example, the display
: grid
property is used to create a grid container. The grid-template-columns
property defines the size and number of columns. Here, we have three columns, each taking up an equal fraction of the available space (1fr
). The grid-gap
property sets the gap between grid items.
Placing Items in the Grid
Once you have a grid container, you can place items into it. Each item can span multiple rows and columns, providing flexibility in your layout.
.grid-item {
grid-column: span 2; /* Item spans 2 columns */
grid-row: 1; /* Item is in the first row */
}
In this example, the grid-column
property specifies that the item should span two columns, and grid-row
places it in the first row.
Responsive Grids
One of the key advantages of CSS Grid is its ability to create responsive layouts. By using media queries, you can adjust the layout based on the screen size.
@media (max-width: 600px) {
.grid-container {
grid-template-columns: 1fr; /* Single column on small screens */
}
}
Here, when the screen width is 600 pixels or less, the grid switches to a single column layout.
Browser support and Polyfill
CSS Grid is well-supported in modern browsers. However, if you need to support older browsers, consider using a polyfill like “autoprefixer” to ensure graceful degradation.
Certainly! Here is a simple HTML page using CSS Grid Layout. We’ll create a grid with three columns and two rows, and place some items in it.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>CSS Grid Layout Example</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 20px;
}
.grid-container {
display: grid;
grid-template-columns: 1fr 1fr 1fr;
grid-template-rows: auto auto;
grid-gap: 20px;
}
.grid-item {
padding: 20px;
border: 1px solid #ccc;
text-align: center;
}
@media (max-width: 600px) {
.grid-container {
grid-template-columns: 1fr;
}
}
</style>
</head>
<body>
<div class="grid-container">
<div class="grid-item">Item 1</div>
<div class="grid-item">Item 2</div>
<div class="grid-item">Item 3</div>
<div class="grid-item">Item 4</div>
<div class="grid-item">Item 5</div>
<div class="grid-item">Item 6</div>
</div>
</body>
</html>
Conclusion
CSS Grid Layout is a powerful tool for creating complex and responsive web layouts. By defining a grid container and placing items within it, you can achieve flexible designs that adapt to different screen sizes. Experiment with CSS Grid in your projects to take advantage of its capabilities and streamline your layout code. However, it’s essential to consider browser compatibility and provide fallbacks for older browsers or those that do not support CSS Grid. Therefore, always ensure graceful degradation for a seamless user experience across a variety of environments.