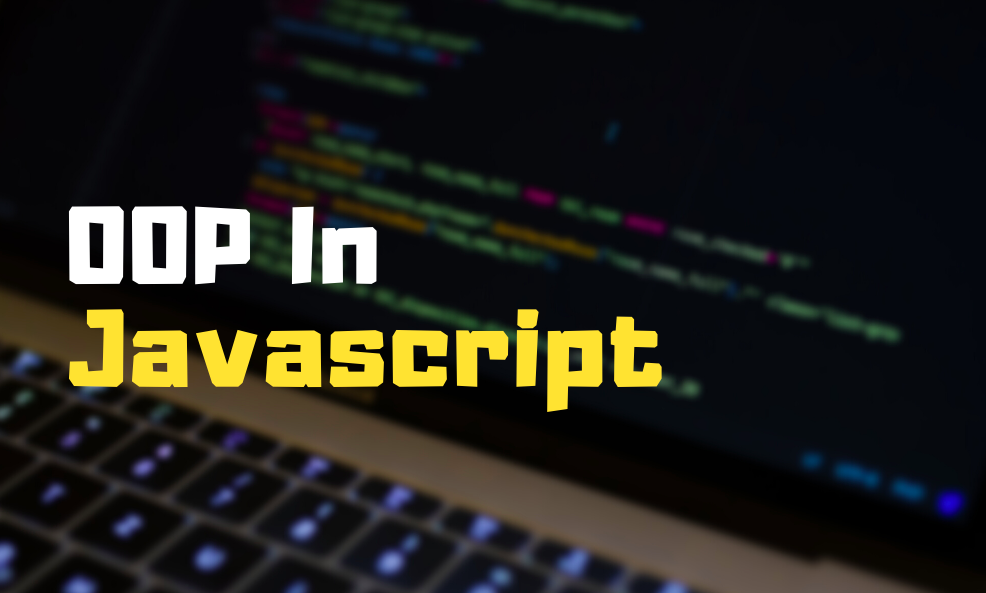
object oriented programming tutorial by codeflare
Object oriented programming is a way of writing code such that different objects (instances) are created from a single object (blueprint).
Each created instance usually have properties that are not shared with other instances.
For example, we can have a ‘Human’ blueprint. This blueprint can have instances such as first name, last name, age, etc. These are unique and peculiar instances that are derived from the ‘Human’ blueprint.
Constructor Functions
Constructors are special functions that contain a “this” keyword.
“this” helps us store and access unique values that are created for each instance.
Example:
function Human(firstName, lastName){
this.firstName = firstName;
this.lastName = lastName;
}
let someGuy = new Human('Prince', 'Rakib');
console.log(someGuy.firstName) //Prince
console.log(someGuy.lastName) //Rakib
Class Syntax
Similar to constructor functions, classes are also a template for creating objects.
They are said to be the “syntactic sugar” of constructor functions. That is, the are an alternative way of writing constructor functions.
Example:
class Human {
constructor(firstName, lastName){
this.firstName = firstName;
this.lastName = lastName;
}
}
let someGuy = new Human('Prince', 'Rakib');
console.log(someGuy.firstName) //Prince
console.log(someGuy.lastName) //Rakib