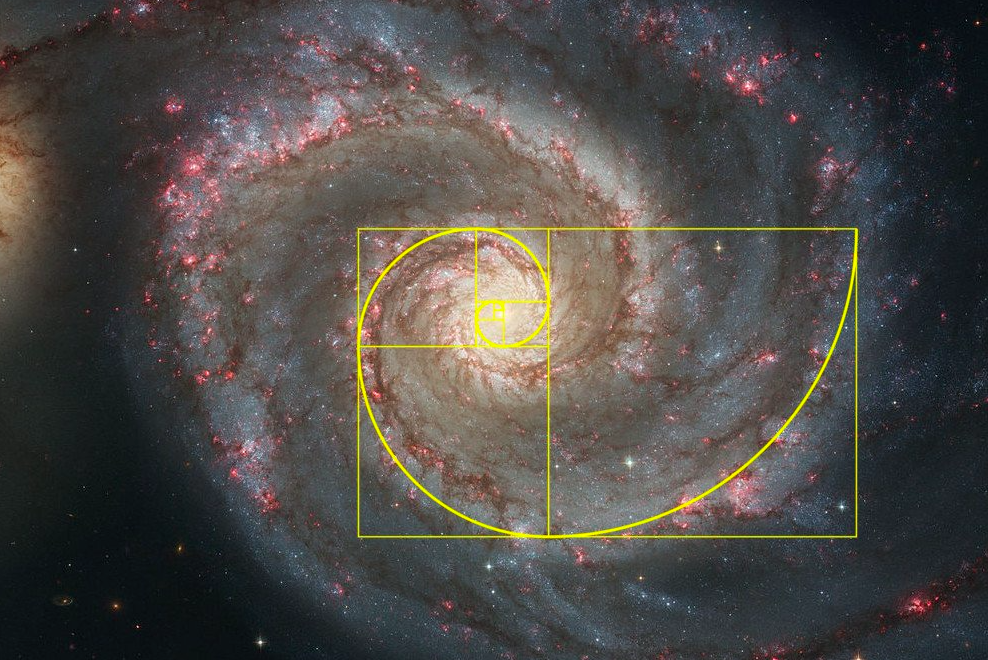
Using recursive functions in javascript
A recursive function is one which defines a problem in terms of itself.
A recursive function calls itself directly or indirectly until it is stopped. If it is not stopped, then it will call itself forever – or maybe until your browser crashes!
Recursive functions let you perform a unit of work multiple times. And while it is similar to a for/while loop, using a recursive solution affords a unique, faster and much more elegant approach to solving a problem.
Let’s write a countdown function, shall we?
- Using Loop:
countDown = (num) => {
for(let i=num; i>0; i--){
console.log(i);
}
}
countDown(6) //5,4,3,2,1
2. Using Recursion
countDown = (num) => {
if(num === 0)
return 0;
console.log(num);
countDown(num - 1);
}
countDown(6) //5,4,3,2,1
A recursive function usually contains what is referred to as a “base case.”
A base case is a condition that checks and stops the recursion. It essentially prevents an infinite loop.
In the above example, the snippet below:
if(num === 0)
return;
is the base case, a condition that checks our program.
More examples …
Supposing we want to write a solution that performs a raise-to-power operation, eg., 23.
We can use the inbuilt Math function in Javascript like so:
Math.pow(2,3)
//result is 8
We can also write a Loop solution as follows:
pow = (a, b) => {
let result = 1;
for(let i=0; i<b; i++){
result *=a;
}
return result;
}
alert(pow(2,3));
//result is 8
And then we can think in terms of recursion by doing the following:
pow = (a, b) => {
if(n==1){
return a;
}else{
return a * pow(a, b-1);
}
}
alert(pow(2,3));
//result is 8
Task: Sum all numbers till the given one.
E.g 5 = 1+2+3+4+5 = 15;
Using recursion, we would do the following
sumAll = (n) => {
if(n === 0){
return 0;
}else{
return n += sumAll(n-1);
}
}
alert(sumAll(5)) //15
There you go. you can start using recursion for your automated programming tasks.