A loop statement allows the programmer to execute a statement or group of statements multiple number of times, based on the given conditions.
Let’s take a look 4 types of loops in Java.
- The While Loop
This repeats a statement or group of statements while a given condition is true. It tests the condition before executing the loop body.
//Syntax
while(expression){
//statements to be executed ...
}
//Print out all numbers from 0 to 100
public class Looper{
public static void main(String args[]){
int x = 0;
while(x<=100){
System.out.println(x);
x++;
System.out.println("\n"); //new line for vertical output
}
}
}
2. For Loop
A for loop is a repetition control structure that allows you to efficiently write a loop that needs to be executed a specific number of times.
A for loop is useful when you know how many times a task is to be repeated.
//Syntax
for(initialization; expression; iteration){
//Statements to be executed;
}
a. Initialization: this step is executed first and only once. It allows you to declare and initialize any loop control and it ends with a semi-color.
b. Expression: next, the expression is executed. If it is true, the body of the loop is executed, else the loop is terminated.
c. Iteration: if the expression is true, the iteration lets you update any loop control variables. This part can be left blank and without a semi-colon at the end.
//print all values from 0 to 100
public class ForLoop{
public static void main(String args[]){
for(int i=0; i<=100; i++){
System.out.println(i);
}
}
}
3. Do … While Loop
A do/while loop is similar to a while loop except that it is guaranteed to execute at least once.
//Syntax
do{
//statements to be executed
}while(expression);
The statement executes once before the expression is tested. If the expression is true, the control jumps back up to the do statement, and the statements in the loop execute again. The process repeats itself until the boolean expression is false.
//print all numbers from 1 to 100
public class DoWhile{
public static void main(String args[]){
int x = 0;
do{
System.out.println(x);
x++;
}while(x<=100);
}
}
4. Enhanced For Loop
Enhanced for loop is used to traverse through a collection of elements, including arrays.
//Syntax
for(declaration: expression){
//statements
}
public class EnForLoop{
public static void main(String args[]){
int [] numbers = {10, 20, 30, 40, 50};
for(int x : numbers){
System.out.println(x); //10, 20, 30, 40, 50
}
}
}
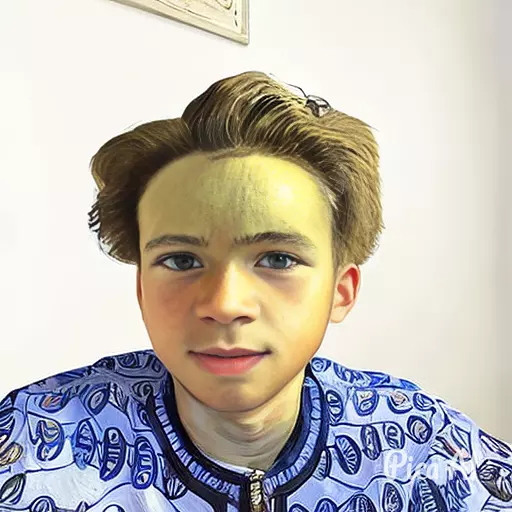