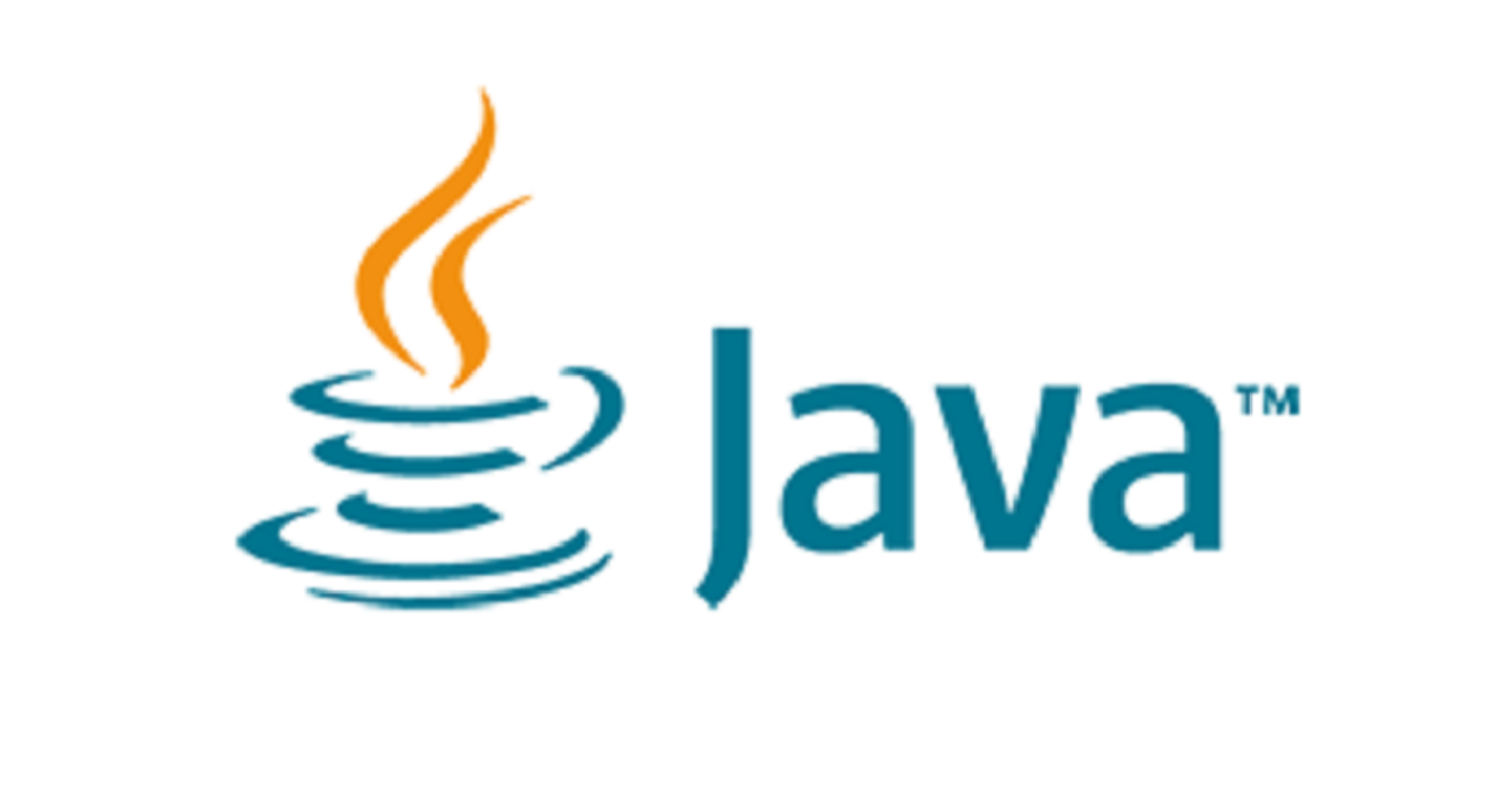
Modifiers are keywords that are added to variables, methods and classes to change their meaning.
There are two (2) types of modifiers:
- Access modifiers
- Non-access modifiers
Access Modifiers
There are four (4) access levels in Java, which are:
- Default. This is by default visible to the package and no modifiers are needed here.
- public (visible to the world)
- private (visible to the class only)
- protected (visible to the package and subclass)
Default Access Modifier
Default access modifier means that we do not explicitly declare any access modifier for a class, field, method, etc. This makes it accessible to any other class in the same package.
Private Access Modifier
Methods, variables and constructors that are declared private can only be accessed within the declared class itself. Private access modifier is the most restrictive access level. But you should note that class and interface cannot be private.
Protected Access Modifier
Variables, methods and constructors which are declared as protected in a superclass can be accessed only by the subclass in other package or any class within the package of the protected members’ class.
Public Access Modifier
This makes the method accessible to the whole world.
Non-access Modifiers
- Static Modifiers
(i) Static variables:
The static keyword is used to create variables that will exist independently of any instances created for the class. Only one copy of the static variable exists, regardless of the number of instances of the class.
Static variables are also known as class variables. Local variables cannot be declared static.
(ii) Static methods
This creates methods that will exist independently of any instances created fo the class. Static methods do not use any instance variables of any object of the class they are defined in. Static methods take no reference to variables.
2. Final Modifiers
(i) Final variables
A final variable can only be initialized once. The final modifier is often used with the static keyword to create a constant and thereby make it a class variable. A final variable cannot be assigned another value.
(ii) Final class
A final class cannot be extended or subclassed.
The below example will not compile:
final class Animal {
//statements here ...
}
class Dog extends Animal {
//this will not throw an error
}
(iii) Final methods
When we use the final specifier with a method, the method cannot be overridden in any of the inheriting classes.
It is also necessary to point out that since private methods are inaccessible, they are implicitly final in Java.
3. Abstract Modifiers
(i) Abstract class:
Abstract classes can never be instantiated.
(ii) Abstract methods:
Abstract methods are methods declared are methods that are declared without any implementation and usually ends with a semi-colon.