Inheritance in programming is the process whereby one class (also called a sub-class) acquires the properties (methods, fields, etc) of another (in this case a super class) using the extends keyword. This makes information manageable in a hierarchical manner.
With inheritance, you can create new classes that are built upon existing classes. When you inherit an existing class, you can reuse methods and fields of the parents class and you can add new methods and fields as well.
Some of the reasons for using inheritance are for method overriding and code reusability.
Types of Inheritance
- Single Inheritance
Example
class Animal{
void eat(){
System.out.println("eating");
}
}
class Dog extends Animal{
void bark(){
System.out.println("barking");
}
}
class TestClass {
public static void main(String args[]){
Dog dog = new Dog();
dog.bark(); //barking
dog.eat(); //eating
}
}
2. Multi-level Inheritance
In a multi-level inheritance, several classes can inherit the properties and methods of each other in a multi-level setting.
class Animal{
void eat(){
System.out.println("eating");
}
}
class Dog extends Animal{
void bark(){
System.out.println("barking");
}
}
class BabyDog extends Dog{
void play(){
System.out.println("A puppy plays");
}
}
class TestClass{
public static void main(String args[]){
BabyDog babydog = new BabyDog();
babydog.play(); //A puppy plays
babydog.bark(): //barking
babydog.eat(); //eating
}
}
3. Hierarchical Inheritance
In a hierarchical inheritance, every subclass inherits from the super class and hence can only call properties of the super class alone. A subclass cannot call properties from another subclass.
class Animal{
void eat(){
System.out.println("eating");
}
}
class Dog extends Animal{
void bark(){
System.out.println("barking");
}
}
class Cat extends Animal{
void meow(){
System.out.println("cat meows")
}
}
class TestClass{
public static void main(String args[]){
Cat c = new Cat();
cat.eat(); //eating
cat.meow(); //cat meows
cat.bark(); //Error! you will have to instantiate the Dog class like so:
Dog dog = new Dog();
dog.eat(); //eating.
}
}
Thank you for reading.
The End.
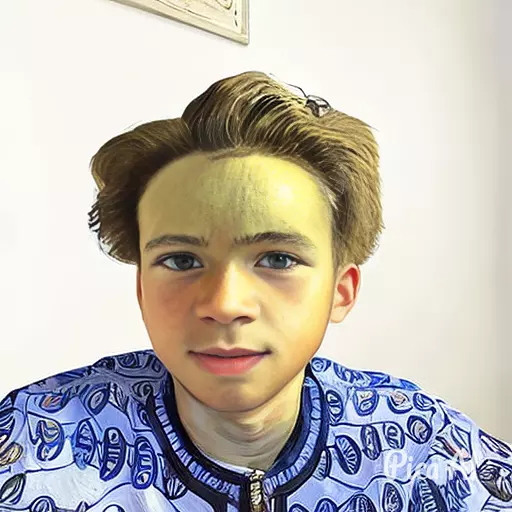