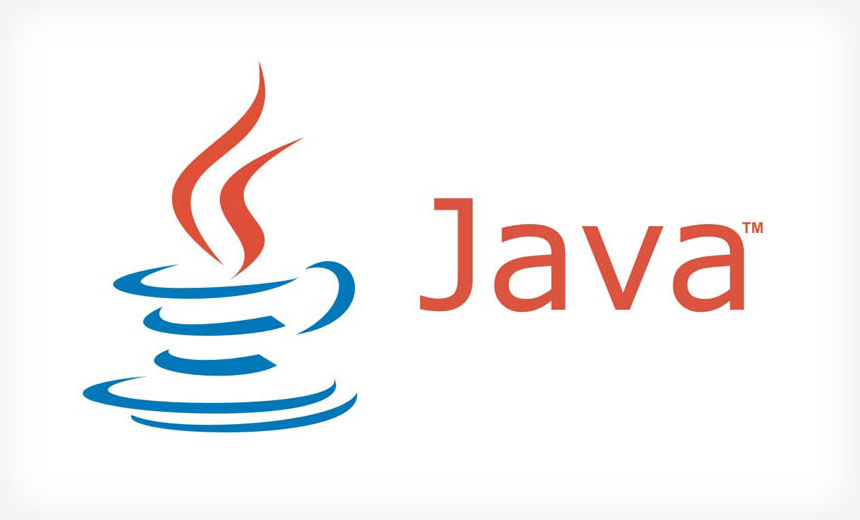
Handling exceptions in Java
An exception is a problem that arises during the execution of a program.
When an exception occurs, the normal flow of the program is disrupted and the program or application terminates abruptly.
An exception can occur for several reasons, some of which include:
- A user has entered an invalid data
- A requested resource was not found
- Network failure
Some of these exceptions can be caused by user error, others programmer’s error, or missing requested resource.
Three (3) Categories of Exceptions
- Checked Exceptions:
A checked exception is one that occurs at compile time. These are also called compile-time exceptions. These exceptions cannot just be ignored at the time of compilation, the programmer has to take care of it.
2. Unchecked Exceptions
An unchecked exception is an exception that occurs at the time of execution. These are also called Run-time Exceptions. An example of this is programming bugs, such as logic errors. Runtime exceptions are ignored at the time of compilation.
Example
public class Unchecked_Exception {
public static void main(String args[]){
int num[] = {1,2,3,4}
System.out.println(num[5])
//Result: ArrayIndexOutOfBoundsException
3. Errors
These are not categorically exceptions but problems that arise beyond the control of the user of the program. Errors are typically ignored because there is very little the user can do to control it. They are also ignored at the time of compilation.
Built-in Exceptions
Java defines several exceptional classes inside the standard java.lang package. Since java.lang is implicitly imported into all Java programs, most exceptions derived from the Runtime Exception are automatically available.
Catching Exceptions
A method catches an exception using a combination of the try/catch keywords.
A try/catch block is placed around the code that might generate an exception. Code within a try/catch block is referred to as a protected code. It has the following syntax:
try{
//Protected code
}catch(ExceptionReference e){
//catch block
}
The error variable e throws more light on what type of exception might have occurred.
A catch statement involves declaring the type of exception you are trying to catch.
Example
import java.io.*
public class ExceptionClass{
public static void main(String args[]){
try{
int num[] = {1,2,3,4};
System.out.println(num[6]);
}catch(ArrayIndexOutOfBoundsException e){
System.out.println("An error occured: " + e);
}
}
}
See programming paths that you can take