Throw
Throw is a keyword that is used in Java to declare an exception which is similar to the try/catch block. It is used to declare an explicit exception inside a block of code or method.
Example
public class AgeCheck {
void checkAge(int age) {
if(age < 18){
throw new ArithmeticException("Not eligible to vote");
}else {
System.out.println("Eligible to vote");
}
}
public static void main(String args[]){
//Create an object of the class to access the method
AgeCheck ageCheck = new AgeCheck();
ageCheck.checkAge(13) //Not eligible to vote
}
}
Throws
Throws is a keyword also as well as a method signature used to declare an exception which might be thrown by the method during the program execution.
public class DivisionCheck {
int divide(int a, int b) throws ArithmeticException {
int result = a/b;
return result;
}
public static void main(String args[]){
//Create an object of the class to access the method
DivisionCheck divisionCheck = new DivisionCheck();
try {
System.out.println(divisionCheck.divide(15,0));
}catch(ArithmeticException e){
System.out.println("You cannot divide by zero);
}
}
}
Throws is usually used to check the process of program execution, especially when you are not sure of the input you might get from the user.
THE END.
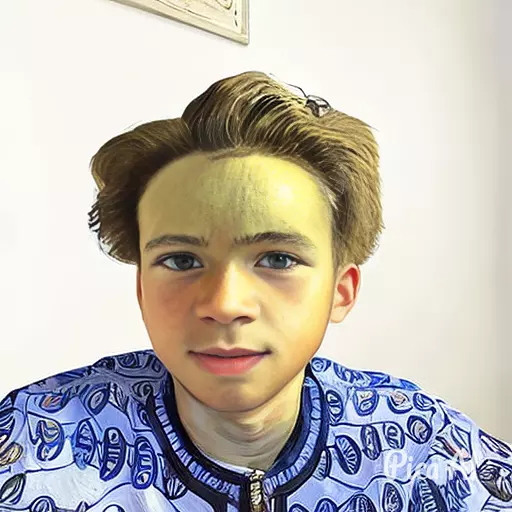