JavaScript is a versatile programming language that powers the dynamic nature of the web. With the introduction of the Fetch API, JavaScript developers gained a powerful tool for making network requests and interacting with servers.
The Fetch API provides a modern, streamlined alternative to the traditional XMLHttpRequest (XHR) approach. In this tutorial, we will explore the Fetch API in detail, its core features, and how it simplifies asynchronous data fetching in JavaScript applications. We will also create a GET request using the Fetch API by consuming the JSON dog placeholder API as our example endpoint.
What is Fetch API
The Fetch API is a built-in JavaScript interface that enables fetching resources asynchronously across the network. It provides a simplified, promise-based syntax for making HTTP requests, allowing developers to send and receive data from servers with ease. The Fetch API supports a wide range of data formats, including JSON, text, blobs, and more.
How to Make a Fetch Request
Using the Fetch API is straightforward. To initiate a basic fetch request, we create a new instance of the fetch()
function and pass in the URL of the resource we want to fetch. The fetch()
function returns a Promise that resolves to the Response object containing the server’s response.
We can then use the Response object’s methods to handle the retrieved data. For example, we can call response.json()
to extract JSON data, response.text()
to get plain text, or response.blob()
to retrieve binary data. These methods also return Promises, allowing us to chain them together or use async/await syntax for cleaner code.
Now, back to our example. What we want to do is create a simple HTML page and using just a bit of Bootstrap. We will show the Bootstrap spinner for the waiting period.
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-gH2yIJqKdNHPEq0n4Mqa/HGKIhSkIHeL5AyhkYV8i59U5AR6csBvApHHNl/vI1Bx" crossorigin="anonymous">
<title></title>
</head>
<body>
<div class="container">
<center>
<div class="spinner-border" role="status" id="loading"></div>
</center>
<div class="row row-cols-1 row-cols-md-3 g-4" id="data"></div>
</div>
Next, we will define our Async function.
<script>
const api_url =
"https://catfact.ninja/breeds";
// Defining async function
async function getapi(url) {
// Storing response
const response = await fetch(url);
// Storing data in form of JSON
var data = await response.json();
console.log(data);
if (response) {
hideloader();
}
show(data);
}
</script>
Next, we need to call the getapi function.
// Calling that async function
getapi(api_url);
Now, you should be able to see some data in your developer console.
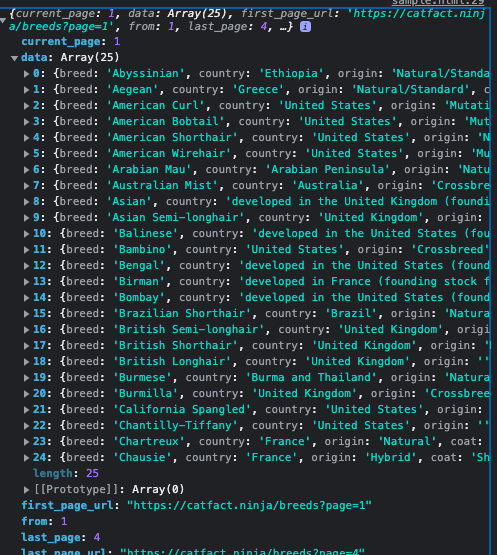
Next, we iterate over the data and display them in the HTML tag.
// Loop to access all rows
for (let item of data.data) {
// let description = item.course_description.substring(0, 100).concat('...')
tab += `
<div class="col-12 col-md-6 mb-6 col-lg-4 d-flex">
<div class="card shadow-light-lg mb-6 mb-md-0 lift lift-lg">
<div class="card-body position-relative">
<div class="position-relative text-right mt-n8 mr-n4 mb-3">
<span class="badge badge-pill badge-success">
<span class="h6 text-uppercase"><i class="fa fa-check"></i></span>
</span>
</div>
<h3 class="text-capitalize" style="font-family:poppins" >
${item.breed}
</h3>
<p class="text-muted">
${item.country}
</p>
<p>${item.origin}</p>
</div>
</div>
</div>
`;
}
// Setting innerHTML as tab variable
document.getElementById("data").innerHTML = tab;
}
Now, we want to tie our function to the load event listener so that when the page loads, the function starts to run.
Here’s the full code:
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-gH2yIJqKdNHPEq0n4Mqa/HGKIhSkIHeL5AyhkYV8i59U5AR6csBvApHHNl/vI1Bx" crossorigin="anonymous">
<title></title>
</head>
<body>
<div class="container">
<center>
<div class="spinner-border" role="status" id="loading"></div>
</center>
<div class="row row-cols-1 row-cols-md-3 g-4" id="data"></div>
</div>
<script>
window.addEventListener('load', (event) => {
const api_url =
"https://catfact.ninja/breeds";
// Defining async function
async function getapi(url) {
// Storing response
const response = await fetch(url);
// Storing data in form of JSON
var data = await response.json();
console.log(data);
if (response) {
hideloader();
}
show(data);
}
// Calling that async function
getapi(api_url);
// Function to hide the loader
function hideloader() {
document.getElementById('loading').style.display = 'none';
}
// Function to define innerHTML for HTML table
function show(data) {
let tab = ``;
// Loop to access all rows
for (let item of data.data) {
// let description = item.course_description.substring(0, 100).concat('...')
tab += `
<div class="col-12 col-md-6 mb-6 col-lg-4 d-flex">
<div class="card shadow-light-lg mb-6 mb-md-0 lift lift-lg">
<div class="card-body position-relative">
<div class="position-relative text-right mt-n8 mr-n4 mb-3">
<span class="badge badge-pill badge-success">
<span class="h6 text-uppercase"><i class="fa fa-check"></i></span>
</span>
</div>
<h3 class="text-capitalize" style="font-family:poppins" >
${item.breed}
</h3>
<p class="text-muted">
${item.country}
</p>
<p>${item.origin}</p>
</div>
</div>
</div>
`;
}
// Setting innerHTML as tab variable
document.getElementById("data").innerHTML = tab;
}
})
</script>
</body>
</html>
Here’s what the result looks like:
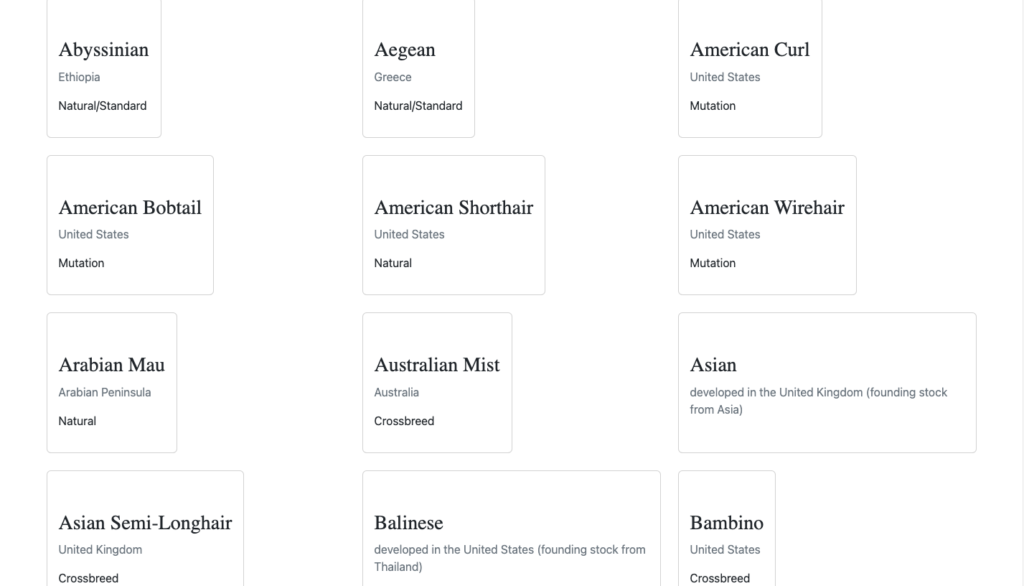
Conclusion
The Fetch API has become an essential part of modern JavaScript development, offering a more intuitive and flexible way to fetch resources from servers. Its simplicity, promise-based nature, and support for various data formats make it a powerful tool for building web applications that rely on dynamic data retrieval.
By leveraging the Fetch API, developers can handle asynchronous network requests with ease, handle errors gracefully, and customize requests to suit their application’s needs. Whether it’s fetching data from APIs, uploading files, or sending form data, the Fetch API provides a versatile solution.
As the web continues to evolve, the Fetch API remains a valuable addition to a JavaScript developer’s toolkit. By mastering this powerful API, developers can unlock new possibilities for creating fast, interactive, and data-driven web applications.
Build a New App with Javascript ES6
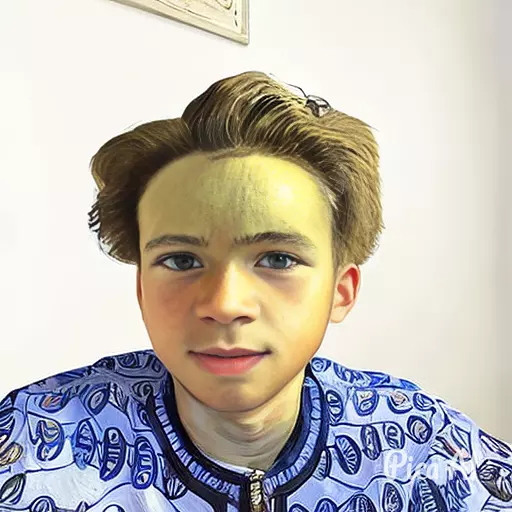