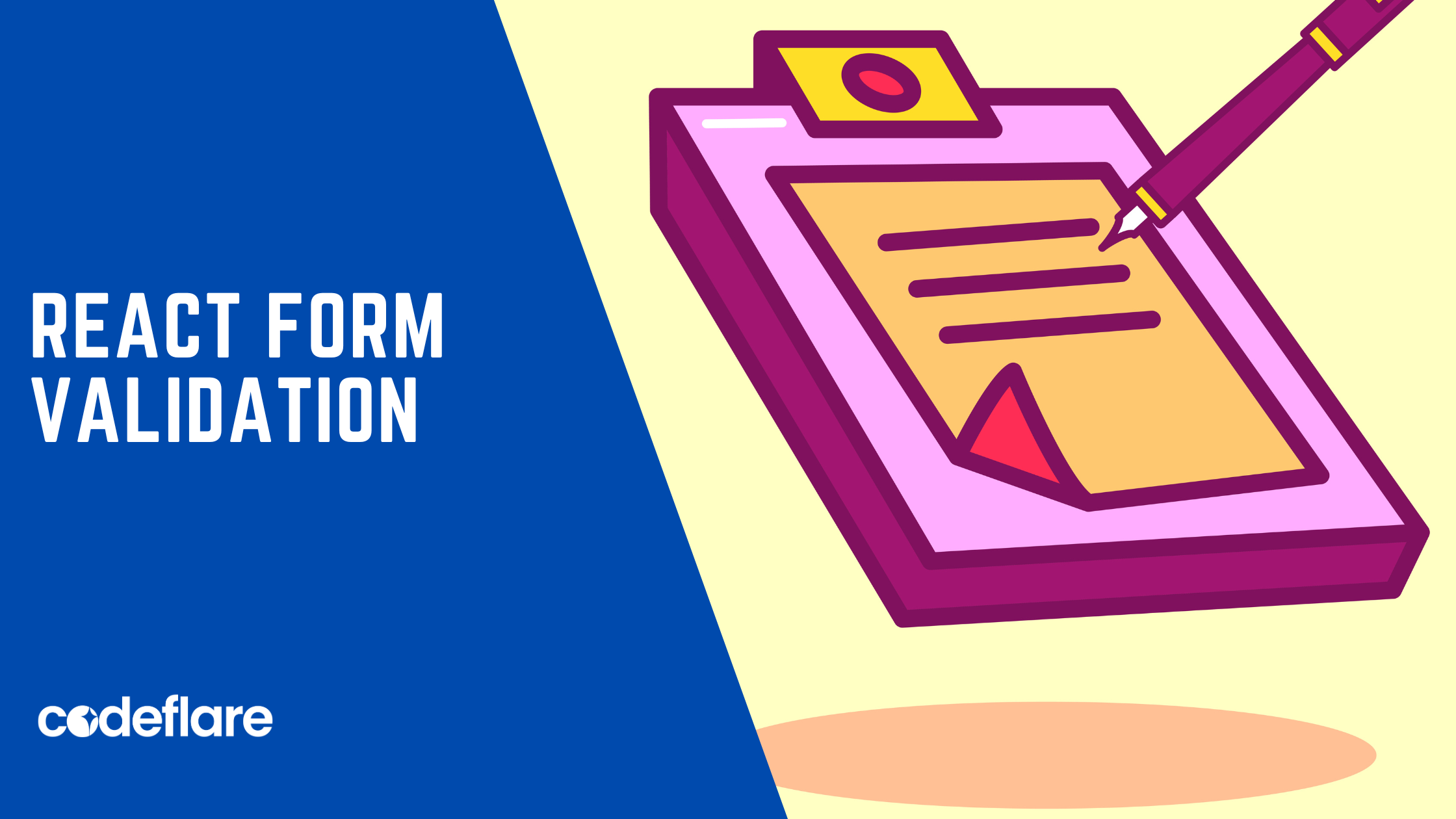
Forms play a crucial role in web applications, enabling users to input and submit data. React provides a powerful way to handle forms, making it efficient to manage user input and update the application state. In this article, we’ll explore various techniques and best practices for handling forms in React.
1. Introduction to React Forms
In React, forms are typically implemented using controlled components. A controlled component is a form element whose value is controlled by the React state. This allows React to be the single source of truth for the form data.
import React, { useState } from 'react';
const MyForm = () => {
const [formData, setFormData] = useState({
username: '',
email: '',
});
const handleInputChange = (e) => {
const { name, value } = e.target;
setFormData({
...formData,
[name]: value,
});
};
const handleSubmit = (e) => {
e.preventDefault();
// Process the form data
console.log(formData);
};
return (
<form onSubmit={handleSubmit}>
<label>
Username:
<input type="text" name="username" value={formData.username} onChange={handleInputChange} />
</label>
<br />
<label>
Email:
<input type="email" name="email" value={formData.email} onChange={handleInputChange} />
</label>
<br />
<button type="submit">Submit</button>
</form>
);
};
export default MyForm;
In this example, the formData
state holds the values of the form inputs, and the handleInputChange
function updates the state when an input value changes.
2. Form Validation
Validating user input is a crucial part of handling forms. React makes it easy to implement form validation by checking the input against certain criteria.
// Inside MyForm component
const [errors, setErrors] = useState({});
const validateForm = () => {
let valid = true;
const newErrors = {};
// Validate username
if (!formData.username.trim()) {
newErrors.username = 'Username is required';
valid = false;
}
// Validate email
const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
if (!formData.email.match(emailRegex)) {
newErrors.email = 'Invalid email format';
valid = false;
}
setErrors(newErrors);
return valid;
};
const handleSubmit = (e) => {
e.preventDefault();
if (validateForm()) {
// Process the form data
console.log(formData);
}
};
In this example, the validateForm
function checks the validity of the form inputs and updates the errors
state accordingly. The form is submitted only if the validation passes.
3. Handling Different Form Elements
React provides various form elements, including text inputs, checkboxes, radio buttons, and select elements. Handling these elements involves slightly different approaches.
Textarea:
<label>
Message:
<textarea name="message" value={formData.message} onChange={handleInputChange} />
</label>
Checkbox:
<label>
<input type="checkbox" name="subscribe" checked={formData.subscribe} onChange={handleInputChange} />
Subscribe to newsletter
</label>
Radio Buttons:
<label>
<input type="radio" name="gender" value="male" checked={formData.gender === 'male'} onChange={handleInputChange} />
Male
</label>
<label>
<input type="radio" name="gender" value="female" checked={formData.gender === 'female'} onChange={handleInputChange} />
Female
</label>
Select Element:
<label>
Country:
<select name="country" value={formData.country} onChange={handleInputChange}>
<option value="usa">USA</option>
<option value="canada">Canada</option>
<option value="uk">UK</option>
</select>
</label>
4. Working with Form Libraries in React
For complex forms, you might consider using form libraries such as Formik or React Hook Form. These libraries provide additional features like form validation, error handling, and form submission management.
Formik:
npm install formik
import { Formik, Form, Field, ErrorMessage } from 'formik';
const MyForm = () => {
return (
<Formik
initialValues={{ username: '', email: '' }}
validate={(values) => {
const errors = {};
// Validation logic here
return errors;
}}
onSubmit={(values) => {
// Handle form submission
console.log(values);
}}
>
<Form>
<label>
Username:
<Field type="text" name="username" />
<ErrorMessage name="username" component="div" />
</label>
<br />
<label>
Email:
<Field type="email" name="email" />
<ErrorMessage name="email" component="div" />
</label>
<br />
<button type="submit">Submit</button>
</Form>
</Formik>
);
};
export default MyForm;
React Hook Form:
npm install react-hook-form
import { useForm } from 'react-hook-form';
const MyForm = () => {
const { register, handleSubmit, errors } = useForm();
const onSubmit = (data) => {
// Handle form submission
console.log(data);
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
<label>
Username:
<input type="text" name="username" ref={register({ required: 'Username is required' })} />
{errors.username && <div>{errors.username}</div>}
</label>
<br />
<label>
Email:
<input type="email" name="email" ref={register({ required: 'Email is required' })} />
{errors.email && <div>{errors.email}</div>}
</label>
<br />
<button type="submit">Submit</button>
</form>
);
};
export default MyForm;
5. Conclusion
Handling forms in React involves managing state, validating user input, and working with various form elements. By utilizing controlled components and integrating form libraries, you can create robust and maintainable forms that enhance the user experience in your React applications.
Create a video component with React