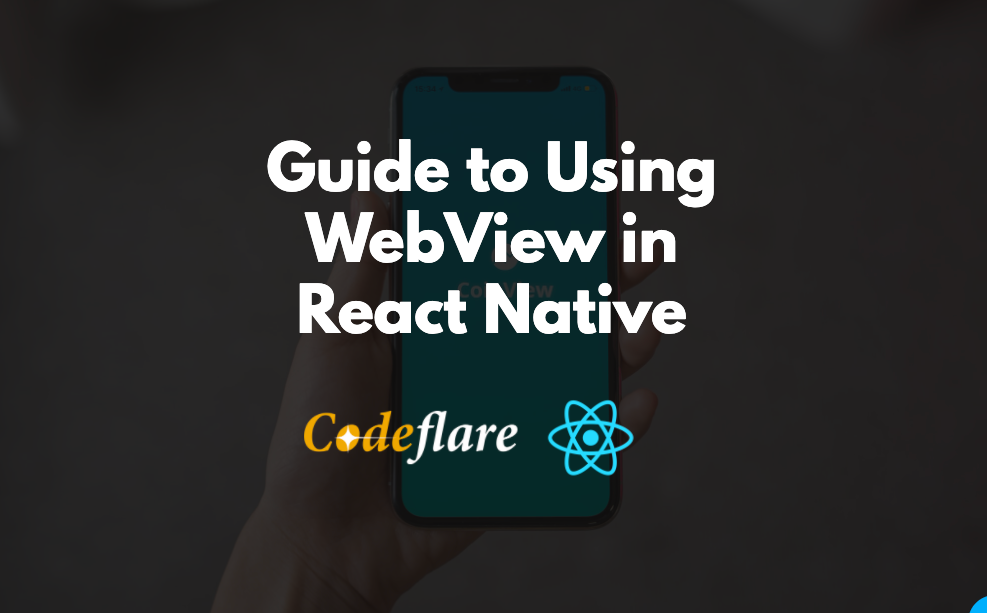
WebView is essentially a component that is used to load a web pages in your React Native project.
WebView sometimes become necessary in your software development project, especially when you have a website that is already built and you would like to create an app off of it.
How to Use React Native WebView
To use WebView we have to first import it as a component.
yarn add react-native-webview
Next, we use the WebView component as follows.
import React, { Component } from 'react';
import {View} from 'react-native';
import { WebView } from 'react-native-webview';
class App extends Component {
render(){
return(
<WebView source={{ uri: 'https://theproli.com/index' }}
ref={(ref) => { this.webview = ref; }}
scalesPageToFit={ true }
renderError={ () => this.loadError() }
/>
)
}
}
export default App
If we run the above code, initially we might not really know if the page is loading or not.
So we want to show the use an activity, like a spinner, while the page loads.
This is so that the user will know that something is going on.
constructor(props){
super(props);
this.state = {
visible: true
}
}
hideSpinner() {
this.setState({ visible: false });
}
Then we need to call the function onLoad() in the WebView component.
Full code:
import React, { Component } from 'react';
import {View} from 'react-native';
import { WebView } from 'react-native-webview';
class App extends Component {
constructor(props){
super(props);
this.state = {
visible: true
}
}
hideSpinner() {
this.setState({ visible: false });
}
render(){
return(
<WebView source={{ uri: 'https://theproli.com/index' }}
ref={(ref) => { this.webview = ref; }}
onLoad={() => this.hideSpinner()}
scalesPageToFit={ true }
renderError={ () => this.loadError() }
/>
{this.state.visible && (
<ActivityIndicator
style={{ position: "absolute", alignSelf:'center', flexDirection:'row', top: 0, left: 0, right: 0, bottom:0 }}
size="large"
color="#0275d8"
/>
)}
)
}
}
export default App
Read more on WebView in the React Native docs