The React Library allows you to define components either as classes or functions. These components allow you to split your UI into independent, reusable pieces, and help you think about each piece in isolation.
You could think of these components as functions in JavaScript. These functions usually have arbitrary inputs (called “props”) and return React elements and describes what should appear on the screen.
In order to invoke a React lifecycle method, you will need to define a component either as a function or as a class. For the sake of this tutorial, we are going to define our components as a class.
Defining a Class Component
To define a class component in React, your class needs to extend React Component. See example below:
import React, {Component} from 'react';
class YourClassName extends Component {
//do actions here
}
Now, notice the import statement at the top of our code. That line is necessary for our extended class to work properly.
“YourClassName” is typically the name of your file in which you are creating the component. This could be App.js, Home.js, or whatever class name you decide to use.
Next, we need to export this our newly created component so that it can be available globally to the our application. If you fail or forget export it, your application will throw an error when you try to use that component.
import React, {Component} from 'react';
class YourClassName extends Component {
//do actions here
}
export default YourClassName;
Mounting a Component
To make a component available and inserted into the DOM, we need to render it and return some texts.
import React, {Component} from 'react';
import { View, Text } from 'react-native';
class YourClassName extends Component {
render(){
return(
<View>
<Text>This is a component</Text>
</View>
)
}
}
export default YourClassName;
When we run this code, we will get the result on the screen:
This is a component
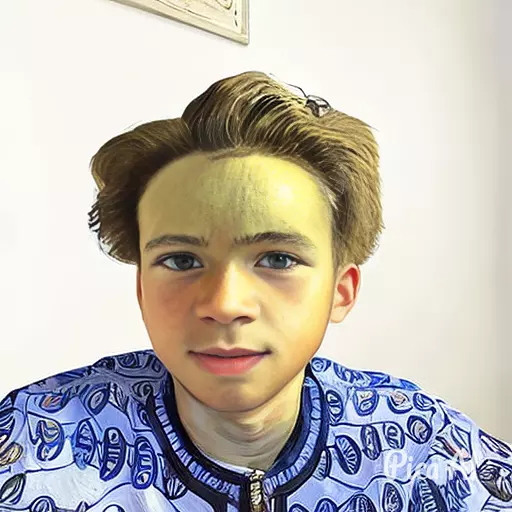
Thank you for your wonderful Content, at least you given us hope in the programming world especially in Nigeria.
Thank you.