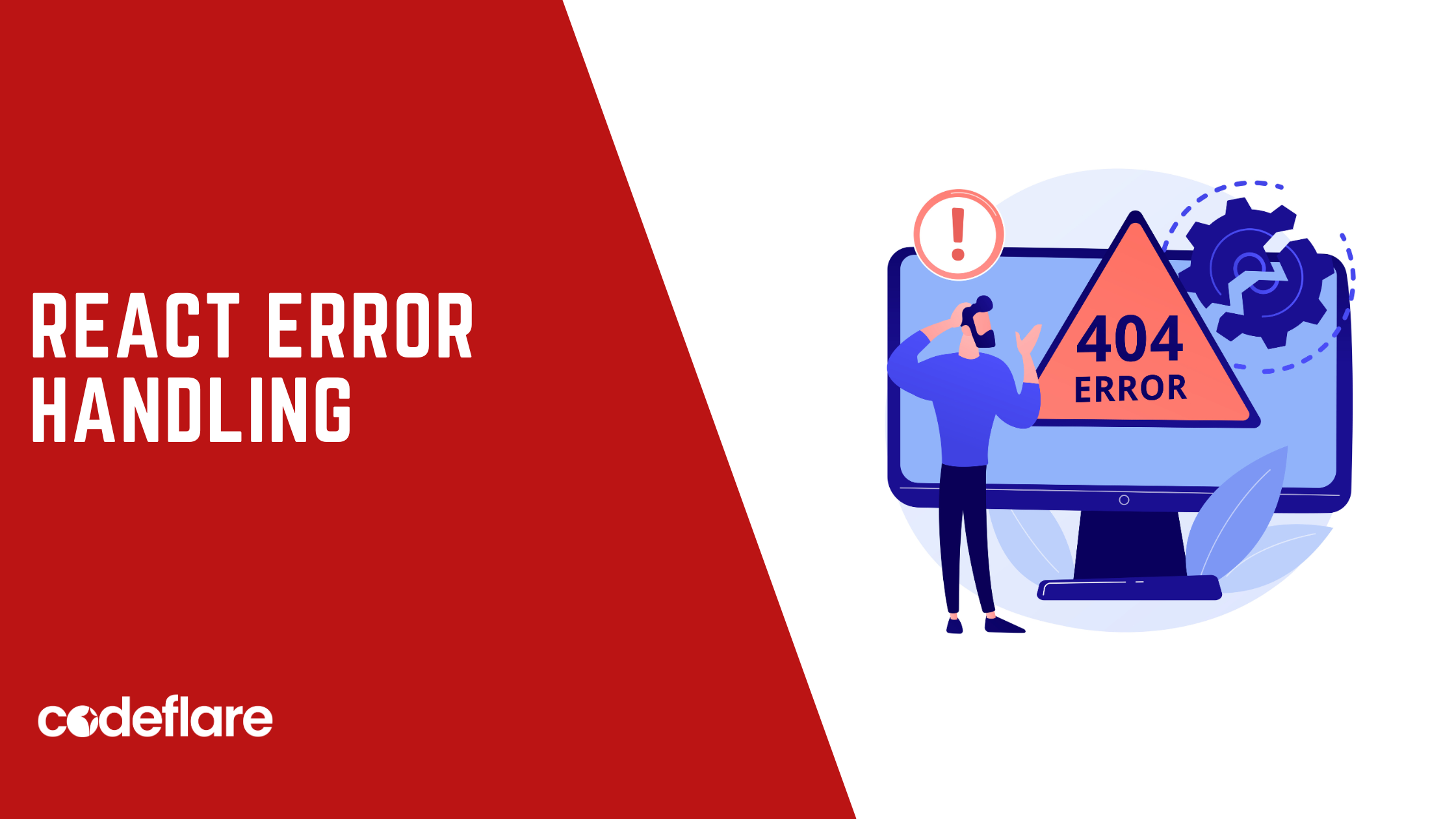
Errors are an inevitable part of software development, and React applications are no exception. In this article, we’ll explore strategies for handling errors gracefully in React applications, including the use of error boundaries and error logging techniques.
Understanding Errors in React
Before diving into error handling strategies, it’s essential to understand the types of errors that can occur in React applications:
- Component Errors: Errors that occur within individual components due to invalid state, improper data handling, or other issues.
- Asynchronous Errors: Errors that occur during asynchronous operations, such as fetching data from an API or handling user input.
- Rendering Errors: Errors that occur during the rendering process, such as invalid JSX syntax or missing components.
Error Boundaries
React provides a built-in mechanism called error boundaries for handling errors that occur within a component tree. Error boundaries are special components that catch errors anywhere in their child component tree and display a fallback UI instead of crashing the entire application.
Implementing Error Boundaries
To create an error boundary in a React application, you can define a component that extends the React.Component
class and implements the componentDidCatch
lifecycle method:
class ErrorBoundary extends React.Component {
state = { hasError: false };
componentDidCatch(error, errorInfo) {
this.setState({ hasError: true });
// Log the error to an error reporting service
logErrorToService(error, errorInfo);
}
render() {
if (this.state.hasError) {
// Render fallback UI
return <FallbackUI />;
}
return this.props.children;
}
}
You can then wrap any part of your component tree with the ErrorBoundary
component to catch errors within that subtree:
<ErrorBoundary>
<MyComponent />
</ErrorBoundary>
Error Logging
In addition to displaying a fallback UI, it’s essential to log errors for debugging and monitoring purposes. Error logging allows developers to track and diagnose issues in production environments.
Implementing Error Logging
There are several ways to implement error logging in a React application:
- Console Logging: Use the
console.error()
method to log errors to the browser console for immediate visibility during development. - Client-Side Logging Libraries: Integrate client-side logging libraries like Sentry or Bugsnag to capture and report errors to a remote server for analysis.
- Server-Side Logging: Log errors on the server-side by sending error data to a logging service or storing them in a database for later analysis.
const logErrorToService = (error, errorInfo) => {
// Log the error and errorInfo to a remote server
// Example: Sentry.captureException(error);
};
Conclusion
Error handling is a critical aspect of building robust and reliable React applications. By using error boundaries and implementing error logging, developers can detect, diagnose, and recover from errors effectively, ensuring a smooth user experience.
In this article, we’ve explored strategies for handling errors gracefully in React applications, including the use of error boundaries to catch errors within component trees and error logging techniques for debugging and monitoring. By incorporating these practices into your development workflow, you can improve the resilience and stability of your React applications.