A recursive function is one which defines a problem in terms of itself.
A recursive function calls itself directly or indirectly until it is stopped. If it is not stopped, then it will call itself forever.
Recursive functions let you perform a unit of work multiple times.
And while it is similar to the workings of a for / while loop, using recursive solutions affords a unique, faster and much more elegant approach to solving a problem.
Let’s write a countdown function, shall we:
- Using Loop.
countDown = (num) => {
for(let i = num; i>0; i--){
console.log(i);
}
}
countDown(6) //5,4,3,2,1
2. Using Recursion
countDown = (num) => {
if(num === 0)
return 0;
console.log(num);
countDown(num-1);
}
countDown(6) //5,4,3,2,1
Base Case
A recursive function usually contains what is referred to as a base case.
A base case is a condition that checks and stops the recursion.
In our above example, the condition:
if(num === 0)
return 0;
is the base case, a condition that checks our program.
Task: sum all numbers until the given one.
Solution:
Using recursion …
function sumAll(val){
if(n === 0){
return 0;
}else{
return val +=sumAll(val-1)
}
}
alert(sumAll(5)) //15
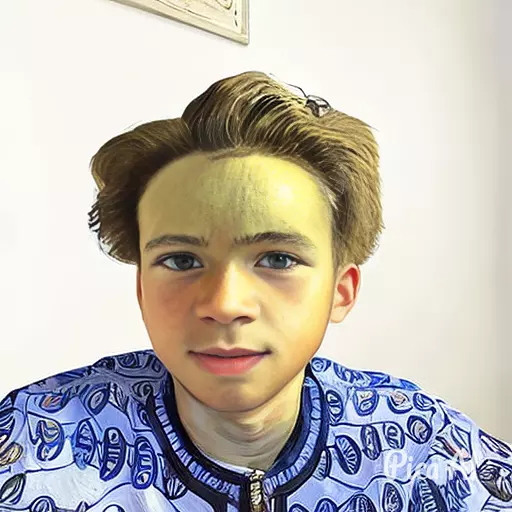