Var Declaration
Before the introduction of ES6 in 2015, var was the go-to way to declare variables in Javascript.
Example:
var a;
a = 5;
console.log(a) //5
But because variable declarations are processed before any code is executed, declaring a variable anywhere in the code is equivalent to declaring it at the top.
This also means that a variable can appear to be used before it is declared.
This behaviour is called “hoisting.”
Example
console.log(bar); //undefined
var bar = 22;
console.log(bar) //22
bar = 33;
console.log(bar) //33
Let Declaration
“let” is one of the ES6 additions to Javascript.
When you use this type of declaration, you are saying you want the variable to be reassigned but not to be redeclared.
Example:
let a = 3;
console.log(a) //3
let a = 5;
console.log(a) //Identifier 'a' has already been declared
//But if we reassign like so
a = 6;
console.log(a) //6
Const Declaration
‘const’ is also an ES6 addition.
When you use const to declare a variable, you are saying that you don’t want that variable to be reassigned or be redeclared.
Example:
const a = 33;
console.log(a) //33
const a = 34;
console.log(a) //Identifier 'a' has already been declared
//Even if we reassign
a = 35;
console.log(a) //Assignment to constant variable
In Summary …
- A var type variable declaration can be both be redeclared and reassigned.
- A let type declaration can only be reassigned but not redeclared.
- A const type declaration can neither be redeclared or reassigned.
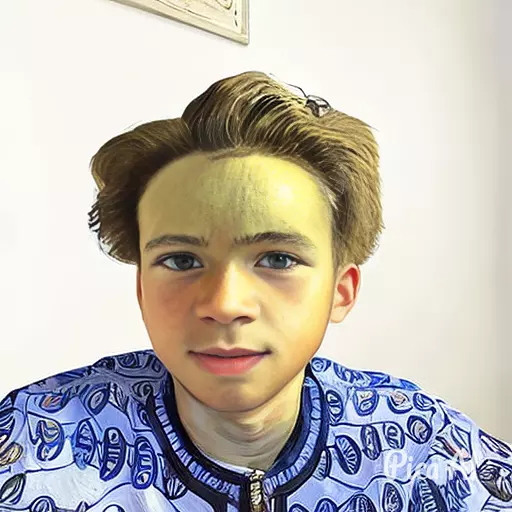