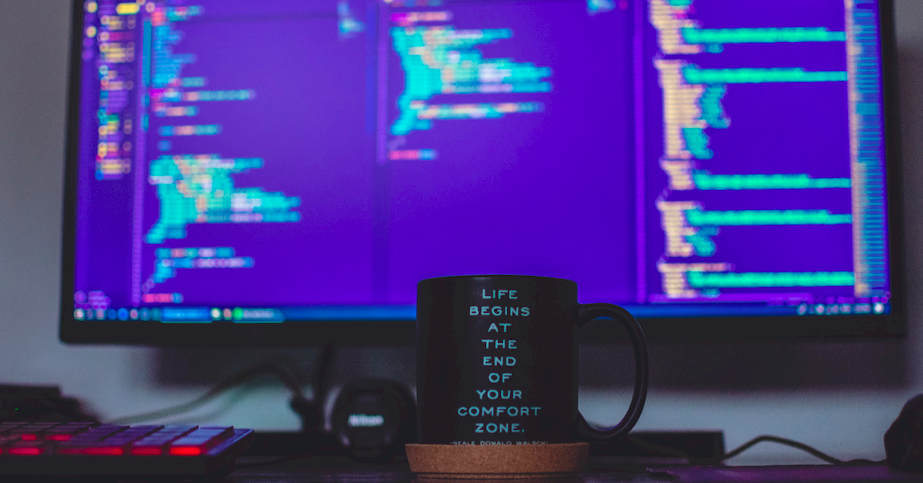
Question: write a JavaScript Program That Prints Out Odd Numbers From 1 – 10
Odd numbers are integers that cannot be divided evenly by 2. In other words, if you divide an odd number by 2, you will get a fraction or decimal as a result, rather than a whole number.
For example, 3, 5, 7, 9, 11, and so on, are all odd numbers because they cannot be divided evenly by 2. In contrast, even numbers can be divided evenly by 2 and have a remainder of 0.
For example, 2, 4, 6, 8, 10, and so on, are all even numbers because they can be divided evenly by 2. Knowing the difference between odd and even numbers is useful in programming and other software development logic implementations, particularly when you need to perform calculations or operations that depend on the parity of numbers.
With that said let’s write a JavaScript program that prints out numbers from 1 – 10
for(let i = 1; i<=10; i++){
if(i%2 !== 0){
console.warn(i);
}
}
This program uses a for
loop to iterate through the numbers 1 to 10. The if
statement inside the loop checks if the current number is odd (i.e., not divisible by 2), and if it is, it prints it to the console using console.log()
. You can adjust the loop range as needed to print out odd numbers within a different range.
You can also modify this program to take user input for the range of numbers to check, like this:
let start = prompt("Enter starting number:");
let end = prompt("Enter ending number:");
for (let i = start; i <= end; i++) {
if (i % 2 !== 0) {
console.log(i);
}
}
This program prompts the user to enter a starting and ending number for the range of numbers to check. The for
loop iterates through this range, and the if
statement inside checks for odd numbers and prints them to the console.
Note that the prompt()
function returns a string, so we need to convert the input to a number using parseInt()
before using it in the loop.