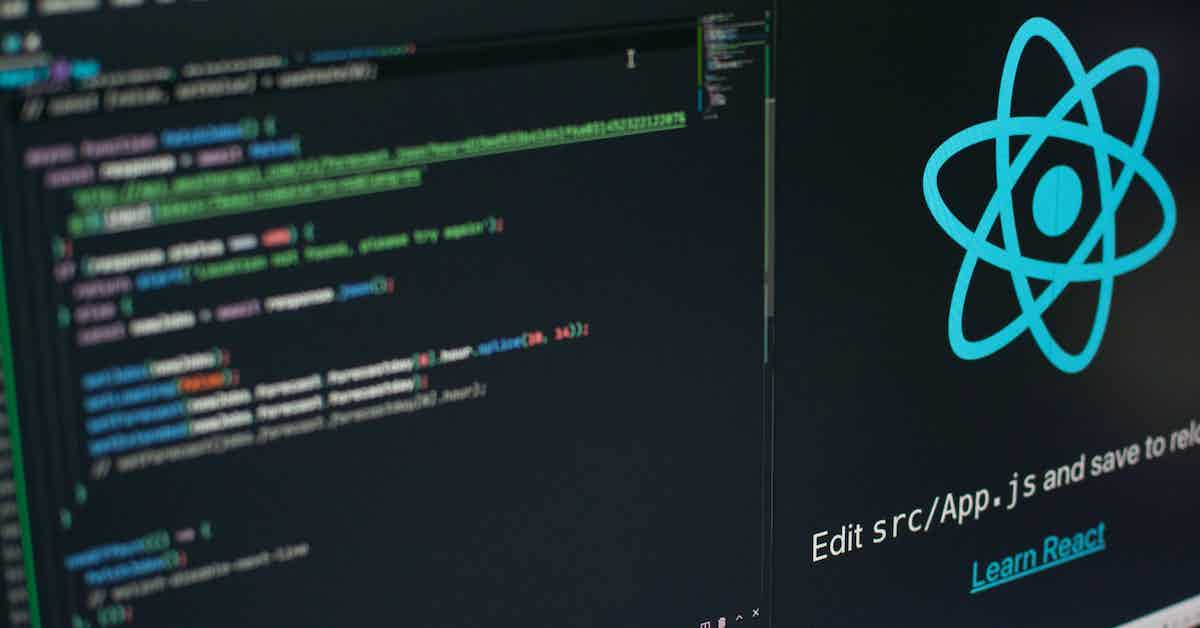
Common React mistakes can slow you down and cause much frustration.
As a software developer, working with the React Library can be fun and exciting. But more often than not, there are common pitfalls that even the most experienced developer can fall into.
Let’s take a look at some of these common React mistakes and how we can solve them.
React Mistakes to Look out for
- Using `class` instead of `
className
`. This is one of the most common mistakes especially if you are coming from traditional web development. React favours the `className` instead of just `class`. And although the code will still run, but you will get loads of warning in your developer console.
<div className="image-box"></div>
2. Forgetting to put the `/` in a self-closing tag. Except you are writing XHTML, self-closing tags can be safely used without the forward slash. But React will not TOLERATE it. All self-closing tags must have a `/` without which the program will throw off error
<div className="image-box">
<img src="" />
</div>
3. Not using keys correctly: When rendering a list of elements in React, it’s important to assign a unique key to each element so that React can efficiently update the DOM. Omitting keys or using non-unique keys can cause issues with rendering and performance.
function NumberList(props) {
const numbers = props.numbers;
const listItems = numbers.map((number) =>
<li key={number.toString()}>
{number}
</li>
);
return (
<ul>{listItems}</ul>
);
}
4. Not handling asynchronous events correctly: React relies heavily on asynchronous events such as API calls and user input. Failing to handle these events properly can cause your application to freeze or crash.
componentDidMount(){
//API calls here
}
5. Improper Error Handling / Not using `try - catch
` block. Improper error handling can also be an oversight and can be one of the most overlooked process. It is important to properly handle errors in your code by setting error boundaries, and `try-catch` is one of the effective ways to do that.
try{
//make calls here
}catch(err){
console.warn(err);
}
6. Using setState() in the render method: This is a big NO. Calling setState() in the render method can cause an infinite loop and slow down your application. Instead, use `setState()`
in lifecycle methods or event handlers.
7. Ignoring React’s lifecycle methods: React has a set of lifecycle methods that allow you to perform actions at specific points in the component’s life cycle. Ignoring these methods can result in unexpected behavior.
8. Overusing props: Passing too many props between components can make your code harder to maintain and can cause performance issues. It’s important to keep your component hierarchy simple and pass only the necessary props.
9. Not using React.memo or useMemo: React.memo and useMemo can help to optimize your application by preventing unnecessary re-renders. Failing to use these methods can cause performance issues as your application grows.