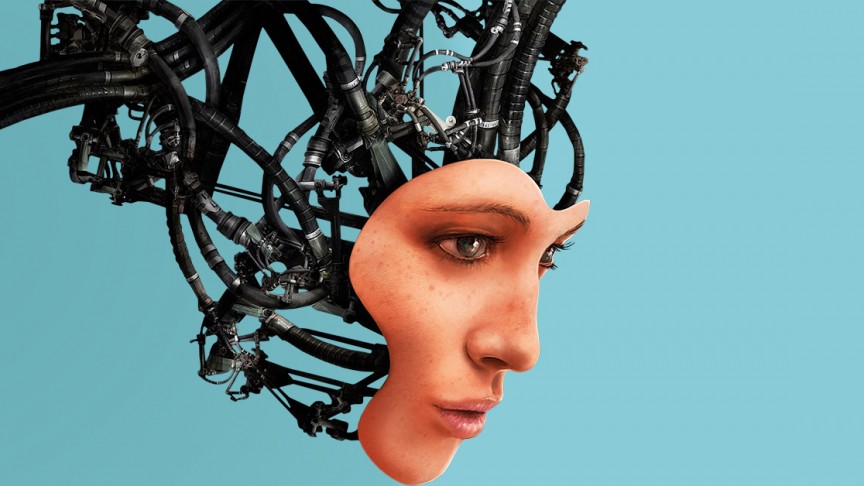
A switch statement gives an expression a value to evaluate and several different statements to execute based on the value of the expression.
It is a useful alternative to the If … else statement and a much more sophisticated way to control program flow in software development.
//Syntax
switch(expression) {
case condition1: statements
break;
case condition2: statements
break;
case condition3: statements
break;
default: statements
}
default: this indicates the statement that runs if all the given conditions are not met. It is similar to the final else block an if statement.
break: the break statements indicate the end of a particular case.
Example
Let us write a simple program that checks the grade of students
checkGrade = (val) => {
switch(val){
case 'A':
console.log('Excellent');
break;
case 'B':
console.log('Very Good');
break;
case 'C':
console.log('Good');
break;
case 'D':
console.log('Fairly Good');
break;
case 'E':
console.log('Fair');
break;
case 'F':
console.log('Fail');
break;
default: console.log('Please enter a valid grade')
}
}
checkGrade('R') //Please enter a valid grade
checkGrade('A') //Excellent