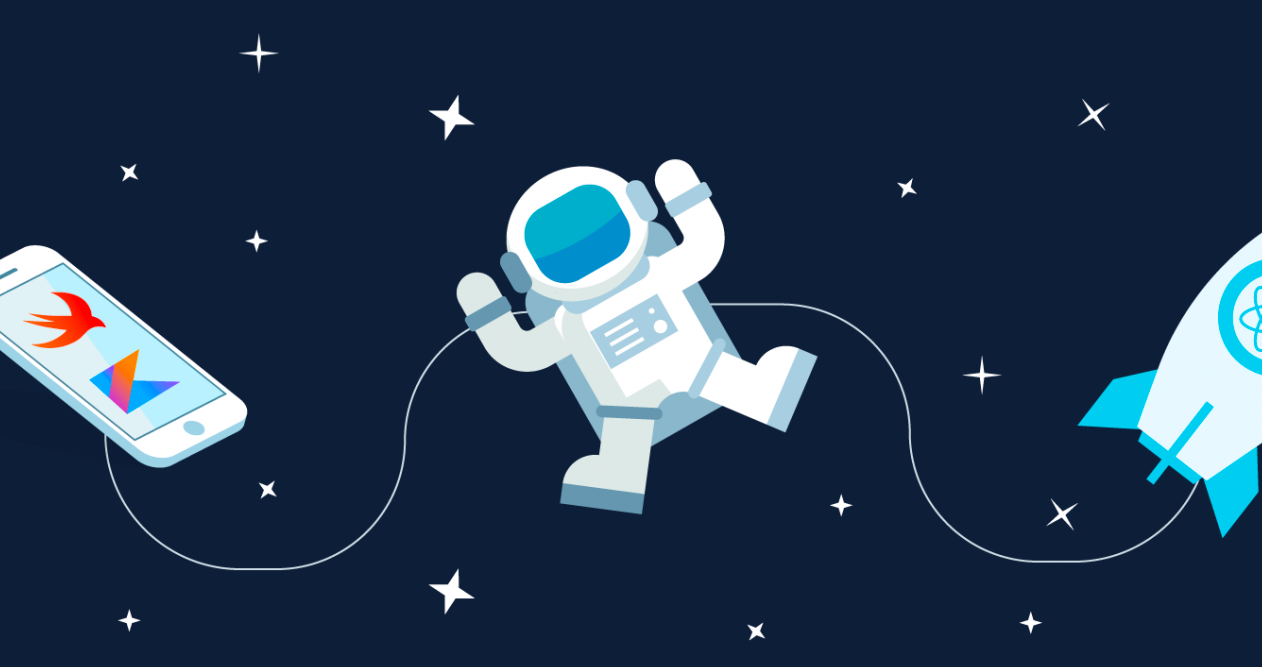
According to the React Native docs, AsyncStorage is an unencrypted, asynchronous, persistent key-value storage system that is global to the app.
This means that data stored in AsyncStorage can be accessed from anywhere, whether class, or function, in the app.
This storage system is also device-specific. What that means is that it does not support data sharing between apps or devices.
Why Use AsyncStorage?
AsyncStorage can be very useful when you want to store small amount of data, especially in text format. Let’s say for instance, you might want to store user login information or perform some form of authentication or the other. Then you can make use of AsyncStorage.
How Much Data Can You Store in AsyncStorage?
Although the iOS AsyncStorage implementation has an unlimited amount of space by default, we cannot say the same for Android. The default memory allocation for Android devices is 6MB. That’s right.
This means that your data should not exceed 6MB or you might encounter some performance issues.
There is, however, a way to increase the storage capacity for Android devices. The steps are as follows:
- First, import AsyncStorage to your application
yarn add @react-native-async-storage/async-storage
2. Next, add this import at the top of your MainActivity.java file:
import com.facebook.react.modules.storage.ReactDatabaseSupplier;
3 Next locate this part of your file that looks like so:
@Override
public void onCreate() {
super.onCreate();
SoLoader.init(this, /* native exopackage */ false);
}
4. Define the number of bytes you want to increase the AsyncStorage to like so:
long size = 70L * 1024L * 1024L; // 70 MB
5. Then set size like so:
com.facebook.react.modules.storage.ReactDatabaseSupplier.getInstance(getApplicationContext()).setMaximumSize(size);
6. Finally your code should look like this:
@Override
public void onCreate() {
super.onCreate();
SoLoader.init(this, /* native exopackage */ false);
long size = 70L * 1024L * 1024L; // 70 MB
com.facebook.react.modules.storage.ReactDatabaseSupplier.getInstance(getApplicationContext()).setMaximumSize(size);
}
This is how you can increase the default AsyncStorage capacity for Android devices. And while this will work, it is not necessarily recommended. If you are going to store large amounts of data in app, consider using a database like SQLite or other preferred database storage systems for optimal performance.
In our next series we will look at how to store items in AsyncStorage