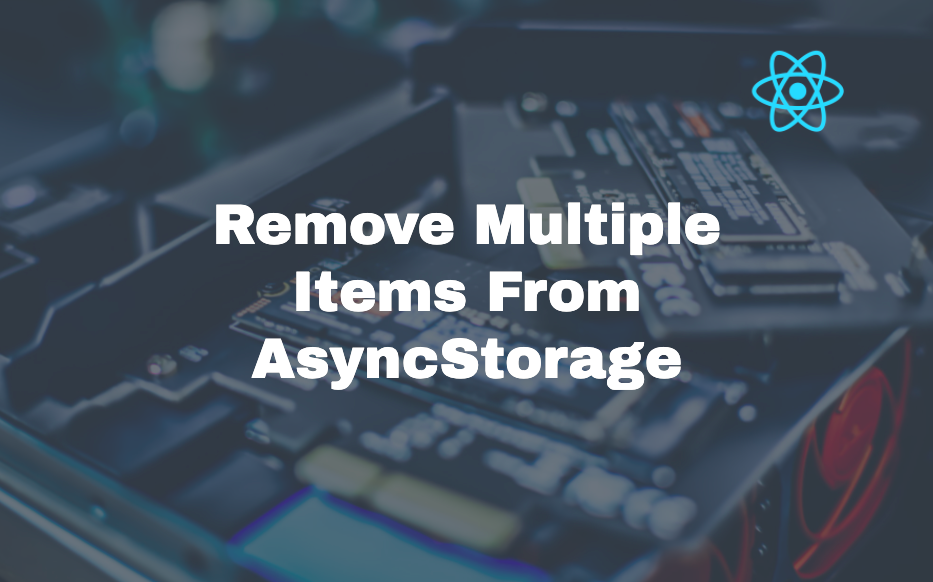
AsyncStorage is used in React Native mobile app development to store items in an unencrypted, asynchronous, persistent, key-value storage way. These stored items are global to the app.
It is true that we can remove single items from the storage, but it is also true we can remove multiple items from the storage at the same time.
See how to add items to AsyncStorage
1. Define an array of keys
The first thing we’ll do is to define our array of keys we want to remove. Let’s assume you’ve already added a token, phone number and email that you are retrieving from an API, we would have to do the following
let keys = ['token', 'phone', 'email'];
2. Remove the items
Next, we will import AsyncStorage and proceed to remove these items.
import React, {Component} from 'react';
import {View, Alert} from 'react-native';
import AsyncStorage from '@react-native-async-storage/async-storage';
AsyncStorage.multiRemove(keys).then((res) => {
Alert.alert("Items removed from storage");
});
There we have it. This is how we can remove multiple items from AsyncStorage.
See also:
Remove an item from AsyncStorage