Using PHP, we can shorten the length of our string and just replace the rest with three dots.
This is usually needed in a software application, especially when the string length is too long to show to the user.
So we will write a function that is re-usable and which we can always call or use in any other programming.
function trimString($string, $repl, $limit)
{
if(strlen($string) > $limit)
{
return substr($string, 12, $limit) . $repl;
}
else
{
return $string;
}
}
?>
echo trimString("Hello Codeflare", "...", 3);
Explanation
The trimString function we created takes three (3) parameters:
- The string which we want to shorten,
- What we want to replace the cut out section with, which in this case is three (3) dots
- And the limit or length of string.
Next, we check if the length of the given string is greater than the limit which we have set. If it is, we truncate it and append our three dots. If it’s not, we just return the given string.
Conclusion
This is how we shorten the length of a string and replace it with three (3) dots in PHP.
Let me know what you think in the comments.
See Also:
Image Upload With Preview Using PHP’s PDO And jQuery
Enrol for Software Development Training
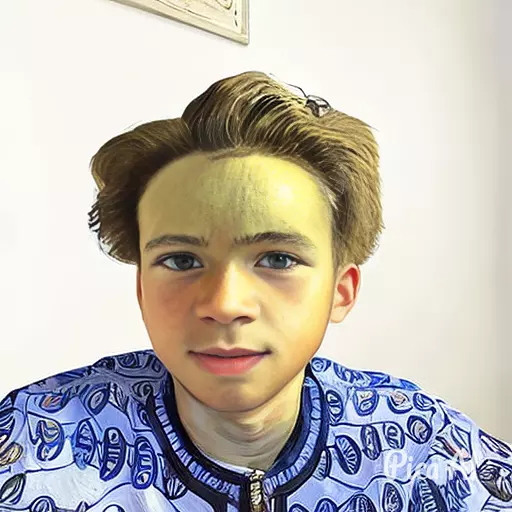