In the realm of frontend web development, managing the state of your application is a critical task. React, a popular JavaScript library, offers an elegant solution to this challenge through its concept of “state.” State variables enable components to store and manage data that can change over time, ensuring that your application remains responsive and interactive. In this article, we’ll delve into the world of React state variables, covering their fundamentals, use cases, best practices, and advanced techniques.
Understanding React State Variables
At its core, React is a library designed to build user interfaces by composing reusable components. In many applications, the data displayed on a user interface can change based on user interactions, data fetching, or other events. React components need a way to hold and manage this changing data, and this is where state variables come into play.
State refers to the data that a component manages and can change over time. React components can have state by using the useState
hook (introduced in React 16.8) or by extending the React.Component
class. State variables allow components to be dynamic, reactive, and responsive to user actions.
Using useState
Hook for State Variables
Introduced as part of the React Hooks API, the useState
hook is the recommended way to manage state in functional components. Let’s dive into its usage.
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
In this example, we initialize a state variable called count
with an initial value of 0
. The setCount
function is used to update the count
value. When the button is clicked, the increment
function is called, which increases the count by 1.
Using setState for State Variables
For class components, we can use the setState feature to both store and manipulate values of a state variable.
Here is an example of the increment counter using class components using React Native. You can see full tutorial here
import { StyleSheet } from 'react-native';
class Sample extends PureComponent{
constructor(props){
super(props);
this.state = {
counter: 0
}
}
increaseCount = () => {
this.setState({counter: this.state.counter + 1})
}
decreaseCount = () => {
this.setState({counter: this.state.counter - 1})
if(this.state.counter === 0){
this.setState({counter: 0 })
}
}
render(){
return(
<View style={styles.container}>
<TouchableOpacity style={styles.button} onPress={()=>this.increaseCount()}>
<Text>Increase</Text>
</TouchableOpacity>
<Text>{this.state.counter}</Text>
<TouchableOpacity style={styles.button} onPress={()=>this.decreaseCount()}>
<Text>Decrease</Text>
</TouchableOpacity>
</View>
)
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
flexDirection: 'row',
justifyContent: 'space-evenly',
alignItems: 'center',
margin: 18,
},
button: {
width: 100,
height: 45,
alignItems: 'center',
justifyContent: 'center',
backgroundColor: '#87CEEB'
},
})
export default Sample
Best Practices for Using State Variables
Effective use of state variables is key to building maintainable and performant React applications. Here are some best practices to keep in mind:
1. Limit State to What’s Necessary
Avoid overloading your components with excessive state variables. Think carefully about what data needs to be managed by state and what can be passed as props.
2. Choose the Right Component for State
If a component doesn’t need to manage complex state, consider using a functional component with the useState
hook. For more complex state management scenarios, use a state management library like Redux or Mobx.
3. Keep Components Stateless
Whenever possible, design your components to be stateless (also known as “dumb” components). This separation of concerns improves reusability and makes your application easier to test.
4. Use Immutability
When updating state, always create a new object or array instead of modifying the existing state directly. This practice prevents unintended side effects and helps React’s reconciliation process work efficiently.
5. Minimize Re-Renders
Use React’s shouldComponentUpdate
or React.memo
to optimize your components and prevent unnecessary re-renders when state changes don’t affect the component’s output.
6. Understand the Component Lifecycle
For class components, understanding the component lifecycle is essential for managing state changes, side effects, and avoiding memory leaks. However, with functional components and hooks, the focus shifts to using the useEffect
hook for handling side effects.
Advanced State Management Techniques
As your application grows, you might encounter more complex scenarios that demand advanced state management techniques. While useState
is suitable for many use cases, there are additional options to consider:
Context API
React’s Context API allows you to manage global state that needs to be shared across multiple components. It’s particularly useful for themes, authentication, and user preferences.
Redux
Redux is a popular state management library that provides a centralized store for your application’s state. It’s beneficial for large-scale applications with complex state interactions.
Mobx
Recoil is a state management library developed by Facebook that leverages the idea of atoms (individual pieces of state) and selectors (computed values based on atoms). It aims to provide a more intuitive way of managing state.
Conclusion
React state variables lie at the heart of building dynamic and interactive user interfaces. By understanding the fundamentals of state, exploring its versatile use cases, and implementing best practices, you can create applications that are both responsive and maintainable. Whether you’re building a simple counter or a sophisticated data-driven application, React’s state management capabilities empower you to deliver exceptional user experiences. Stay curious, experiment with different techniques, and continuously refine your understanding of state management as you embark on your journey as a React developer.
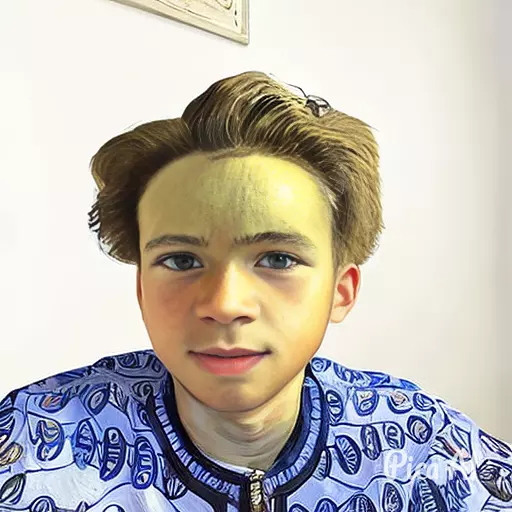